
smoothie port to mbed online compiler (smoothieware.org)
Embed:
(wiki syntax)
Show/hide line numbers
Gcode.cpp
00001 /* 00002 This file is part of Smoothie (http://smoothieware.org/). The motion control part is heavily based on Grbl (https://github.com/simen/grbl). 00003 Smoothie is free software: you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation, either version 3 of the License, or (at your option) any later version. 00004 Smoothie is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details. 00005 You should have received a copy of the GNU General Public License along with Smoothie. If not, see <http://www.gnu.org/licenses/>. 00006 */ 00007 00008 00009 #include <string> 00010 using std::string; 00011 #include "Gcode.h" 00012 #include "libs/StreamOutput.h" 00013 00014 #include <stdlib.h> 00015 00016 00017 Gcode::Gcode(){} 00018 00019 // Whether or not a Gcode has a letter 00020 bool Gcode::has_letter( char letter ){ 00021 //return ( this->command->find( letter ) != string::npos ); 00022 for (size_t i=0; i < this->command.length(); i++){ 00023 if( this->command.at(i) == letter ){ 00024 return true; 00025 } 00026 } 00027 return false; 00028 } 00029 00030 // Retrieve the value for a given letter 00031 // We don't use the high-level methods of std::string because they call malloc and it's very bad to do that inside of interrupts 00032 double Gcode::get_value( char letter ){ 00033 //__disable_irq(); 00034 for (size_t i=0; i <= this->command.length()-1; i++){ 00035 if( letter == this->command.at(i) ){ 00036 size_t beginning = i+1; 00037 char buffer[20]; 00038 for(size_t j=beginning; j <= this->command.length(); j++){ 00039 char c; 00040 if( j == this->command.length() ){ c = ';'; }else{ c = this->command.at(j); } 00041 if( c != '.' && c != '-' && ( c < '0' || c > '9' ) ){ 00042 buffer[j-beginning] = '\0'; 00043 //__enable_irq(); 00044 return atof(buffer); 00045 }else{ 00046 buffer[j-beginning] = c; 00047 } 00048 } 00049 } 00050 } 00051 //__enable_irq(); 00052 return 0; 00053 }
Generated on Tue Jul 12 2022 14:14:40 by
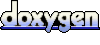