
Blank parameters for midterm
Dependencies: MMA8451Q8b SLCD mbed
KL46z_single_tap_2017.cpp
00001 #include "mbed.h" 00002 #include "MMA8451Q8g.h" 00003 #include "SLCD.h" 00004 00005 #define BLINKTIME 1.5 00006 #define RELAYON 0 00007 #define RELAYOFF 1 00008 #define LEDDELAY 0.4 00009 #define WAITDELAY 0.7 00010 #define LCDLEN 10 00011 00012 #define REG_WHO_AM_I 0x0D 00013 #define XYZ_DATA_CFG 0x0E 00014 00015 #define REG_OUT_X_MSB 0x01 00016 #define REG_OUT_Y_MSB 0x03 00017 #define REG_OUT_Z_MSB 0x05 00018 #define REG_PULSE_CFG 0x21 00019 #define REG_PULSE_SRC 0x22 00020 #define REG_PULSE_THSZ 0x25 00021 #define REH_PULSE_TMLT 0x26 00022 #define REG_CTRL_4 0x2D 00023 #define REG_CTRL_5 0x2E 00024 00025 #define MAX_2G 0x00 00026 #define MAX_4G 0x01 00027 #define MAX_8G 0x02 00028 /************************************** 00029 You need to fill nn the missing numbers below for this to work 00030 0x00 is just a place holder 00031 *************************************/ 00032 #define LATCH_DATA 0x00 // for use in REG_PULSE_CFG 00033 #define AXIS_DATA 0x00 00034 #define SET_INTERRUPT 0x00 //using interrupt INT1 PTC5 00035 #define SET_INT_LINE 0x00 00036 00037 #define SET_THZ 0x00 // See Table 49 in data sheet 00038 00039 #define SET_TMLT 0x00 // See Table 51 in data sheet 00040 /*********************************************************/ 00041 00042 //#define PRINTDBUG 00043 // Accelerometer I2C pins 00044 #if defined (TARGET_KL25Z) || defined (TARGET_KL46Z) 00045 PinName const SDA = PTE25; 00046 PinName const SCL = PTE24; 00047 #elif defined (TARGET_KL05Z) 00048 PinName const SDA = PTB4; 00049 PinName const SCL = PTB3; 00050 #else 00051 #error TARGET NOT DEFINED 00052 #endif 00053 00054 #define MMA8451_I2C_ADDRESS (0x1d<<1) 00055 00056 Ticker ledBlink; // timinginterrupt for RED led 00057 InterruptIn MMA8451QInt1(PTC5); //push botton with internal pullup 00058 //InterruptIn MMA8451QInt1(PTD1); 00059 DigitalOut myled(LED_RED); // red led 00060 DigitalOut relay(LED_GREEN); // green led 00061 00062 MMA8451Q acc(SDA, SCL, MMA8451_I2C_ADDRESS); 00063 00064 float delay = WAITDELAY; 00065 int relayState = RELAYOFF; 00066 int outState = false; 00067 SLCD slcd; //define LCD display 00068 char LCDMessages[2][LCDLEN] = {"TRUE", "FALS"}; 00069 00070 00071 void LCDMess(char *lMess, float dWait){ 00072 slcd.Home(); 00073 slcd.clear(); 00074 slcd.printf(lMess); 00075 wait(dWait); 00076 } 00077 void LCDMessNoDwell(char *lMess){ 00078 slcd.Home(); 00079 slcd.clear(); 00080 slcd.printf(lMess); 00081 } 00082 00083 // Interrupt routines 00084 void LEDBlinker(){ // RED LED interrupt 00085 outState = !outState; 00086 myled.write(outState); 00087 } 00088 00089 void GreenLEDBlinker(){ // Green LED interrupt 00090 //uint8_t i_regData; 00091 relayState = !relayState; 00092 relay.write(relayState); 00093 //acc.readRegs(REG_PULSE_SRC, &i_regData, 1); // Clear the tap event 00094 } 00095 00096 // end interrupt routines 00097 00098 int main() 00099 { 00100 uint8_t regData; 00101 00102 00103 char lcdData[LCDLEN]; 00104 00105 myled.write(outState); 00106 relay.write(relayState); 00107 00108 // set up interrrupts to be used later for taps 00109 MMA8451QInt1.rise(&GreenLEDBlinker); 00110 MMA8451QInt1.mode(PullNone); 00111 00112 // set up interrrupts to be used later for taps 00113 ledBlink.attach(&LEDBlinker, LEDDELAY); 00114 00115 // Read Pulse Source Data and check to see if things have been set 00116 acc.readRegs(REG_PULSE_CFG, ®Data, 1); // check it 00117 sprintf (lcdData,"%x",regData); 00118 LCDMess(lcdData,BLINKTIME); 00119 00120 // *********** Initialize for tap tecognition *********** 00121 regData = LATCH_DATA | AXIS_DATA; 00122 acc.setRegisterInStandby(REG_PULSE_CFG, regData); // write the data 00123 acc.readRegs(REG_PULSE_CFG, ®Data, 1); // check it 00124 sprintf (lcdData,"%x",regData); 00125 LCDMess(lcdData,BLINKTIME); 00126 00127 // Check to see if accerlometer is alive and well 00128 acc.setGLimit(MAX_4G); // For now set to 2g 00129 acc.readRegs(XYZ_DATA_CFG, ®Data, 1); 00130 sprintf (lcdData,"%x",regData); // Note displaying in hexidecimal 00131 LCDMess(lcdData,BLINKTIME); 00132 00133 // Setup single-tap pulse prarameters. 00134 acc.setRegisterInStandby(REG_PULSE_THSZ, SET_THZ); // write the data 00135 acc.setRegisterInStandby(REH_PULSE_TMLT, SET_TMLT); // write the data 00136 00137 // Set up (pulse) interrupt to INT1 pin 00138 acc.setRegisterInStandby(REG_CTRL_4, SET_INTERRUPT); // write the data 00139 acc.setRegisterInStandby(REG_CTRL_5, SET_INT_LINE); // write the data 00140 // End or seetup 00141 00142 acc.readRegs(REG_WHO_AM_I, ®Data, 1); 00143 sprintf (lcdData,"%x",regData); 00144 LCDMess(lcdData,BLINKTIME); 00145 00146 00147 while (true) { 00148 acc.readRegs(REG_PULSE_SRC, ®Data, 1); 00149 sprintf (lcdData,"%x",regData); 00150 LCDMess(lcdData,BLINKTIME); 00151 LCDMessNoDwell(LCDMessages[relayState]); 00152 wait(delay); 00153 } 00154 }
Generated on Mon Jul 25 2022 09:10:11 by
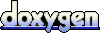