Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
PortInOut.h
00001 /* mbed Microcontroller Library - PortInOut 00002 * Copyright (c) 2006-2011 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_PORTINOUT_H 00006 #define MBED_PORTINOUT_H 00007 00008 #include "device.h" 00009 00010 #if DEVICE_PORTINOUT 00011 00012 #include "PortNames.h" 00013 #include "PinNames.h" 00014 00015 namespace mbed { 00016 00017 /** A multiple pin digital in/out used to set/read multiple bi-directional pins 00018 */ 00019 class PortInOut { 00020 public: 00021 00022 /** Create an PortInOut, connected to the specified port 00023 * 00024 * @param port Port to connect to (Port0-Port5) 00025 * @param mask A bitmask to identify which bits in the port should be included (0 - ignore) 00026 */ 00027 PortInOut(PortName port, int mask = 0xFFFFFFFF); 00028 00029 /** Write the value to the output port 00030 * 00031 * @param value An integer specifying a bit to write for every corresponding port pin 00032 */ 00033 void write(int value); 00034 00035 /** Read the value currently output on the port 00036 * 00037 * @returns 00038 * An integer with each bit corresponding to associated port pin setting 00039 */ 00040 int read(); 00041 00042 /** Set as an output 00043 */ 00044 void output(); 00045 00046 /** Set as an input 00047 */ 00048 void input(); 00049 00050 /** Set the input pin mode 00051 * 00052 * @param mode PullUp, PullDown, PullNone, OpenDrain 00053 */ 00054 void mode(PinMode mode); 00055 00056 /** A shorthand for write() 00057 */ 00058 PortInOut& operator= (int value) { 00059 write(value); 00060 return *this; 00061 } 00062 00063 PortInOut& operator= (PortInOut& rhs) { 00064 write(rhs.read()); 00065 return *this; 00066 } 00067 00068 /** A shorthand for read() 00069 */ 00070 operator int() { 00071 return read(); 00072 } 00073 00074 private: 00075 #if defined(TARGET_LPC1768) || defined(TARGET_LPC2368) 00076 LPC_GPIO_TypeDef *_gpio; 00077 #endif 00078 PortName _port; 00079 uint32_t _mask; 00080 }; 00081 00082 } // namespace mbed 00083 00084 #endif 00085 00086 #endif
Generated on Tue Jul 12 2022 11:27:27 by
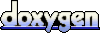