
This is an MBED controlled liquid dispensing robot. With applications in bars.
Dependencies: EthernetInterface WebSocketClient mbed-rtos mbed
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetInterface.h" 00003 #include "Websocket.h" 00004 #include "stdio.h" 00005 #include "string.h" 00006 00007 //Used to Log to the PC 00008 Serial pc(USBTX,USBRX); 00009 00010 //Input Pin for the Paddle Wheel Flow Meter 00011 DigitalIn pulse(p8); 00012 00013 // Pin Outs for Solenoids 00014 DigitalOut Ctrl(p9); 00015 DigitalOut Ctrl2(p10); 00016 00017 // 00018 #define PULSE_VOL 4.50 // More flow due to angle of paddle wheel Pour 1 00019 #define PULSE_VOL2 6.75 // Less flow due to angle of paddle wheel Pour 2 00020 00021 //Function Declarations 00022 void pourOne(float milliliters); 00023 void pourTwo(float milliliters); 00024 00025 /* 00026 The main function opens a web socket client and listens for a preset series 00027 of recipies. If one is found, it calls functions to pour liquid. 00028 */ 00029 int main() { 00030 pc.printf("Starting BarBot!\r\n"); 00031 00032 // Close Both Solenoids 00033 Ctrl = 0; 00034 Ctrl2 = 0; 00035 00036 // Receive Buffer 00037 char recv[30]; 00038 char recipe[] = "Rum & Coke"; 00039 char recipe2[] = "Whiskey Ginger"; 00040 char recipe3[] = "Whiskey Neat"; 00041 00042 // Ethernet Interfacing 00043 EthernetInterface eth; 00044 eth.init(); //Use DHCP 00045 eth.connect(); 00046 pc.printf("IP Address is %s\n\r", eth.getIPAddress()); 00047 00048 // Open a Websocket 00049 Websocket ws("ws://sockets.mbed.org:443/ws/sid/ro"); //This Websocket server is provided by MBED for prototyping 00050 ws.connect(); 00051 00052 // Wait to receive an order from websocket 00053 while (1) { 00054 00055 if(ws.read(recv)) { 00056 pc.printf("%s\r\n", recv); 00057 00058 // Recipe based process 00059 if(strcmp(recv, recipe) == 0) { 00060 pourOne(132); 00061 pourTwo(44); 00062 } 00063 else if(strcmp(recv, recipe2) == 0) { 00064 pourOne(0); 00065 pourTwo(88); 00066 } 00067 else if(strcmp(recv, recipe3) == 0) { 00068 pourOne(88); 00069 pourTwo(0); 00070 } 00071 } 00072 00073 wait(1.0); 00074 } 00075 00076 } 00077 00078 void pourOne(float milliliters) { 00079 00080 pc.printf("Pour One\r\n"); 00081 00082 //pouring an empty amount (neat drinks) 00083 if(milliliters <= 0) { 00084 return; 00085 } 00086 00087 int pulses = 0; 00088 bool lastPulse = pulse; 00089 bool thisPulse; 00090 float volume = 0; 00091 00092 //open solenoid one 00093 Ctrl = 1; 00094 00095 while(volume < milliliters) { 00096 thisPulse = pulse; 00097 00098 //check to see if pulse has changed since the last time 00099 if(thisPulse != lastPulse) { 00100 pulses += 1; 00101 } 00102 lastPulse = thisPulse; 00103 00104 //increase the volume 00105 volume = pulses * PULSE_VOL; 00106 00107 pc.printf("Poured %f From First Bottle\r\n", volume); 00108 } 00109 00110 00111 //close solenoid one 00112 Ctrl = 0; 00113 } 00114 00115 void pourTwo(float milliliters) { 00116 00117 pc.printf("Pour Two\r\n"); 00118 00119 //pouring an empty amount (neat drinks) 00120 if(milliliters <= 0) { 00121 return; 00122 } 00123 00124 int pulses = 0; 00125 bool lastPulse = pulse; 00126 bool thisPulse; 00127 float volume = 0; 00128 00129 //open solenoid two 00130 Ctrl2 = 1; 00131 00132 while(volume < milliliters) { 00133 thisPulse = pulse; 00134 00135 //check to see if pulse has changed since last time 00136 if(thisPulse != lastPulse) { 00137 pulses += 1; 00138 } 00139 00140 lastPulse = thisPulse; 00141 00142 //increase the volume 00143 volume = pulses * PULSE_VOL2; 00144 00145 pc.printf("Poured %f From Second Bottle\r\n", volume); 00146 } 00147 00148 //close solenoid two 00149 Ctrl2 = 0; 00150 }
Generated on Sat Jul 16 2022 20:47:13 by
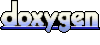