
For test
Embed:
(wiki syntax)
Show/hide line numbers
ESP8266.cpp
00001 #include "ESP8266.h " 00002 00003 00004 ESP8266::ESP8266(PinName tx, PinName rx, int baud_rate):m_uart(tx, rx) { 00005 m_uart.begin(baud_rate); 00006 m_uart.flush(); 00007 m_uart.setTimeout(1000); 00008 } 00009 00010 int ESP8266::getMuxID(void) { 00011 return m_mux_id; 00012 } 00013 00014 bool ESP8266::reset(void) 00015 { 00016 unsigned long start; 00017 m_uart.setTimeout(100); 00018 m_uart.flush(); 00019 m_uart.println("AT+RST"); 00020 if (!m_uart.find("OK")) { 00021 return false; 00022 } 00023 00024 delay(2000); 00025 00026 start = millis(); 00027 while (millis() - start < 3000) { 00028 m_uart.flush(); 00029 m_uart.println("AT"); 00030 if (m_uart.find("OK")) { 00031 delay(500); 00032 return true; 00033 } 00034 delay(100); 00035 } 00036 return false; 00037 } 00038 00039 bool ESP8266::setStationMode(String ssid, String pwd) 00040 { 00041 if (!confMode(ESP8266_MODE_STA)) 00042 { 00043 return false; 00044 } 00045 return confJAP(ssid, pwd); 00046 } 00047 00048 bool ESP8266::setSoftAPMode(String sap_ssid, String sap_pwd, int chl, ESP8266Encrypstion ecn) 00049 { 00050 if (!confMode(ESP8266_MODE_SAP)) 00051 { 00052 return false; 00053 } 00054 return confSAP(sap_ssid, sap_pwd, chl, ecn); 00055 } 00056 00057 bool ESP8266::setStationSoftAPMode(String ssid, String pwd, String sap_ssid, String sap_pwd , 00058 int chl, ESP8266Encrypstion ecn) 00059 { 00060 if (!confMode(ESP8266_MODE_STA_SAP)) 00061 { 00062 return false; 00063 } 00064 if (confJAP(ssid, pwd) && confSAP(sap_ssid, sap_pwd, chl, ecn)) { 00065 return true; 00066 } else { 00067 return false; 00068 } 00069 } 00070 00071 bool ESP8266::ipConfig(ESP8266CommType type, String addr, int port, ESP8266Mux mux, int id) 00072 { 00073 bool result = false; 00074 if (mux == ESP8266_MUX_SINGLE) 00075 { 00076 confMux(mux); 00077 delay(5000); 00078 result = newMux(type, addr, port); 00079 } 00080 else if (mux == ESP8266_MUX_MULTIPLE) 00081 { 00082 confMux(mux); 00083 delay(5000); 00084 result = newMux(id, type, addr, port); 00085 } 00086 return result; 00087 } 00088 00089 int ESP8266::recvData(char *buf) 00090 { 00091 String data = ""; 00092 if (m_uart.available()>0) 00093 { 00094 00095 unsigned long start; 00096 start = millis(); 00097 char c0 = m_uart.readChr(); 00098 if (c0 == '+') 00099 { 00100 00101 while (millis()-start<5000) 00102 { 00103 if (m_uart.available()>0) 00104 { 00105 char c = m_uart.readChr(); 00106 data += c; 00107 } 00108 if (data.indexOf("\nOK")!=-1) 00109 { 00110 break; 00111 } 00112 } 00113 00114 int sLen = strlen(data.c_str()); 00115 int i,j; 00116 for (i = 0; i <= sLen; i++) 00117 { 00118 if (data[i] == ':') 00119 { 00120 break; 00121 } 00122 00123 } 00124 bool found = false; 00125 for (j = 4; j <= i; j++) 00126 { 00127 if (data[j] == ',') 00128 { 00129 found = true; 00130 break; 00131 } 00132 00133 } 00134 int iSize; 00135 if(found ==true) 00136 { 00137 String _id = data.substring(4, j); 00138 m_mux_id = _id.toInt(); 00139 String _size = data.substring(j+1, i); 00140 iSize = _size.toInt(); 00141 00142 String str = data.substring(i+1, i+1+iSize); 00143 strcpy(buf, str.c_str()); 00144 00145 00146 } 00147 else 00148 { 00149 String _size = data.substring(4, i); 00150 iSize = _size.toInt(); 00151 00152 00153 String str = data.substring(i+1, i+1+iSize); 00154 strcpy(buf, str.c_str()); 00155 00156 } 00157 return iSize; 00158 } 00159 } 00160 00161 return 0; 00162 } 00163 00164 String ESP8266::showMode() 00165 { 00166 String data; 00167 m_uart.println("AT+CWMODE?"); 00168 unsigned long start; 00169 start = millis(); 00170 while (millis()-start<2000) { 00171 if(m_uart.available()>0) 00172 { 00173 char a =m_uart.readChr(); 00174 data=data+a; 00175 } 00176 if (data.indexOf("OK")!=-1) 00177 { 00178 break; 00179 } 00180 } 00181 if(data.indexOf("1")!=-1) 00182 { 00183 return "Station"; 00184 }else if(data.indexOf("2")!=-1) 00185 { 00186 return "AP"; 00187 }else if(data.indexOf("3")!=-1) 00188 { 00189 return "AP+Station"; 00190 }else{ 00191 return "Invalid Mode!"; 00192 } 00193 } 00194 00195 bool ESP8266::confMode(ESP8266WorkMode mode) 00196 { 00197 String data; 00198 m_uart.flush(); 00199 m_uart.print("AT+CWMODE="); 00200 m_uart.println(String(mode)); 00201 unsigned long start; 00202 start = millis(); 00203 while (millis()-start<2000) { 00204 while(m_uart.available()>0) { 00205 char c = m_uart.readChr(); 00206 data += c; 00207 } 00208 if (data.indexOf("OK")!=-1 || data.indexOf("no change")!=-1) 00209 { 00210 return true; 00211 } else if (data.indexOf("ERROR")!=-1 || data.indexOf("busy")!=-1) 00212 { 00213 return false; 00214 } 00215 } 00216 return false; 00217 } 00218 00219 String ESP8266::showAP(void) 00220 { 00221 String data; 00222 m_uart.flush(); 00223 m_uart.print("AT+CWLAP\r\n"); 00224 delay(5000); 00225 00226 unsigned long start; 00227 start = millis(); 00228 while (millis()-start<8000) { 00229 if(m_uart.available()>0) 00230 { 00231 char a =m_uart.readChr(); 00232 data=data+a; 00233 } 00234 if (data.indexOf("OK")!=-1 || data.indexOf("ERROR")!=-1 ) 00235 { 00236 break; 00237 } 00238 } 00239 if(data.indexOf("ERROR")!=-1) 00240 { 00241 return "ERROR"; 00242 } 00243 else{ 00244 char head[4] = {0x0D,0x0A}; 00245 char tail[7] = {0x0D,0x0A,0x0D,0x0A}; 00246 data.replace("AT+CWLAP",""); 00247 data.replace("OK",""); 00248 data.replace("+CWLAP","ESP8266"); 00249 data.replace(tail,""); 00250 data.replace(head,""); 00251 00252 return data; 00253 } 00254 } 00255 00256 String ESP8266::showJAP(void) 00257 { 00258 bool ret = false; 00259 m_uart.flush(); 00260 m_uart.println("AT+CWJAP?"); 00261 00262 String data; 00263 unsigned long start; 00264 start = millis(); 00265 while (millis()-start < 3000) { 00266 while(m_uart.available() > 0) { 00267 char a = m_uart.readChr(); 00268 data += a; 00269 //printf("%c", a); 00270 } 00271 if (data.indexOf("OK") != -1) { 00272 ret = true; 00273 break; 00274 } else if (data.indexOf("ERROR") != -1) { 00275 ret = false; 00276 break; 00277 } 00278 } 00279 if (ret) { 00280 int index1 = data.indexOf(":\""); 00281 int index2 = data.indexOf("\"\r\n"); 00282 if (index1 != -1 && index2 != -1) { 00283 return data.substring(index1 + 2, index2); 00284 } else { 00285 return "not found"; 00286 } 00287 } else { 00288 return "null"; 00289 } 00290 } 00291 00292 bool ESP8266::confJAP(String ssid , String pwd) 00293 { 00294 bool ret = false; 00295 m_uart.flush(); 00296 m_uart.print("AT+CWJAP="); 