Aitendo OLED device driver library.
Dependents: AitendoOLED_TestProgram
AitendoOLED.h
00001 /** 00002 * ============================================================================= 00003 * Aitendo OLED device driver library. (Version 0.0.2) 00004 * 00005 * [The product] 00006 * OLED-2P-095BWNN-SPI : http://www.aitendo.co.jp/product/2099 00007 * ALO-095BWNN-J9 : http://www.aitendo.co.jp/product/1449 00008 * 00009 * [References] 00010 * http://serdisplib.sourceforge.net/ser/doc/Treiber_IC-SSD1332_OLED_96x64_COLOR.pdf 00011 * ============================================================================= 00012 * Copyright (c) 2010 Shinichiro Nakamura (CuBeatSystems) 00013 * 00014 * Permission is hereby granted, free of charge, to any person obtaining a copy 00015 * of this software and associated documentation files (the "Software"), to deal 00016 * in the Software without restriction, including without limitation the rights 00017 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00018 * copies of the Software, and to permit persons to whom the Software is 00019 * furnished to do so, subject to the following conditions: 00020 * 00021 * The above copyright notice and this permission notice shall be included in 00022 * all copies or substantial portions of the Software. 00023 * 00024 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00025 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00026 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00027 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00028 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00029 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00030 * THE SOFTWARE. 00031 * ============================================================================= 00032 */ 00033 #ifndef AITENDO_OLED_H 00034 #define AITENDO_OLED_H 00035 00036 #include "mbed.h" 00037 00038 /** 00039 * OLED. 00040 */ 00041 class AitendoOLED { 00042 public: 00043 /** 00044 * Create. 00045 * 00046 * @param pin_mosi MOSI. 00047 * @param pin miso MISO. 00048 * @param pin_sclk SCLK. 00049 * @param pin_res RES. 00050 * @param pin_cs CS. 00051 * @param pin_dc DC. 00052 */ 00053 AitendoOLED( 00054 PinName pin_mosi, 00055 PinName pin_miso, 00056 PinName pin_sclk, 00057 PinName pin_res, 00058 PinName pin_cs, 00059 PinName pin_dc); 00060 00061 /** 00062 * Dispose. 00063 */ 00064 ~AitendoOLED(); 00065 00066 typedef struct color { 00067 uint8_t r; 00068 uint8_t g; 00069 uint8_t b; 00070 } Color; 00071 00072 /** 00073 * Draw pixel. 00074 * 00075 * @param x X. 00076 * @param y Y. 00077 * @param c Color. 00078 */ 00079 void drawPixel(int x, int y, Color c); 00080 00081 /** 00082 * Draw line. 00083 * 00084 * @param x1 X1. 00085 * @param y1 Y1. 00086 * @param x2 X2. 00087 * @param y2 Y2. 00088 * @param c Color. 00089 */ 00090 void drawLine(int x1, int y1, int x2, int y2, Color c); 00091 00092 /** 00093 * Fill box. 00094 * 00095 * @param x1 X1. 00096 * @param y1 Y1. 00097 * @param x2 X2. 00098 * @param y2 Y2. 00099 * @param c1 Color1. 00100 * @param c2 Color2. 00101 */ 00102 void fillBox(int x1, int y1, int x2, int y2, Color c1, Color c2); 00103 00104 /** 00105 * Copy. 00106 * 00107 * @param x1 X1. 00108 * @param y1 Y1. 00109 * @param x2 X2. 00110 * @param y2 Y2. 00111 * @param nx X of the destination. 00112 * @param ny Y of the destination. 00113 */ 00114 void copy(int x1, int y1, int x2, int y2, int nx, int ny); 00115 00116 /** 00117 * Darker. 00118 * 00119 * @param x1 X1. 00120 * @param y1 Y1. 00121 * @param x2 X2. 00122 * @param y2 Y2. 00123 */ 00124 void darker(int x1, int y1, int x2, int y2); 00125 00126 /** 00127 * Clear. 00128 * 00129 * @param x1 X1. 00130 * @param y1 Y1. 00131 * @param x2 X2. 00132 * @param y2 Y2. 00133 */ 00134 void clear(int x1, int y1, int x2, int y2); 00135 private: 00136 AitendoOLED(); 00137 00138 SPI io_spi; 00139 DigitalOut io_res; 00140 DigitalOut io_cs; 00141 DigitalOut io_dc; 00142 00143 /** 00144 * Reset. 00145 */ 00146 void reset(); 00147 00148 /** 00149 * Write data. 00150 * 00151 * @param data Data. 00152 */ 00153 void writeData(uint8_t data); 00154 00155 /** 00156 * Set mode. 00157 * 00158 * @param fillrect Fill the rectangle. 