
few changes for RTS/CTS control
Dependencies: MTS-Serial libmDot mbed-rtos mbed
Fork of mDot_AT_firmware by
CommandTerminal.h
00001 /** 00002 ****************************************************************************** 00003 * File Name : command.h 00004 * Date : 18/04/2014 10:57:12 00005 * Description : This file provides code for command line prompt 00006 ****************************************************************************** 00007 * 00008 * COPYRIGHT(c) 2014 MultiTech Systems, Inc. 00009 * 00010 * Redistribution and use in source and binary forms, with or without modification, 00011 * are permitted provided that the following conditions are met: 00012 * 1. Redistributions of source code must retain the above copyright notice, 00013 * this list of conditions and the following disclaimer. 00014 * 2. Redistributions in binary form must reproduce the above copyright notice, 00015 * this list of conditions and the following disclaimer in the documentation 00016 * and/or other materials provided with the distribution. 00017 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00018 * may be used to endorse or promote products derived from this software 00019 * without specific prior written permission. 00020 * 00021 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00022 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00023 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00024 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00025 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00026 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00027 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00028 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00029 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00030 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00031 * 00032 ****************************************************************************** 00033 */ 00034 00035 #include "mbed.h" 00036 #include "ATSerial.h" 00037 #include "MTSSerial.h" 00038 #include "MTSSerialFlowControl.h" 00039 #include "Commands.h" 00040 #include "mDot.h" 00041 #include "mDotEvent.h" 00042 00043 /* Define to prevent recursive inclusion -------------------------------------*/ 00044 #ifndef __command_terminal_H__ 00045 #define __command_terminal_H__ 00046 00047 class CommandTerminal { 00048 00049 class RadioEvent : public mDotEvent { 00050 00051 mts::ATSerial& _serial; 00052 public: 00053 RadioEvent(mts::ATSerial& serial) : _serial(serial) {} 00054 00055 virtual ~RadioEvent() {} 00056 00057 /*! 00058 * MAC layer event callback prototype. 00059 * 00060 * \param [IN] flags Bit field indicating the MAC events occurred 00061 * \param [IN] info Details about MAC events occurred 00062 */ 00063 virtual void MacEvent(LoRaMacEventFlags *flags, LoRaMacEventInfo *info); 00064 00065 virtual uint8_t MeasureBattery(void) { 00066 return 255; 00067 } 00068 }; 00069 00070 00071 public: 00072 00073 enum WaitType { 00074 WAIT_JOIN, 00075 WAIT_RECV, 00076 WAIT_LINK, 00077 WAIT_SEND, 00078 WAIT_NA 00079 }; 00080 00081 CommandTerminal(mts::ATSerial& serial, mDot* dot); 00082 virtual ~CommandTerminal(); 00083 00084 // Command prompt text... 00085 static const char banner[]; 00086 static const char helpline[]; 00087 static const char prompt[]; 00088 00089 // Command error text... 00090 static const char command_error[]; 00091 00092 // Response texts... 00093 static const char help[]; 00094 static const char cmd_error[]; 00095 static const char newline[]; 00096 static const char connect[]; 00097 static const char no_carrier[]; 00098 static const char done[]; 00099 static const char error[]; 00100 00101 // Escape sequence 00102 static const char escape_sequence[]; 00103 00104 static std::string formatPacketData(const std::vector<uint8_t>& data, const uint8_t& format); 00105 static bool waitForEscape(int timeout, mDot* dot=NULL, WaitType wait=WAIT_NA); 00106 00107 void start(); 00108 00109 private: 00110 00111 static void idle(void const* args) { 00112 while (1) 00113 __WFI(); 00114 } 00115 00116 mts::ATSerial& _serial; 00117 static mts::ATSerial* _serialp; 00118 00119 mDot* _dot; 00120 CommandTerminal::RadioEvent* _events; 00121 mDot::Mode _mode; 00122 std::vector<Command*> _commands; 00123 Thread _idle_thread; 00124 bool _sleep_standby; 00125 DigitalOut _xbee_on_sleep; 00126 00127 void addCommand(Command* cmd); 00128 00129 void serialLoop(); 00130 bool autoJoinCheck(); 00131 00132 void printHelp(); 00133 00134 bool readable(); 00135 bool writeable(); 00136 char read(); 00137 void write(const char* message); 00138 void writef(const char* format, ... ); 00139 00140 void sleep(bool standby); 00141 void wakeup(void); 00142 00143 }; 00144 00145 #endif // __command_terminal_H__ 00146
Generated on Tue Jul 12 2022 16:01:24 by
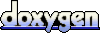