attempt to fix posible power issues with the sharp
Dependencies: ADS1115 BME280 CronoDot SDFileSystem mbed
Fork of Outdoor_UPAS_v1_2_Tboard by
main.cpp
00001 #include "mbed.h" 00002 #include "SDFileSystem.h" 00003 #include "Adafruit_ADS1015.h" 00004 #include "BME280.h" 00005 #include "CronoDot.h" 00006 00007 00008 //Edit for fork 00009 ///////////////////////////////////////////// 00010 //General Items 00011 ///////////////////////////////////////////// 00012 I2C i2c(PB_9, PB_8);//(D14, D15); SDA,SCL 00013 Serial pc(USBTX, USBRX); 00014 BME280 bmesensor(PB_9, PB_8);//(D14, D15); 00015 CronoDot RTC_UPAS(PB_9, PB_8);//(D14, D15); 00016 00017 00018 ///////////////////////////////////////////// 00019 //Analog to Digital Converter 00020 ///////////////////////////////////////////// 00021 Adafruit_ADS1115 ads(&i2c); 00022 Adafruit_ADS1115 ads2(&i2c); 00023 //DigitalIn ADS_ALRT(PA_10); //Connected but currently unused. (ADS1115) http://www.ti.com/lit/ds/symlink/ads1115.pdf 00024 00025 00026 00027 ///////////////////////////////////////////// 00028 //SD Card 00029 ///////////////////////////////////////////// 00030 char filename[] = "/sd/SHARP_LOG00.txt"; 00031 SDFileSystem sd(D11, D12, D13, D10, "sd"); // (MOSI, MISO, SCK, SEL) 00032 //DigitalIn sdCD(PA_11, PullUp); 00033 00034 00035 ///////////////////////////////////////////// 00036 //Callbacks 00037 ///////////////////////////////////////////// 00038 Ticker logg; //This is the logging callback object 00039 00040 00041 ///////////////////////////////////////////// 00042 //Varible Definitions 00043 ///////////////////////////////////////////// 00044 uint16_t serial_num = 1; // Default serial/calibration number 00045 int RunReady =0; 00046 00047 bool ledOn = 0; 00048 00049 struct tm STtime; 00050 char timestr[32]; 00051 char yrstr[4]; 00052 char mostr[4]; 00053 char daystr[4]; 00054 char hrstr[4]; 00055 char minstr[4]; 00056 char secstr[4]; 00057 00058 int stYr, stMo, stDay, stHr, stMin, stSec; 00059 00060 00061 float press; 00062 float temp; 00063 float rh; 00064 float atmoRho; //g/L 00065 int logInerval = 2;//seconds 00066 00067 DigitalOut sharp1LED(D7, 1); 00068 DigitalOut sharp2LED(D6, 1); 00069 DigitalOut sharp3LED(D5, 1); 00070 DigitalOut sharp4LED(D4, 1); 00071 DigitalOut sharp5LED(D3, 1); 00072 DigitalOut sharp6LED(D2, 1); 00073 00074 int samplingTime = 280; 00075 int deltaTime = 40; 00076 int sleepTime= 9680; 00077 int sharp1, sharp2, sharp3, sharp4, sharp5, sharp6; 00078 float sharpVolt1, sharpVolt2, sharpVolt3, sharpVolt4, sharpVolt5, sharpVolt6; //V 00079 00080 00081 00082 00083 ////////////////////////////////////////////////////////////// 00084 //SD Logging Function 00085 ////////////////////////////////////////////////////////////// 00086 void log_data() 00087 { 00088 //Get RTC time(s) 00089 /////////////////////////// 00090 RTC_UPAS.get_time(); 00091 time_t seconds = time(NULL); 00092 strftime(timestr, 32, "%y%m%d%H%M%S", localtime(&seconds)); 00093 /* 00094 strftime(yrstr, 4, "%y", localtime(&seconds)); 00095 stYr = atoi(yrstr); 00096 00097 strftime(mostr, 4, "%m", localtime(&seconds)); 