
few changes for RTS/CTS control
Dependencies: MTS-Serial libmDot mbed-rtos mbed
Fork of mDot_AT_firmware by
ATSerial.h
00001 #ifndef ATSERIAL_H 00002 #define ATSERIAL_H 00003 00004 #include "MTSSerial.h" 00005 #include "MTSBufferedIO.h" 00006 00007 namespace mts 00008 { 00009 00010 /** This class derives from MTSBufferedIO and provides a buffered wrapper to the 00011 * standard mbed Serial class. Since it depends only on the mbed Serial class for 00012 * accessing serial data, this class is inherently portable accross different mbed 00013 * platforms. 00014 */ 00015 class ATSerial : public MTSSerial 00016 { 00017 public: 00018 /** Creates a new ATSerial object that can be used to talk to an mbed serial port 00019 * through internal SW buffers. 00020 * 00021 * @param TXD the transmit data pin on the desired mbed Serial interface. 00022 * @param RXD the receive data pin on the desired mbed Serial interface. 00023 * @param txBufferSize the size in bytes of the internal SW transmit buffer. The 00024 * default is 256 bytes. 00025 * @param rxBufferSize the size in bytes of the internal SW receive buffer. The 00026 * default is 256 bytes. 00027 */ 00028 ATSerial(PinName TXD, PinName RXD, int txBufferSize = 256, int rxBufferSize = 256); 00029 00030 /** Destructs an ATSerial object and frees all related resources, including 00031 * internal buffers. 00032 */ 00033 virtual ~ATSerial(); 00034 00035 /** 00036 * Attach the internal serial object to provided pins 00037 * @param TXD the transmit data pin on the desired mbed Serial interface. 00038 * @param RXD the receive data pin on the desired mbed Serial interface. 00039 */ 00040 void reattach(PinName TXD, PinName RXD); 00041 00042 /** This method is used to the set the baud rate of the serial port. 00043 * 00044 * @param baudrate the baudrate in bps as an int. The default is 9600 bps. 00045 */ 00046 void baud(int baudrate); 00047 00048 /** This method sets the transmission format used by the serial port. 00049 * 00050 * @param bits the number of bits in a word (5-8; default = 8) 00051 * @param parity the parity used (SerialBase::None, SerialBase::Odd, SerialBase::Even, 00052 * SerialBase::Forced1, SerialBase::Forced0; default = SerialBase::None) 00053 * @param stop the number of stop bits (1 or 2; default = 1) 00054 */ 00055 void format(int bits=8, SerialBase::Parity parity=mbed::SerialBase::None, int stop_bits=1); 00056 00057 /** Generate a break condition on the serial line 00058 */ 00059 void sendBreak(); 00060 00061 /** Check for escape sequence detected on serial input 00062 * @return true if escape sequence was seen 00063 */ 00064 bool escaped(); 00065 00066 void escapeChar(char esc); 00067 00068 char escapeChar(); 00069 00070 void clearEscaped(); 00071 00072 00073 protected: 00074 00075 RawSerial* _serial; // Internal mbed Serial object 00076 int _baudrate; 00077 int _bits; 00078 SerialBase::Parity _parity; 00079 int _stop_bits; 00080 Timer timer; 00081 int _last_time; 00082 int _esc_cnt; 00083 char _esc_ch; 00084 bool _escaped; 00085 00086 virtual void handleWrite(); // Method for handling data to be written 00087 virtual void handleRead(); // Method for handling data to be read 00088 00089 00090 }; 00091 00092 } 00093 00094 #endif /* ATSERIAL_H */
Generated on Tue Jul 12 2022 16:01:22 by
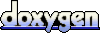