
smoothie port to mbed online compiler (smoothieware.org)
Embed:
(wiki syntax)
Show/hide line numbers
RingBuffer.h
00001 /* 00002 This file is part of Smoothie (http://smoothieware.org/). The motion control part is heavily based on Grbl (https://github.com/simen/grbl). 00003 Smoothie is free software: you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation, either version 3 of the License, or (at your option) any later version. 00004 Smoothie is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details. 00005 You should have received a copy of the GNU General Public License along with Smoothie. If not, see <http://www.gnu.org/licenses/>. 00006 00007 With chucks taken from http://en.wikipedia.org/wiki/Circular_buffer, see licence there also 00008 */ 00009 00010 #ifndef RINGBUFFER_H 00011 #define RINGBUFFER_H 00012 00013 00014 template<class kind, int length> class RingBuffer { 00015 public: 00016 RingBuffer(); 00017 int size(); 00018 int capacity(); 00019 int next_block_index(int index); 00020 int prev_block_index(int index); 00021 void push_back(kind object); 00022 void pop_front(kind &object); 00023 void get( int index, kind &object); 00024 kind* get_ref( int index); 00025 void delete_first(); 00026 00027 kind buffer[length]; 00028 int head; 00029 int tail; 00030 }; 00031 00032 00033 template<class kind, int length> RingBuffer<kind, length>::RingBuffer(){ 00034 this->head = this->tail = 0; 00035 } 00036 00037 template<class kind, int length> int RingBuffer<kind, length>::capacity(){ 00038 return length-1; 00039 } 00040 00041 template<class kind, int length> int RingBuffer<kind, length>::size(){ 00042 return((this->head>this->tail)?length:0)+this->tail-head; 00043 } 00044 00045 template<class kind, int length> int RingBuffer<kind, length>::next_block_index(int index){ 00046 index++; 00047 if (index == length) { index = 0; } 00048 return(index); 00049 } 00050 00051 template<class kind, int length> int RingBuffer<kind, length>::prev_block_index(int index){ 00052 if (index == 0) { index = length; } 00053 index--; 00054 return(index); 00055 } 00056 00057 template<class kind, int length> void RingBuffer<kind, length>::push_back(kind object){ 00058 this->buffer[this->tail] = object; 00059 this->tail = (tail+1)&(length-1); 00060 } 00061 00062 template<class kind, int length> void RingBuffer<kind, length>::get(int index, kind &object){ 00063 int j= 0; 00064 int k= this->head; 00065 while (k != this->tail){ 00066 if (j == index) break; 00067 j++; 00068 k= (k + 1) & (length - 1); 00069 } 00070 if (k == this->tail){ 00071 //return NULL; 00072 } 00073 object = this->buffer[k]; 00074 } 00075 00076 00077 template<class kind, int length> kind* RingBuffer<kind, length>::get_ref(int index){ 00078 int j= 0; 00079 int k= this->head; 00080 while (k != this->tail){ 00081 if (j == index) break; 00082 j++; 00083 k= (k + 1) & (length - 1); 00084 } 00085 if (k == this->tail){ 00086 return NULL; 00087 } 00088 return &(this->buffer[k]); 00089 } 00090 00091 template<class kind, int length> void RingBuffer<kind, length>::pop_front(kind &object){ 00092 object = this->buffer[this->head]; 00093 this->head = (this->head+1)&(length-1); 00094 } 00095 00096 template<class kind, int length> void RingBuffer<kind, length>::delete_first(){ 00097 //kind dummy; 00098 //this->pop_front(dummy); 00099 this->head = (this->head+1)&(length-1); 00100 } 00101 00102 00103 #endif
Generated on Tue Jul 12 2022 14:14:41 by
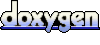