00098 stMo = atoi(mostr); 00099 00100 strftime(daystr, 4, "%d", localtime(&seconds)); 00101 stDay = atoi(daystr); 00102 00103 strftime(hrstr, 4, "%H", localtime(&seconds)); 00104 stHr = atoi(hrstr); 00105 00106 strftime(minstr, 4, "%M", localtime(&seconds)); 00107 stMin = atoi(minstr); 00108 00109 strftime(secstr, 4, "%S", localtime(&seconds)); 00110 stSec = atoi(secstr); 00111 */ 00112 //pc.printf("%s,%s,%d,%s,%d,%s,%d,%s,%d,%s,%d,%s,%d\r\n", timestr,yrstr,stYr,mostr,stMo,daystr,stDay,hrstr,stHr,minstr,stMin,secstr,stSec); 00113 00114 00115 //Get Sensor Data except GPS 00116 //////////////////////////// 00117 press = bmesensor.getPressure(); 00118 temp = bmesensor.getTemperature(); 00119 rh = bmesensor.getHumidity(); 00120 atmoRho = ((press-((6.1078*pow((float)10,(float)((7.5*temp)/(237.3+temp))))*(rh/100)))*100)/(287.0531*(temp+273.15))+((6.1078*pow((float)10,(float)((7.5*temp)/(237.3+temp))))*(rh/100)*100)/(461.4964*(temp+273.15)); 00121 00122 //pc.printf("%f,%f,%f,%f\r\n", temp, press, rh, atmoRho); 00123 00124 sharp1LED = 0; 00125 wait_us(samplingTime); 00126 sharp1 = ads.readADC_SingleEnded(0, 0xC383); // read channel 1 00127 sharpVolt1 = (sharp1*4.096)/(32768*1); 00128 wait_us(deltaTime); 00129 sharp1LED = 1; 00130 wait_us(sleepTime); 00131 00132 sharp2LED = 0; 00133 wait_us(samplingTime); 00134 sharp2 = ads.readADC_SingleEnded(1, 0xD383); // read channel 1 00135 sharpVolt2 = (sharp2*4.096)/(32768*1); 00136 wait_us(deltaTime); 00137 sharp2LED = 1; 00138 wait_us(sleepTime); 00139 00140 sharp3LED = 0; 00141 wait_us(samplingTime); 00142 sharp3 = ads.readADC_SingleEnded(2, 0xE383); // read channel 1 00143 sharpVolt3 = (sharp3*4.096)/(32768*1); 00144 wait_us(deltaTime); 00145 sharp3LED = 1; 00146 wait_us(sleepTime); 00147 00148 sharp4LED = 0; 00149 wait_us(samplingTime); 00150 sharp4 = ads.readADC_SingleEnded(3, 0xF383); // read channel 1 00151 sharpVolt4 = (sharp4*4.096)/(32768*1); 00152 wait_us(deltaTime); 00153 sharp4LED = 1; 00154 wait_us(sleepTime); 00155 00156 sharp5LED = 0; 00157 wait_us(samplingTime); 00158 sharp5 = ads2.readADC_SingleEnded(0, 0xC383); // read channel 1 00159 sharpVolt5 = (sharp1*4.096)/(32768*1); 00160 sharp5LED = 1; 00161 wait_us(sleepTime); 00162 00163 sharp6LED = 0; 00164 wait_us(samplingTime); 00165 sharp6 = ads2.readADC_SingleEnded(0, 0xD383); // read channel 1 00166 sharpVolt6 = (sharp1*4.096)/(32768*1); 00167 sharp6LED = 1; 00168 wait_us(sleepTime); 00169 00170 00171 00172 00173 00174 00175 00176 //pc.printf("%d,%f,%d,%f,\r\n" ,sharp5,sharpVolt5, sharp6, sharpVolt6); 00177 pc.printf("%d,%d\r\n",sharp1, sharp4); 00178 //pc.printf("%d,%f,%d,%f,%d,%f,%d,%f\r\n" ,sharp1,sharpVolt1, sharp2, sharpVolt2,sharp3,sharpVolt3, sharp4, sharpVolt4); 00179 //pc.printf("%2.2f,%4.2f,%2.1f,%1.3f\r\n", temp,press,rh,atmoRho); 00180 //pc.printf("%d,%f,%d,%f\r\n" ,sharp3,sharpVolt3, sharp4, sharpVolt4); 00181 00182 FILE *fp = fopen(filename, "scotttrial"); 00183 fprintf(fp, "%02d,%02d,%02d,%02d,%02d,%02d,",RTC_UPAS.year, RTC_UPAS.month,RTC_UPAS.date,RTC_UPAS.hour,RTC_UPAS.minutes,RTC_UPAS.seconds); 00184 fprintf(fp, "%s,", timestr); 