
comit to publish
Dependencies: CANnucleo CANnucleo_Hello mbed
Fork of CANnucleo_Hello by
main.cpp
00001 /* 00002 * An example showing how to use the CANnucleo library: 00003 * 00004 * Two affordable (less than $3 on ebay) STM32F103C8T6 boards (20kB SRAM, 64kB Flash), 00005 * (see [https://developer.mbed.org/users/hudakz/code/STM32F103C8T6_Hello/] for more details) 00006 * are connected to the same CAN bus via transceivers (MCP2551 or TJA1040, or etc.). 00007 * CAN transceivers are not part of NUCLEO boards, therefore must be added by you. 00008 * Remember also that CAN bus (even a short one) must be terminated with 120 Ohm resitors at both ends. 00009 * 00010 * For more details see the wiki page <https://developer.mbed.org/users/hudakz/code/CANnucleo_Hello/> 00011 * 00012 * NOTE: If you'd like to use the official NUCLEO boards comment out line 22 00013 * 00014 * The same code is used for both NUCLEO boards, but: 00015 * For board #1 compile the example without any change. 00016 * For board #2 comment out line 23 before compiling 00017 * 00018 * Once the binaries have been downloaded to the boards reset board #1. 00019 * 00020 */ 00021 00022 //#define TARGET_STM32F103C8T6 1 // uncomment this line when using STM32F103C8T6 boards! 00023 //#define BOARD1 1 // comment out this line when compiling for board #2 00024 00025 00026 #define start_charge_msg "\x22\x80\x24\x01" 00027 #define stop_charge_msg "\x22\x80\x24\x02" 00028 #define charger_startup_msg "\x0\xB\x0\x0\x0\x0\x0\xB" // charger writes this during startup 00029 #define change_charger_can_bitrate_1Mbs "\x22\xDA\x20\x01\x04" 00030 00031 const unsigned int RX_ID = 0x100; 00032 const unsigned int TX_ID = 0x101; 00033 00034 #include "CANnucleo.h" 00035 #include "mbed.h" 00036 00037 /* 00038 * To avaoid name collision with the CAN and CANMessage classes built into the mbed library 00039 * the CANnucleo's CAN and CANMessage classes have been moved into the CANnucleo namespace. 00040 * Remember to qualify them with the CANnucleo namespace. 00041 */ 00042 CANnucleo::CAN can(PA_11, PA_12); // CAN Rx pin name, CAN Tx pin name 00043 CANnucleo::CANMessage rxMsg; 00044 CANnucleo::CANMessage txMsg; 00045 CANnucleo::CANMessage throttle_txMsg; 00046 00047 00048 DigitalOut led(PA_5); 00049 DigitalOut controller_key_switch(PA_6); // SS_Rel 00050 00051 Timer timer; 00052 int counter = 0; 00053 volatile bool msgAvailable = false; 00054 volatile bool to_send = false; 00055 00056 /** 00057 * @brief 'CAN receive-complete' interrup handler. 00058 * @note Called on arrival of new CAN message. 00059 * Keep it as short as possible. 00060 * @param 00061 * @retval 00062 */ 00063 void onMsgReceived() 00064 { 00065 msgAvailable = true; 00066 } 00067 00068 /** 00069 * @brief Main 00070 * @note 00071 * @param 00072 * @retval 00073 */ 00074 bool to_charge = 0; 00075 bool charge = 0; 00076 void led_error() 00077 { 00078 00079 led = !led; 00080 wait(0.1); 00081 led=!led; 00082 wait(0.1); 00083 led = !led; 00084 wait(0.1); 00085 led = !led; 00086 wait(0.1); 00087 led = !led; 00088 00089 } 00090 int build_message(unsigned char * badjoras, const char * command) 00091 { 00092 char *src; 00093 strcpy(src,command); 00094 strncpy((char *) badjoras,src,sizeof(command)-1); 00095 return (sizeof(command)-1); 00096 } 00097 00098 void flip() 00099 { 00100 to_send=1; // append first data item 00101 to_charge=1; 00102 //controller_key_switch = !controller_key_switch; 00103 led = !led; 00104 charge = !charge; 00105 } 00106 00107 bool controller = 0;//flag for turning on controller_key_switch 00108 Ticker flipper; 00109 void change_can_bitrate(){ 00110 to_send= 1; 00111 } 00112 InterruptIn button(PC_13); 00113 00114 int main() 00115 00116 { 00117 can.frequency(125000); // set bit rate to 1Mbps 00118 can.attach(&onMsgReceived); // attach 'CAN receive-complete' interrupt handler 00119 flipper.attach(&change_can_bitrate, 5); //heartbeat 00120 button.rise(&flip); 00121 led=0; 00122 printf("badjoras\n"); 00123 00124 while(true) { 00125 00126 if(msgAvailable) { 00127 msgAvailable = false; // reset flag for next use 00128 int len = can.read(rxMsg); 00129 printf("Id: %x ", rxMsg.id); 00130 for(int i = 0; i<len; i++) { 00131 printf("%x,", rxMsg.data[i]); 00132 } 00133 printf("\n\r"); 00134 /* 00135 rxMsg >> controller; 00136 led = controller; 00137 controller_key_switch = controller;*/ 00138 if(rxMsg.id == 0x28B) { 00139 if (memcmp(rxMsg.data,charger_startup_msg,8)==0) { 00140 printf(" this is the message\n\r"); 00141 txMsg.clear(); // clear Tx message storage 00142 txMsg.id = 0x60B; 00143 build_message(txMsg.data,stop_charge_msg); 00144 txMsg.len = 4; 00145 to_send = 1; 00146 } 00147 } 00148 } 00149 if(to_send) { 00150 to_send = 0; 00151 if(to_charge) { 00152 to_charge = 0; 00153 if(charge) { 00154 txMsg.clear(); // clear Tx message storage 00155 txMsg.id = 0x60B; 00156 build_message(txMsg.data,start_charge_msg); 00157 txMsg.len = 4; 00158 } else { 00159 txMsg.clear(); // clear Tx message storage 00160 txMsg.id = 0x60B; 00161 build_message(txMsg.data,stop_charge_msg); 00162 txMsg.len = 4; 00163 } 00164 00165 } else { 00166 txMsg.clear(); 00167 txMsg.id = 0x60B; 00168 build_message(txMsg.data, change_charger_can_bitrate_1Mbs); 00169 txMsg.len = 8; 00170 } 00171 if(!can.write(txMsg)) { 00172 led_error(); 00173 } else { 00174 printf("sent message: ID: %x DATA: %x %x %x %x %x %x %x %x\n\r",txMsg.id,txMsg.data[0],txMsg.data[1],txMsg.data[2],txMsg.data[3],txMsg.data[4],txMsg.data[5],txMsg.data[6],txMsg.data[7]); 00175 } 00176 } 00177 } 00178 } 00179 00180 00181
Generated on Thu Jul 28 2022 23:45:27 by
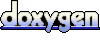