Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
PortOut.h
00001 /* mbed Microcontroller Library - PortOut 00002 * Copyright (c) 2006-2011 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_PORTOUT_H 00006 #define MBED_PORTOUT_H 00007 00008 #include "device.h" 00009 00010 #if DEVICE_PORTOUT 00011 00012 #include "platform.h" 00013 #include "PinNames.h" 00014 #include "Base.h" 00015 00016 #include "PortNames.h" 00017 00018 namespace mbed { 00019 /** A multiple pin digital out 00020 * 00021 * Example: 00022 * @code 00023 * // Toggle all four LEDs 00024 * 00025 * #include "mbed.h" 00026 * 00027 * // LED1 = P1.18 LED2 = P1.20 LED3 = P1.21 LED4 = P1.23 00028 * #define LED_MASK 0x00B40000 00029 * 00030 * PortOut ledport(Port1, LED_MASK); 00031 * 00032 * int main() { 00033 * while(1) { 00034 * ledport = LED_MASK; 00035 * wait(1); 00036 * ledport = 0; 00037 * wait(1); 00038 * } 00039 * } 00040 * @endcode 00041 */ 00042 class PortOut { 00043 public: 00044 00045 /** Create an PortOut, connected to the specified port 00046 * 00047 * @param port Port to connect to (Port0-Port5) 00048 * @param mask A bitmask to identify which bits in the port should be included (0 - ignore) 00049 */ 00050 PortOut(PortName port, int mask = 0xFFFFFFFF); 00051 00052 /** Write the value to the output port 00053 * 00054 * @param value An integer specifying a bit to write for every corresponding PortOut pin 00055 */ 00056 void write(int value); 00057 00058 /** Read the value currently output on the port 00059 * 00060 * @returns 00061 * An integer with each bit corresponding to associated PortOut pin setting 00062 */ 00063 int read(); 00064 00065 /** A shorthand for write() 00066 */ 00067 PortOut& operator= (int value) { 00068 write(value); 00069 return *this; 00070 } 00071 00072 PortOut& operator= (PortOut& rhs) { 00073 write(rhs.read()); 00074 return *this; 00075 } 00076 00077 /** A shorthand for read() 00078 */ 00079 operator int() { 00080 return read(); 00081 } 00082 00083 private: 00084 #if defined(TARGET_LPC1768) || defined(TARGET_LPC2368) 00085 LPC_GPIO_TypeDef *_gpio; 00086 #endif 00087 PortName _port; 00088 uint32_t _mask; 00089 }; 00090 00091 } // namespace mbed 00092 00093 #endif 00094 00095 #endif
Generated on Tue Jul 12 2022 11:27:27 by
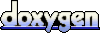