00297 m_uart.print("\""); //"ssid" 00298 m_uart.print(ssid); 00299 m_uart.print("\""); 00300 00301 m_uart.print(","); 00302 00303 m_uart.print("\""); //"pwd" 00304 m_uart.print(pwd); 00305 m_uart.println("\""); 00306 00307 String data; 00308 unsigned long start; 00309 start = millis(); 00310 while (millis() - start < 10000) { 00311 while (m_uart.available() > 0) { 00312 char c = m_uart.readChr(); 00313 data += c; 00314 } 00315 if(data.indexOf("OK") != -1) { 00316 ret = true; 00317 break; 00318 } else if (data.indexOf("ERROR") != -1) { 00319 ret = false; 00320 break; 00321 } 00322 } 00323 00324 if (ret) { 00325 return true; 00326 } else { 00327 return false; 00328 } 00329 } 00330 00331 bool ESP8266::quitAP(void) 00332 { 00333 m_uart.println("AT+CWQAP"); 00334 unsigned long start; 00335 start = millis(); 00336 while (millis()-start<3000) { 00337 if(m_uart.find("OK")==true) 00338 { 00339 return true; 00340 00341 } 00342 } 00343 return false; 00344 00345 } 00346 00347 String ESP8266::showSAP() 00348 { 00349 m_uart.println("AT+CWSAP?"); 00350 String data; 00351 unsigned long start; 00352 start = millis(); 00353 while (millis()-start<3000) { 00354 if(m_uart.available()>0) 00355 { 00356 char a =m_uart.readChr(); 00357 data=data+a; 00358 } 00359 if (data.indexOf("OK")!=-1 || data.indexOf("ERROR")!=-1 ) 00360 { 00361 break; 00362 } 00363 } 00364 char head[4] = {0x0D,0x0A}; 00365 char tail[7] = {0x0D,0x0A,0x0D,0x0A}; 00366 data.replace("AT+CWSAP?",""); 00367 data.replace("+CWSAP","mySAP"); 00368 data.replace("OK",""); 00369 data.replace(tail,""); 00370 data.replace(head,""); 00371 00372 return data; 00373 } 00374 00375 bool ESP8266::confSAP(String ssid , String pwd , int chl , int ecn) 00376 { 00377 m_uart.print("AT+CWSAP="); 00378 m_uart.print("\""); //"ssid" 00379 m_uart.print(ssid); 00380 m_uart.print("\""); 00381 00382 m_uart.print(","); 00383 00384 m_uart.print("\""); //"pwd" 00385 m_uart.print(pwd); 00386 m_uart.print("\""); 00387 00388 m_uart.print(","); 00389 m_uart.print(String(chl)); 00390 00391 m_uart.print(","); 00392 m_uart.println(String(ecn)); 00393 unsigned long start; 00394 start = millis(); 00395 while (millis()-start<3000) { 00396 if(m_uart.find("OK")==true ) 00397 { 00398 return true; 00399 } 00400 } 00401 00402 return false; 00403 00404 } 00405 00406 00407 String ESP8266::showStatus(void) 00408 { 00409 m_uart.println("AT+CIPSTATUS"); 00410 String data; 00411 unsigned long start; 00412 start = millis(); 00413 while (millis()-start<3000) { 00414 if(m_uart.available()>0) 00415 { 00416 char a =m_uart.readChr(); 00417 data=data+a; 00418 } 00419 if (data.indexOf("OK")!=-1) 00420 { 00421 break; 00422 } 00423 } 00424 00425 char head[4] = {0x0D,0x0A}; 00426 char tail[7] = {0x0D,0x0A,0x0D,0x0A}; 00427 data.replace("AT+CIPSTATUS",""); 00428 data.replace("OK",""); 00429 data.replace(tail,""); 00430 data.replace(head,""); 00431 00432 return data; 00433 } 00434 00435 String ESP8266::showMux(void) 00436 { 00437 String data; 00438 m_uart.println("AT+CIPMUX?"); 00439 00440 unsigned long start; 00441 start = millis(); 00442 while (millis()-start<3000) { 00443 if(m_uart.available()>0) 00444 { 00445 char a =m_uart.readChr(); 00446 data=data+a; 00447 } 00448 if (data.indexOf("OK")!