00185 fprintf(fp, "%2.2f,%4.2f,%2.1f,%1.3f", temp,press,rh,atmoRho); 00186 fprintf(fp, "%d,%f,%d,%f," ,sharp1,sharpVolt1, sharp2, sharpVolt2); 00187 fprintf(fp, "%d,%f,%d,%f" ,sharp3,sharpVolt3, sharp4, sharpVolt4); 00188 fprintf(fp, "%d,%f,%d,%f,\r\n" ,sharp5,sharpVolt5, sharp6, sharpVolt6); 00189 fclose(fp); 00190 free(fp); 00191 00192 00193 00194 00195 00196 00197 } 00198 00199 00200 ////////////////////////////////////////////////////////////// 00201 //Main Function 00202 ////////////////////////////////////////////////////////////// 00203 int main(){ 00204 00205 00206 00207 pc.baud(115200); // set what you want here depending on your terminal program speed 00208 pc.printf("\f\n\r-------------Startup-------------\n\r"); 00209 wait(0.5); 00210 00211 00212 RTC_UPAS.set_time(0,0,0,1,1,1,16);//sets chronodot RTC 00213 00214 /////////////////////// 00215 //sets ST RTC 00216 ////////////////////// 00217 STtime.tm_sec = 0; // 0-59 00218 STtime.tm_min = 0; // 0-59 00219 STtime.tm_hour = 0; // 0-23 00220 STtime.tm_mday = 1; // 1-31 00221 STtime.tm_mon = 0; // 0-11 00222 STtime.tm_year = 116; // year since 1900 (116 = 2016) 00223 time_t STseconds = mktime(&STtime); 00224 set_time(STseconds); // Set RTC time 00225 00226 00227 RTC_UPAS.get_time(); 00228 00229 00230 time_t seconds = time(NULL); 00231 strftime(timestr, 32, "%y-%m-%d-%H=%M=%S", localtime(&seconds)); 00232 00233 strftime(yrstr, 4, "%y", localtime(&seconds)); 00234 stYr = atoi(yrstr); 00235 00236 strftime(mostr, 4, "%m", localtime(&seconds)); 00237 stMo = atoi(mostr); 00238 00239 strftime(daystr, 4, "%d", localtime(&seconds)); 00240 stDay = atoi(daystr); 00241 00242 strftime(hrstr, 4, "%H", localtime(&seconds)); 00243 stHr = atoi(hrstr); 00244 00245 strftime(minstr, 4, "%M", localtime(&seconds)); 00246 stMin = atoi(minstr); 00247 00248 strftime(secstr, 4, "%S", localtime(&seconds)); 00249 stSec = atoi(secstr); 00250 00251 00252 00253 00254 // sprintf(filename,"/sd/SHARP_LOG00.txt"); 00255 00256 for (uint8_t i = 0; i < 100; i++) { 00257 filename[13] = i/10 + '0'; 00258 filename[14] = i%10 + '0'; 00259 FILE *fp = fopen(filename, "r"); 00260 if (fp == NULL) { 00261 // only open a new file if it doesn't exist 00262 FILE *fp = fopen(filename, "w"); 00263 fclose(fp); 00264 break; // leave the loop! 00265 } 00266 } 00267 00268 00269 00270 while(fmod((double)stSec,10)!=0) { 00271 //pc.printf("%f, %f\r\n", floor(secondsD), floor(lastsecondD)); 00272 time_t seconds = time(NULL); 00273 strftime(secstr, 4, "%S", localtime(&seconds)); 00274 stSec = atoi(secstr); 00275 wait_ms(100); 00276 } 00277 00278 logg.attach(&log_data, 3); 00279 00280 00281 00282 00283 //** end of initalization **// 00284 //---------------------------------------------------------------------------------------------// 00285 //---------------------------------------------------------------------------------------------// 00286 // Main Control Loop 00287 00288 while (1) { 00289 // Do other things... 00290 00291 } 00292 00293 00294 00295 00296 }
Generated on Sat Jul 16 2022 18:08:20 by
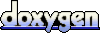