GROVE i2c color sensor Library. based on https://github.com/Seeed-Studio/Grove_I2C_Color_Sensor_TCS3472
Dependents: PROJ 2PA2S 2PA2S_v2 2PA2S-interrupteur
Adafruit_TCS34725.h
00001 /**************************************************************************/ 00002 /*! 00003 @file Adafruit_TCS34725.h 00004 @author KTOWN (Adafruit Industries) 00005 00006 @section LICENSE 00007 00008 Software License Agreement (BSD License) 00009 00010 Copyright (c) 2013, Adafruit Industries 00011 All rights reserved. 00012 00013 Redistribution and use in source and binary forms, with or without 00014 modification, are permitted provided that the following conditions are met: 00015 1. Redistributions of source code must retain the above copyright 00016 notice, this list of conditions and the following disclaimer. 00017 2. Redistributions in binary form must reproduce the above copyright 00018 notice, this list of conditions and the following disclaimer in the 00019 documentation and/or other materials provided with the distribution. 00020 3. Neither the name of the copyright holders nor the 00021 names of its contributors may be used to endorse or promote products 00022 derived from this software without specific prior written permission. 00023 00024 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS ''AS IS'' AND ANY 00025 EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00026 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER BE LIABLE FOR ANY 00028 DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00029 (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00030 LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00031 ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00032 (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00033 SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 */ 00035 /**************************************************************************/ 00036 #ifndef Adafruit_TCS34725_H_ 00037 #define Adafruit_TCS34725_H_ 00038 00039 #include "mbed.h" 00040 00041 #define TCS34725_ADDRESS (0x29<<1) 00042 00043 #define TCS34725_COMMAND_BIT (0x80) 00044 00045 #define TCS34725_ENABLE (0x00) 00046 #define TCS34725_ENABLE_AIEN (0x10) /* RGBC Interrupt Enable */ 00047 #define TCS34725_ENABLE_WEN (0x08) /* Wait enable - Writing 1 activates the wait timer */ 00048 #define TCS34725_ENABLE_AEN (0x02) /* RGBC Enable - Writing 1 actives the ADC, 0 disables it */ 00049 #define TCS34725_ENABLE_PON (0x01) /* Power on - Writing 1 activates the internal oscillator, 0 disables it */ 00050 #define TCS34725_ATIME (0x01) /* Integration time */ 00051 #define TCS34725_WTIME (0x03) /* Wait time (if TCS34725_ENABLE_WEN is asserted) */ 00052 #define TCS34725_WTIME_2_4MS (0xFF) /* WLONG0 = 2.4ms WLONG1 = 0.029s */ 00053 #define TCS34725_WTIME_204MS (0xAB) /* WLONG0 = 204ms WLONG1 = 2.45s */ 00054 #define TCS34725_WTIME_614MS (0x00) /* WLONG0 = 614ms WLONG1 = 7.4s */ 00055 #define TCS34725_AILTL (0x04) /* Clear channel lower interrupt threshold */ 00056 #define TCS34725_AILTH (0x05) 00057 #define TCS34725_AIHTL (0x06) /* Clear channel upper interrupt threshold */ 00058 #define TCS34725_AIHTH (0x07) 00059 #define TCS34725_PERS (0x0C) /* Persistence register - basic SW filtering mechanism for interrupts */ 00060 #define TCS34725_PERS_NONE (0b0000) /* Every RGBC cycle generates an interrupt */ 00061 #define TCS34725_PERS_1_CYCLE (0b0001) /* 1 clean channel value outside threshold range generates an interrupt */ 00062 #define TCS34725_PERS_2_CYCLE (0b0010) /* 2 clean channel values outside threshold range generates an interrupt */ 00063 #define TCS34725_PERS_3_CYCLE (0b0011) /* 3 clean channel values outside threshold range generates an interrupt */ 00064 #define TCS34725_PERS_5_CYCLE (0b0100) /* 5 clean channel values outside threshold range generates an interrupt */ 00065 #define TCS34725_PERS_10_CYCLE (0b0101) /* 10 clean channel values outside threshold range generates an interrupt */ 00066 #define TCS34725_PERS_15_CYCLE (0b0110) /* 15 clean channel values outside threshold range