=-1) 00449 { 00450 break; 00451 } 00452 } 00453 char head[4] = {0x0D,0x0A}; 00454 char tail[7] = {0x0D,0x0A,0x0D,0x0A}; 00455 data.replace("AT+CIPMUX?",""); 00456 data.replace("+CIPMUX","showMux"); 00457 data.replace("OK",""); 00458 data.replace(tail,""); 00459 data.replace(head,""); 00460 00461 return data; 00462 } 00463 00464 bool ESP8266::confMux(ESP8266Mux mux) 00465 { 00466 m_uart.print("AT+CIPMUX="); 00467 m_uart.println(mux); 00468 unsigned long start; 00469 start = millis(); 00470 while (millis()-start<3000) { 00471 if(m_uart.find("OK")==true ) 00472 { 00473 return true; 00474 } 00475 } 00476 00477 return false; 00478 } 00479 00480 bool ESP8266::newMux(ESP8266CommType type, String addr, int port) 00481 00482 { 00483 String data; 00484 m_uart.flush(); 00485 m_uart.print("AT+CIPSTART="); 00486 if(ESP8266_COMM_TCP == type) { 00487 m_uart.print("\"TCP\""); 00488 } else if (ESP8266_COMM_UDP == type) { 00489 m_uart.print("\"UDP\""); 00490 } 00491 m_uart.print(","); 00492 m_uart.print("\""); 00493 m_uart.print(addr); 00494 m_uart.print("\""); 00495 m_uart.print(","); 00496 m_uart.println(String(port)); 00497 unsigned long start; 00498 start = millis(); 00499 while (millis()-start<10000) { 00500 if(m_uart.available()>0) 00501 { 00502 char a =m_uart.readChr(); 00503 data=data+a; 00504 } 00505 if (data.indexOf("OK")!=-1 || data.indexOf("ALREAY CONNECT")!=-1 || data.indexOf("ERROR")!=-1) 00506 { 00507 return true; 00508 } 00509 } 00510 return false; 00511 } 00512 00513 bool ESP8266::newMux( int id, ESP8266CommType type, String addr, int port) 00514 { 00515 m_uart.print("AT+CIPSTART="); 00516 m_uart.print("\""); 00517 m_uart.print(String(id)); 00518 m_uart.print("\""); 00519 if(ESP8266_COMM_TCP == type) { 00520 m_uart.print("\"TCP\""); 00521 } else if (ESP8266_COMM_UDP == type) { 00522 m_uart.print("\"UDP\""); 00523 } 00524 m_uart.print(","); 00525 m_uart.print("\""); 00526 m_uart.print(addr); 00527 m_uart.print("\""); 00528 m_uart.print(","); 00529 m_uart.println(String(port)); 00530 String data; 00531 unsigned long start; 00532 start = millis(); 00533 while (millis()-start<3000) { 00534 if(m_uart.available()>0) 00535 { 00536 char a =m_uart.readChr(); 00537 data=data+a; 00538 } 00539 if (data.indexOf("OK")!=-1 || data.indexOf("ALREAY CONNECT")!=-1 ) 00540 { 00541 return true; 00542 } 00543 } 00544 return false; 00545 00546 00547 } 00548 00549 bool ESP8266::send(String str) 00550 { 00551 m_uart.flush(); 00552 m_uart.print("AT+CIPSEND="); 00553 m_uart.println(str.length()); 00554 unsigned long start; 00555 start = millis(); 00556 bool found = false; 00557 while (millis()-start<5000) { 00558 if(m_uart.find(">")==true ) 00559 { 00560 found = true; 00561 break; 00562 } 00563 } 00564 if(found) 00565 m_uart.print(str); 00566 else 00567 { 00568 closeMux(); 00569 return false; 00570 } 00571 00572 00573 String data; 00574 start = millis(); 00575 while (millis()-start<5000) { 00576 if(m_uart.available()>0) 00577 { 00578 char a =m_uart.readChr(); 00579 data=data+a; 00580 } 00581 if (data.indexOf("SEND OK")!