
few changes for RTS/CTS control
Dependencies: MTS-Serial libmDot mbed-rtos mbed
Fork of mDot_AT_firmware by
Command.cpp
00001 #include "Command.h" 00002 #include <algorithm> 00003 00004 const char Command::newline[] = "\r\n"; 00005 00006 Command::Command(mDot* dot) : _dot(dot), _name(""), _text(""), _desc("") 00007 { 00008 _usage = "NONE"; 00009 _queryable = false; 00010 } 00011 00012 Command::Command(mDot* dot, const char* name, const char* text, const char* desc) : 00013 _dot(dot), _name(name), _text(text), _desc(desc) 00014 { 00015 _usage = "NONE"; 00016 _queryable = false; 00017 } 00018 00019 std::string &Command::errorMessage() 00020 { 00021 return _errorMessage; 00022 } 00023 00024 void Command::setErrorMessage(const char* message) 00025 { 00026 _errorMessage.assign(message); 00027 } 00028 00029 void Command::setErrorMessage(const std::string& message) 00030 { 00031 _errorMessage.assign(message); 00032 } 00033 00034 std::string Command::usage() const 00035 { 00036 std::string usage(_text); 00037 usage.append(": "); 00038 usage.append(_usage); 00039 return usage; 00040 } 00041 00042 bool Command::queryable() const 00043 { 00044 return _queryable; 00045 } 00046 00047 void Command::readByteArray(const std::string& input, std::vector<uint8_t>& out, size_t len) 00048 { 00049 // if input length is greater than expected byte output 00050 // there must be a delimiter included 00051 if (input.length() > len * 2) 00052 { 00053 std::vector < std::string > bytes; 00054 if (input.find(" ") != std::string::npos) 00055 bytes = mts::Text::split(input, " "); 00056 else if (input.find(":") != std::string::npos) 00057 bytes = mts::Text::split(input, ":"); 00058 else if (input.find("-") != std::string::npos) 00059 bytes = mts::Text::split(input, "-"); 00060 else if (input.find(".") != std::string::npos) 00061 bytes = mts::Text::split(input, "."); 00062 00063 if (bytes.size() != len) { 00064 return; 00065 } 00066 00067 int temp; 00068 // Read in the key components... 00069 for (size_t i = 0; i < len; i++) 00070 { 00071 sscanf(bytes[i].c_str(), "%02x", &temp); 00072 out.push_back(temp); 00073 } 00074 } 00075 else 00076 { 00077 // no delims 00078 int temp; 00079 00080 // Read in the key components... 00081 for (size_t i = 0; i < len; i++) 00082 { 00083 if (i * 2 < input.size()) 00084 { 00085 sscanf(input.substr(i * 2).c_str(), "%02x", &temp); 00086 out.push_back(temp); 00087 } 00088 } 00089 } 00090 } 00091 00092 bool Command::isHexString(const std::string& str, size_t bytes) { 00093 int numDelims = bytes - 1; 00094 size_t minSize = bytes * 2; 00095 size_t maxSize = minSize + numDelims; 00096 00097 if (str.size() == minSize) { 00098 return str.find_first_not_of("0123456789abcdefABCDEF") == std::string::npos; 00099 } 00100 else if (str.size() == maxSize) { 00101 if (str.find_first_of(":-.") == std::string::npos) { 00102 // no delim found 00103 return false; 00104 } 00105 if (str.find(":") != std::string::npos && std::count(str.begin(), str.end(), ':') != numDelims) { 00106 return false; 00107 } 00108 if (str.find(".") != std::string::npos && std::count(str.begin(), str.end(), '.') != numDelims) { 00109 return false; 00110 } 00111 if (str.find("-") != std::string::npos && std::count(str.begin(), str.end(), '-') != numDelims) { 00112 return false; 00113 } 00114 00115 return str.find_first_not_of("0123456789abcdefABCDEF:-.") == std::string::npos; 00116 } 00117 00118 return false; 00119 } 00120 00121 bool Command::verify(std::vector<std::string> args) { 00122 if (args.size() == 1) 00123 return true; 00124 00125 setErrorMessage("Invalid arguments"); 00126 return false; 00127 } 00128
Generated on Tue Jul 12 2022 16:01:24 by
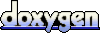