Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
SPISlave.h
00001 /* mbed Microcontroller Library - SPISlave 00002 * Copyright (c) 2010-2011 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_SPISLAVE_H 00006 #define MBED_SPISLAVE_H 00007 00008 #include "device.h" 00009 00010 #if DEVICE_SPISLAVE 00011 00012 #include "platform.h" 00013 #include "PinNames.h" 00014 #include "PeripheralNames.h" 00015 #include "Base.h" 00016 00017 namespace mbed { 00018 00019 /** A SPI slave, used for communicating with a SPI Master device 00020 * 00021 * The default format is set to 8-bits, mode 0, and a clock frequency of 1MHz 00022 * 00023 * Example: 00024 * @code 00025 * // Reply to a SPI master as slave 00026 * 00027 * #include "mbed.h" 00028 * 00029 * SPISlave device(p5, p6, p7, p8); // mosi, miso, sclk, ssel 00030 * 00031 * int main() { 00032 * device.reply(0x00); // Prime SPI with first reply 00033 * while(1) { 00034 * if(device.receive()) { 00035 * int v = device.read(); // Read byte from master 00036 * v = (v + 1) % 0x100; // Add one to it, modulo 256 00037 * device.reply(v); // Make this the next reply 00038 * } 00039 * } 00040 * } 00041 * @endcode 00042 */ 00043 class SPISlave : public Base { 00044 00045 public: 00046 00047 /** Create a SPI slave connected to the specified pins 00048 * 00049 * Pin Options: 00050 * (5, 6, 7i, 8) or (11, 12, 13, 14) 00051 * 00052 * mosi or miso can be specfied as NC if not used 00053 * 00054 * @param mosi SPI Master Out, Slave In pin 00055 * @param miso SPI Master In, Slave Out pin 00056 * @param sclk SPI Clock pin 00057 * @param ssel SPI chip select pin 00058 * @param name (optional) A string to identify the object 00059 */ 00060 SPISlave(PinName mosi, PinName miso, PinName sclk, PinName ssel, 00061 const char *name = NULL); 00062 00063 /** Configure the data transmission format 00064 * 00065 * @param bits Number of bits per SPI frame (4 - 16) 00066 * @param mode Clock polarity and phase mode (0 - 3) 00067 * 00068 * @code 00069 * mode | POL PHA 00070 * -----+-------- 00071 * 0 | 0 0 00072 * 1 | 0 1 00073 * 2 | 1 0 00074 * 3 | 1 1 00075 * @endcode 00076 */ 00077 void format(int bits, int mode = 0); 00078 00079 /** Set the spi bus clock frequency 00080 * 00081 * @param hz SCLK frequency in hz (default = 1MHz) 00082 */ 00083 void frequency(int hz = 1000000); 00084 00085 /** Polls the SPI to see if data has been received 00086 * 00087 * @returns 00088 * 0 if no data, 00089 * 1 otherwise 00090 */ 00091 int receive(void); 00092 00093 /** Retrieve data from receive buffer as slave 00094 * 00095 * @returns 00096 * the data in the receive buffer 00097 */ 00098 int read(void); 00099 00100 /** Fill the transmission buffer with the value to be written out 00101 * as slave on the next received message from the master. 00102 * 00103 * @param value the data to be transmitted next 00104 */ 00105 void reply(int value); 00106 00107 protected: 00108 00109 SPIName _spi; 00110 00111 int _bits; 00112 int _mode; 00113 int _hz; 00114 00115 }; 00116 00117 } // namespace mbed 00118 00119 #endif 00120 00121 #endif
Generated on Tue Jul 12 2022 11:27:27 by
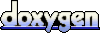