=-1) 00582 { 00583 return true; 00584 } 00585 } 00586 return false; 00587 } 00588 00589 bool ESP8266::send(int id, String str) 00590 { 00591 m_uart.print("AT+CIPSEND="); 00592 00593 m_uart.print(String(id)); 00594 m_uart.print(","); 00595 m_uart.println(str.length()); 00596 unsigned long start; 00597 start = millis(); 00598 bool found = false; 00599 while (millis()-start<5000) { 00600 if(m_uart.find(">")==true ) 00601 { 00602 found = true; 00603 break; 00604 } 00605 } 00606 if(found) 00607 m_uart.print(str); 00608 else 00609 { 00610 closeMux(id); 00611 return false; 00612 } 00613 00614 00615 String data; 00616 start = millis(); 00617 while (millis()-start<5000) { 00618 if(m_uart.available()>0) 00619 { 00620 char a =m_uart.readChr(); 00621 data=data+a; 00622 } 00623 if (data.indexOf("SEND OK")!=-1) 00624 { 00625 return true; 00626 } 00627 } 00628 return false; 00629 } 00630 00631 00632 void ESP8266::closeMux(void) 00633 { 00634 m_uart.println("AT+CIPCLOSE"); 00635 00636 String data; 00637 unsigned long start; 00638 start = millis(); 00639 while (millis()-start<3000) { 00640 if(m_uart.available()>0) 00641 { 00642 char a =m_uart.readChr(); 00643 data=data+a; 00644 } 00645 if (data.indexOf("Linked")!=-1 || data.indexOf("ERROR")!=-1 || data.indexOf("we must restart")!=-1) 00646 { 00647 break; 00648 } 00649 } 00650 } 00651 00652 void ESP8266::closeMux(int id) 00653 { 00654 m_uart.print("AT+CIPCLOSE="); 00655 m_uart.println(String(id)); 00656 String data; 00657 unsigned long start; 00658 start = millis(); 00659 while (millis()-start<3000) { 00660 if(m_uart.available()>0) 00661 { 00662 char a =m_uart.readChr(); 00663 data=data+a; 00664 } 00665 if (data.indexOf("OK")!=-1 || data.indexOf("Link is not")!=-1 || data.indexOf("Cant close")!=-1) 00666 { 00667 break; 00668 } 00669 } 00670 00671 } 00672 00673 String ESP8266::showIP(void) 00674 { 00675 bool ret = false; 00676 m_uart.flush(); 00677 m_uart.println("AT+CIFSR"); 00678 00679 String data; 00680 unsigned long start; 00681 start = millis(); 00682 while (millis()-start < 3000) { 00683 while(m_uart.available() > 0) { 00684 char a = m_uart.readChr(); 00685 data += a; 00686 } 00687 if (data.indexOf("OK") != -1) { 00688 ret = true; 00689 break; 00690 } else if (data.indexOf("ERROR") != -1) { 00691 ret = false; 00692 break; 00693 } 00694 } 00695 //printf("data = [%s]\r\n", data.c_str()); 00696 if (ret) { 00697 int index1 = data.indexOf("AT+CIFSR\r\r\n"); 00698 int index2 = data.indexOf("\r\n\r\nOK"); 00699 if (index1 != -1 && index2 != -1) { 00700 return data.substring(index1 + strlen("AT+CIFSR\r\r\n"), index2); 00701 } else { 00702 return "not found"; 00703 } 00704 } else { 00705 return "null"; 00706 } 00707 } 00708 00709 bool ESP8266::confServer(int mode, int port) 00710 { 00711 m_uart.print("AT+CIPSERVER="); 00712 m_uart.print(String(mode)); 00713 m_uart.print(","); 00714 m_uart.println(String(port)); 00715 00716 String data; 00717 unsigned long start; 00718 start = millis(); 00719 bool found = false; 00720 while (millis()-start<3000) { 00721 if(m_uart.available()>0) 00722 { 00723 char a =m_uart.readChr(); 00724 data=data+a; 00725 } 00726 if (data.indexOf("OK")!=-1 || data.indexOf("no charge")!=-1) 00727 { 00728 found = true; 00729 break; 00730 } 00731 } 00732 m_uart.flush(); 00733 return found; 00734 }
Generated on Tue Jul 12 2022 18:59:49 by
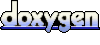