
few changes for RTS/CTS control
Dependencies: MTS-Serial libmDot mbed-rtos mbed
Fork of mDot_AT_firmware by
ATSerialFlowControl.cpp
00001 #include "mbed.h" 00002 #include "ATSerialFlowControl.h" 00003 #include "MTSLog.h" 00004 #include "Utils.h" 00005 00006 using namespace mts; 00007 00008 ATSerialFlowControl::ATSerialFlowControl(PinName TXD, PinName RXD, PinName RTS, PinName CTS, int txBufSize, int rxBufSize) 00009 : ATSerial(TXD, RXD, txBufSize, rxBufSize) 00010 , rxReadyFlag(false) 00011 , rts(RTS) 00012 , cts(CTS) 00013 , dtr(PA_11) 00014 { 00015 notifyStartSending(); 00016 00017 // Calculate the high and low watermark values 00018 highThreshold = mts_max(rxBufSize - 10, rxBufSize * 0.85); 00019 lowThreshold = rxBufSize * 0.3; 00020 00021 // Setup the low watermark callback on the internal receive buffer 00022 rxBuffer.attach(this, &ATSerialFlowControl::notifyStartSending, lowThreshold, LESS); 00023 } 00024 00025 ATSerialFlowControl::~ATSerialFlowControl() 00026 { 00027 } 00028 00029 //Override the rxClear function to make sure that flow control lines are set correctly. 00030 void ATSerialFlowControl::rxClear() 00031 { 00032 MTSBufferedIO::rxClear(); 00033 notifyStartSending(); 00034 } 00035 00036 void ATSerialFlowControl::notifyStartSending() 00037 { 00038 if(!rxReadyFlag) { 00039 rts.write(0); 00040 rxReadyFlag = true; 00041 //printf("RTS LOW: READY - RX[%d/%d]\r\n", rxBuffer.size(), rxBuffer.capacity()); 00042 } 00043 } 00044 00045 void ATSerialFlowControl::notifyStopSending() 00046 { 00047 if(rxReadyFlag) { 00048 rts.write(1); 00049 rxReadyFlag = false; 00050 //printf("RTS HIGH: NOT-READY - RX[%d/%d]\r\n", rxBuffer.size(), rxBuffer.capacity()); 00051 } 00052 } 00053 00054 void ATSerialFlowControl::handleRead() 00055 { 00056 ATSerial::handleRead(); 00057 if (rxBuffer.size() >= highThreshold) { 00058 notifyStopSending(); 00059 } 00060 } 00061 00062 void ATSerialFlowControl::handleWrite() 00063 { 00064 while(txBuffer.size() != 0) { 00065 if (serial.writeable() && cts.read() == 0) { 00066 dtr.write(0); 00067 char byte; 00068 if(txBuffer.read(byte) == 1) { 00069 serial.attach(NULL, Serial::RxIrq); 00070 serial.putc(byte); 00071 serial.attach(this, &ATSerialFlowControl::handleRead, Serial::RxIrq); 00072 } 00073 } 00074 else { 00075 if (cts.read() == 1) dtr.write(1); 00076 return; 00077 } 00078 } 00079 } 00080
Generated on Tue Jul 12 2022 16:01:22 by
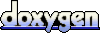