
few changes for RTS/CTS control
Dependencies: MTS-Serial libmDot mbed-rtos mbed
Fork of mDot_AT_firmware by
CmdUplinkCounter.cpp
00001 #include "CmdUplinkCounter.h" 00002 00003 CmdUplinkCounter::CmdUplinkCounter(mDot* dot, mts::MTSSerial& serial) 00004 : 00005 Command(dot, "Uplink Counter", "AT+ULC", "Get or set the uplink counter for the next packet"), 00006 _serial(serial) { 00007 _help = std::string(text()) + ": " + std::string(desc()); 00008 _usage = "(0-4294967295)"; 00009 _queryable = true; 00010 } 00011 00012 uint32_t CmdUplinkCounter::action(std::vector<std::string> args) { 00013 if (args.size() == 1) { 00014 if (_dot->getVerbose()) 00015 _serial.writef("Uplink Counter: "); 00016 00017 _serial.writef("%u\r\n", _dot->getUpLinkCounter()); 00018 } else if (args.size() == 2) { 00019 int32_t code; 00020 int count; 00021 sscanf(args[1].c_str(), "%d", &count); 00022 00023 if ((code = _dot->setUpLinkCounter(count)) != mDot::MDOT_OK) { 00024 setErrorMessage(_dot->getLastError());; 00025 return 1; 00026 } 00027 } 00028 return 0; 00029 } 00030 00031 bool CmdUplinkCounter::verify(std::vector<std::string> args) { 00032 if (args.size() == 1) 00033 return true; 00034 00035 if (args.size() == 2) { 00036 00037 int count; 00038 if (sscanf(args[1].c_str(), "%d", &count) == 1) { 00039 if (count > 4294967295) { 00040 setErrorMessage("Invalid uplink counter, expects (0-4294967295)"); 00041 return false; 00042 } 00043 return true; 00044 } 00045 } 00046 00047 setErrorMessage("Invalid arguments"); 00048 return false; 00049 } 00050
Generated on Tue Jul 12 2022 16:01:24 by
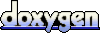