
three axis accelerometer shown as degrees for arccosine
Dependencies: MMA8451Q SLCD_degrees mbed
Fork of ACC_LCD_341_trig by
acc_angles.cpp
00001 #include "mbed.h" 00002 #include <math.h> 00003 #include "MMA8451Q.h" 00004 #include "SLCD.h" 00005 00006 #define NUMAXES 3 00007 #define XAXIS 0 00008 #define YAXIS 1 00009 #define ZAXIS 2 00010 #define NUMBUTS 2 00011 #define LBUT PTC12 // port addresses for buttons 00012 #define RBUT PTC3 00013 #define BUTTONTIME 0.200 00014 #define DATAINTERVAL 0.200 00015 #define LCDWAIT 1.5 00016 #define LCDDATALEN 10 00017 #define PI 3.14159265 00018 #define PITODEG 180.0 00019 00020 #define PROGNAME "ACC_LCD_all_axes_v1\r\n" 00021 00022 #define PRINTDBUG 00023 // 00024 #if defined (TARGET_KL25Z) || defined (TARGET_KL46Z) 00025 PinName const SDA = PTE25; // Data pins for the accelerometer/magnetometer. 00026 PinName const SCL = PTE24; // DO NOT CHANGE 00027 #elif defined (TARGET_KL05Z) 00028 PinName const SDA = PTB4; 00029 PinName const SCL = PTB3; 00030 #else 00031 #error TARGET NOT DEFINED 00032 #endif 00033 00034 #define MMA8451_I2C_ADDRESS (0x1d<<1) 00035 00036 SLCD slcd; //define LCD display 00037 char lcdData[LCDDATALEN]; //buffer needs places dor decimal pt and colon 00038 int currentAxis = XAXIS; // xaxis 00039 00040 MMA8451Q acc(SDA, SCL, MMA8451_I2C_ADDRESS); 00041 Serial pc(USBTX, USBRX); 00042 Timer dataTimer; 00043 Timer ButtonTimer; // for reading button states 00044 DigitalIn buttons[NUMBUTS] = {RBUT, LBUT}; // set up buttons 00045 00046 char axisName[NUMAXES][LCDDATALEN] = {"<X<","<Y<", "<Z<"}; 00047 00048 void LCDMess(char *lMess){ 00049 slcd.Home(); 00050 slcd.clear(); 00051 slcd.printf(lMess); 00052 } 00053 00054 void LCDsignedFloat(float theNumber){ 00055 sprintf (lcdData," %3.2f",theNumber); 00056 // changed SLCD.cpp to interpret < as - 00057 if (theNumber < 0.0) sprintf (lcdData,"<%3.2f",fabs(theNumber)); 00058 LCDMess(lcdData); 00059 } 00060 void LCDsignedAngle(float theAngle){ 00061 sprintf (lcdData," %2.0f@",theAngle); 00062 // changed SLCD.cpp to interpret < as - 00063 if (theAngle < 0.0) sprintf (lcdData,"<%2.0f@",fabs(theAngle)); 00064 LCDMess(lcdData); 00065 } 00066 00067 void initialize_global_vars(){ 00068 pc.printf(PROGNAME); 00069 // set up DAQ timers 00070 ButtonTimer.start(); 00071 ButtonTimer.reset(); 00072 dataTimer.start(); 00073 dataTimer.reset(); 00074 LCDMess(axisName[currentAxis]); 00075 wait(LCDWAIT); 00076 00077 } 00078 00079 int main() { 00080 int i; // loop index 00081 float axisValue[NUMAXES]; 00082 float angleValue[NUMAXES]; 00083 int buttonSum = 0; 00084 00085 initialize_global_vars(); 00086 // main loop forever 00087 while(true) { 00088 while (ButtonTimer > BUTTONTIME){ 00089 buttonSum = 0; 00090 buttonSum = !buttons[0] + !buttons[1]; 00091 if (buttonSum != 0) { 00092 currentAxis = (currentAxis + 1 ) % NUMAXES; 00093 LCDMess(axisName[currentAxis]); 00094 wait(LCDWAIT); 00095 }// for loop to look at buttons 00096 ButtonTimer.reset(); 00097 } 00098 while (dataTimer.read() > DATAINTERVAL){ 00099 dataTimer.reset(); 00100 axisValue[XAXIS]= acc.getAccX(); 00101 axisValue[YAXIS] = acc.getAccY(); 00102 axisValue[ZAXIS] = acc.getAccZ(); 00103 #ifdef PRINTDBUG 00104 for (i=0; i< NUMAXES;i++){ 00105 pc.printf("Acc %d = %f\r\n",i, axisValue[i]); 00106 } 00107 #endif 00108 if(fabs(axisValue[currentAxis]) > 1.0) axisValue[currentAxis] = 1.0; 00109 angleValue[currentAxis] = acos(axisValue[currentAxis]) * PITODEG / PI; 00110 LCDsignedAngle(angleValue[currentAxis]); 00111 //LCDsignedFloat(axisValue[currentAxis]); 00112 } 00113 } 00114 }
Generated on Thu Jul 21 2022 19:18:34 by
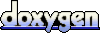