00159 * @param revcopy Reverse copy. 00160 */ 00161 void setMode(bool fillrect, bool revcopy); 00162 00163 /** 00164 * Set column address. 00165 * 00166 * @param start Start address. (0-95) 00167 * @param end End address. (0-95) 00168 */ 00169 void setColumnAddress(int start, int end); 00170 00171 /** 00172 * Set row address. 00173 * 00174 * @param start Start address. (0-63) 00175 * @param end End address. (0-63) 00176 */ 00177 void setRowAddress(int start, int end); 00178 00179 /** 00180 * Set contrast for Color A. 00181 * 00182 * @param contrast Contrast. (Default:0x80) 00183 */ 00184 void setContrastForColorA(int contrast); 00185 00186 /** 00187 * Set contrast for Color B. 00188 * 00189 * @param contrast Contrast. (Default:0x80) 00190 */ 00191 void setContrastForColorB(int contrast); 00192 00193 /** 00194 * Set contrast for Color C. 00195 * 00196 * @param contrast Contrast. (Default:0x80) 00197 */ 00198 void setContrastForColorC(int contrast); 00199 00200 /** 00201 * Set master current control value. 00202 * 00203 * @param current Current value. (0x00-0x0F) 00204 */ 00205 void setMasterCurrentControl(int current); 00206 00207 /** 00208 * Set remapping mode and the data format. 00209 * 00210 * @param mode See the document. 00211 */ 00212 void setRemapAndDataFormat(int mode); 00213 00214 /** 00215 * Set display start line. 00216 * 00217 * @param line Start line number. (0-63) 00218 */ 00219 void setDisplayStartLine(int line); 00220 00221 /** 00222 * Set display offset line. 00223 * 00224 * @param offset Offset line number. (0-63) 00225 */ 00226 void setDisplayOffset(int offset); 00227 00228 typedef enum { 00229 NormalDisplay, 00230 EntireDisplayOn, 00231 EntireDisplayOff, 00232 InverseDisplay 00233 } DisplayMode; 00234 00235 /** 00236 * Set display mode. 00237 * 00238 * @param mode Display mode. 00239 */ 00240 void setDisplayMode(DisplayMode mode); 00241 00242 /** 00243 * Set multiplex ratio. 00244 * 00245 * @param ratio Ratio. 00246 */ 00247 void setMultiplexRatio(int ratio); 00248 00249 /** 00250 * Set display on/off. 00251 * 00252 * @param on On. 00253 */ 00254 void setDisplayOnOff(bool on); 00255 00256 typedef struct gray_scale_table { 00257 char data[32]; 00258 } gray_scale_table_t; 00259 00260 /** 00261 * Set gray scale table. 00262 * 00263 * @param p A pointer to the look up table. 00264 */ 00265 void setGrayScaleTable(gray_scale_table_t *p); 00266 00267 /** 00268 * NOP. 00269 */ 00270 void nop(); 00271 00272 /** 00273 * Set power saving mode. 00274 * 00275 * @param value Value. (0x00:None, 0x12:power saving) 00276 */ 00277 void setPowerSavingMode(int value); 00278 00279 /** 00280 * Set phase period. 00281 * 00282 * @param value Value. (Default:0x74) 00283 */ 00284 void setPhasePeriod(int value); 00285 00286 /** 00287 * Set display clock divide ratio. 00288 * 00289 * @param value Value. (Default:0x00) 00290 */ 00291 void setDisplayClockDivideRatio(int value); 00292 00293 /** 00294 * Set second pre-charge speed for color A. 00295 * 00296 * @param value Value. 00297 */ 00298 void setSecondPreChargeSpeedForColorA(int value); 00299 00300 /** 00301 * Set second pre-charge speed for color B. 00302 * 00303 * @param value Value. 00304 */ 00305 void setSecondPreChargeSpeedForColorB(int value); 00306 00307 /** 00308 * Set second pre-charge speed for color C. 00309 * 00310 * @param value Value. 00311 */ 00312 void setSecondPreChargeSpeedForColorC(int value); 00313 00314 /** 00315 * Set pre charge level for color A. 00316 * 00317 * @param value The value. 00318 */ 00319 void setPreChargeLevelForColorA(int value); 00320 00321 /** 00322 * Set pre charge level for color B. 00323 * 00324 * @param value The value. 00325 */ 00326 void setPreChargeLevelForColorB(int value); 00327 00328 /** 00329 * Set pre charge level for color C. 00330 * 00331 * @param value The value. 00332 */ 00333 void setPreChargeLevelForColorC(int value); 00334 00335 /** 00336 * Set VCOMH. 00337 * 00338 * @param value VCOMH value. (0x00:0.43 * Vref, 0x3F:0x83 * Vref) 00339 */ 00340 void setVCOMH(int value); 00341 00342 /** 00343 * Read the status register. 00344 * 00345 * @return the value. 00346 */ 00347 uint8_t readStatusRegister(); 00348 }; 00349 00350 #endif
Generated on Mon Jul 18 2022 10:37:03 by
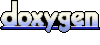