generates an interrupt */ 00067 #define TCS34725_PERS_20_CYCLE (0b0111) /* 20 clean channel values outside threshold range generates an interrupt */ 00068 #define TCS34725_PERS_25_CYCLE (0b1000) /* 25 clean channel values outside threshold range generates an interrupt */ 00069 #define TCS34725_PERS_30_CYCLE (0b1001) /* 30 clean channel values outside threshold range generates an interrupt */ 00070 #define TCS34725_PERS_35_CYCLE (0b1010) /* 35 clean channel values outside threshold range generates an interrupt */ 00071 #define TCS34725_PERS_40_CYCLE (0b1011) /* 40 clean channel values outside threshold range generates an interrupt */ 00072 #define TCS34725_PERS_45_CYCLE (0b1100) /* 45 clean channel values outside threshold range generates an interrupt */ 00073 #define TCS34725_PERS_50_CYCLE (0b1101) /* 50 clean channel values outside threshold range generates an interrupt */ 00074 #define TCS34725_PERS_55_CYCLE (0b1110) /* 55 clean channel values outside threshold range generates an interrupt */ 00075 #define TCS34725_PERS_60_CYCLE (0b1111) /* 60 clean channel values outside threshold range generates an interrupt */ 00076 #define TCS34725_CONFIG (0x0D) 00077 #define TCS34725_CONFIG_WLONG (0x02) /* Choose between short and long (12x) wait times via TCS34725_WTIME */ 00078 #define TCS34725_CONTROL (0x0F) /* Set the gain level for the sensor */ 00079 #define TCS34725_ID (0x12) /* 0x44 = TCS34721/TCS34725, 0x4D = TCS34723/TCS34727 */ 00080 #define TCS34725_STATUS (0x13) 00081 #define TCS34725_STATUS_AINT (0x10) /* RGBC Clean channel interrupt */ 00082 #define TCS34725_STATUS_AVALID (0x01) /* Indicates that the RGBC channels have completed an integration cycle */ 00083 #define TCS34725_CDATAL (0x14) /* Clear channel data */ 00084 #define TCS34725_CDATAH (0x15) 00085 #define TCS34725_RDATAL (0x16) /* Red channel data */ 00086 #define TCS34725_RDATAH (0x17) 00087 #define TCS34725_GDATAL (0x18) /* Green channel data */ 00088 #define TCS34725_GDATAH (0x19) 00089 #define TCS34725_BDATAL (0x1A) /* Blue channel data */ 00090 #define TCS34725_BDATAH (0x1B) 00091 00092 typedef enum 00093 { 00094 TCS34725_INTEGRATIONTIME_2_4MS = 0xFF, /**< 2.4ms - 1 cycle - Max Count: 1024 */ 00095 TCS34725_INTEGRATIONTIME_24MS = 0xF6, /**< 24ms - 10 cycles - Max Count: 10240 */ 00096 TCS34725_INTEGRATIONTIME_50MS = 0xEB, /**< 50ms - 20 cycles - Max Count: 20480 */ 00097 TCS34725_INTEGRATIONTIME_101MS = 0xD5, /**< 101ms - 42 cycles - Max Count: 43008 */ 00098 TCS34725_INTEGRATIONTIME_154MS = 0xC0, /**< 154ms - 64 cycles - Max Count: 65535 */ 00099 TCS34725_INTEGRATIONTIME_700MS = 0x00 /**< 700ms - 256 cycles - Max Count: 65535 */ 00100 } 00101 tcs34725IntegrationTime_t ; 00102 00103 typedef enum 00104 { 00105 TCS34725_GAIN_1X = 0x00, /**< No gain */ 00106 TCS34725_GAIN_4X = 0x01, /**< 2x gain */ 00107 TCS34725_GAIN_16X = 0x02, /**< 16x gain */ 00108 TCS34725_GAIN_60X = 0x03 /**< 60x gain */ 00109 } 00110 tcs34725Gain_t ; 00111 00112 class Adafruit_TCS34725 { 00113 public: 00114 Adafruit_TCS34725(I2C *i2c, tcs34725IntegrationTime_t = TCS34725_INTEGRATIONTIME_2_4MS, tcs34725Gain_t = TCS34725_GAIN_1X); 00115 00116 bool begin(void); 00117 void setIntegrationTime(tcs34725IntegrationTime_t it); 00118 void setGain(tcs34725Gain_t gain); 00119 void getRawData(uint16_t *r, uint16_t *g, uint16_t *b, uint16_t *c); 00120 uint16_t calculateColorTemperature(uint16_t r, uint16_t g, uint16_t b); 00121 uint16_t calculateLux(uint16_t r, uint16_t g, uint16_t b); 00122 void write8 (uint8_t reg, uint32_t value); 00123 uint8_t read8 (uint8_t reg); 00124 uint16_t read16 (uint8_t reg); 00125 void setInterrupt(bool flag); 00126 void clearInterrupt(void); 00127 void setIntLimits(uint16_t l, uint16_t h); 00128 void enable(void); 00129 00130 private: 00131 bool _tcs34725Initialised; 00132 tcs34725Gain_t _tcs34725Gain; 00133 tcs34725IntegrationTime_t _tcs34725IntegrationTime; 00134 00135 I2C *_i2c; 00136 00137 void disable(void); 00138 }; 00139 00140 #endif
Generated on Sun Jul 17 2022 20:00:18 by
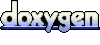