
smoothie port to mbed online compiler (smoothieware.org)
Embed:
(wiki syntax)
Show/hide line numbers
Config.cpp
00001 /* 00002 This file is part of Smoothie (http://smoothieware.org/). The motion control part is heavily based on Grbl (https://github.com/simen/grbl). 00003 Smoothie is free software: you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation, either version 3 of the License, or (at your option) any later version. 00004 Smoothie is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details. 00005 You should have received a copy of the GNU General Public License along with Smoothie. If not, see <http://www.gnu.org/licenses/>. 00006 */ 00007 00008 00009 using namespace std; 00010 #include <vector> 00011 #include <string> 00012 00013 #include "libs/Kernel.h" 00014 #include "Config.h" 00015 #include "ConfigValue.h" 00016 #include "ConfigSource.h" 00017 #include "ConfigCache.h" 00018 #include "libs/nuts_bolts.h" 00019 #include "libs/utils.h" 00020 #include "libs/SerialMessage.h" 00021 #include "libs/ConfigSources/FileConfigSource.h" 00022 00023 00024 Config::Config(){ 00025 00026 this->config_cache_loaded = false; 00027 00028 // Config source for */config files 00029 this->config_sources.push_back( new FileConfigSource("/local/config", LOCAL_CONFIGSOURCE_CHECKSUM) ); 00030 //this->config_sources.push_back( new FileConfigSource("/sd/config", SD_CONFIGSOURCE_CHECKSUM ) ); 00031 00032 // Pre-load the config cache 00033 this->config_cache_load(); 00034 00035 } 00036 00037 void Config::on_module_loaded(){} 00038 00039 void Config::on_console_line_received( void* argument ){} 00040 00041 void Config::set_string( string setting, string value ){ 00042 ConfigValue* cv = new ConfigValue; 00043 cv->found = true; 00044 cv->check_sums = get_checksums(setting); 00045 cv->value = value; 00046 00047 this->config_cache.replace_or_push_back(cv); 00048 00049 this->kernel->call_event(ON_CONFIG_RELOAD); 00050 } 00051 00052 void Config::get_module_list(vector<uint16_t>* list, uint16_t family){ 00053 for( int i=1; i<this->config_cache.size(); i++){ 00054 ConfigValue* value = this->config_cache.at(i); 00055 if( value->check_sums.size() == 3 && value->check_sums.at(2) == 29545 && value->check_sums.at(0) == family ){ 00056 // We found a module enable for this family, add it's number 00057 list->push_back(value->check_sums.at(1)); 00058 } 00059 } 00060 } 00061 00062 00063 // Command to load config cache into buffer for multiple reads during init 00064 void Config::config_cache_load(){ 00065 00066 this->config_cache_clear(); 00067 00068 // First element is a special empty ConfigValue for values not found 00069 ConfigValue* result = new ConfigValue; 00070 this->config_cache.push_back(result); 00071 // For each ConfigSource in our stack 00072 for( unsigned int i = 0; i < this->config_sources.size(); i++ ){ 00073 ConfigSource* source = this->config_sources[i]; 00074 source->transfer_values_to_cache(&this->config_cache); 00075 } 00076 00077 this->config_cache_loaded = true; 00078 } 00079 00080 // Command to clear the config cache after init 00081 void Config::config_cache_clear(){ 00082 while( ! this->config_cache.empty() ){ 00083 delete this->config_cache.back(); 00084 this->config_cache.pop_back(); 00085 } 00086 this->config_cache_loaded = false; 00087 } 00088 00089 00090 ConfigValue* Config::value(uint16_t check_sum_a, uint16_t check_sum_b, uint16_t check_sum_c ){ 00091 vector<uint16_t> check_sums; 00092 check_sums.push_back(check_sum_a); 00093 check_sums.push_back(check_sum_b); 00094 check_sums.push_back(check_sum_c); 00095 return this->value(check_sums); 00096 } 00097 00098 ConfigValue* Config::value(uint16_t check_sum_a, uint16_t check_sum_b){ 00099 vector<uint16_t> check_sums; 00100 check_sums.push_back(check_sum_a); 00101 check_sums.push_back(check_sum_b); 00102 return this->value(check_sums); 00103 } 00104 00105 ConfigValue* Config::value(uint16_t check_sum){ 00106 vector<uint16_t> check_sums; 00107 check_sums.push_back(check_sum); 00108 return this->value(check_sums); 00109 } 00110 00111 // Get a value from the configuration as a string 00112 // Because we don't like to waste space in Flash with lengthy config parameter names, we take a checksum instead so that the name does not have to be stored 00113 // See get_checksum 00114 ConfigValue* Config::value(vector<uint16_t> check_sums){ 00115 ConfigValue* result = this->config_cache[0]; 00116 //if( this->has_config_file() == false ){ 00117 // return result; 00118 //} 00119 // Check if the config is cached, and load it temporarily if it isn't 00120 bool cache_preloaded = this->config_cache_loaded; 00121 if( !cache_preloaded ){ this->config_cache_load(); } 00122 00123 for( int i=1; i<this->config_cache.size(); i++){ 00124 // If this line matches the checksum 00125 bool match = true; 00126 for( unsigned int j = 0; j < check_sums.size(); j++ ){ 00127 uint16_t checksum_node = check_sums[j]; 00128 00129 //printf("%u(%s) against %u\r\n", get_checksum(key_node), key_node.c_str(), checksum_node); 00130 if(this->config_cache[i]->check_sums[j] != checksum_node ){ 00131 match = false; 00132 break; 00133 } 00134 } 00135 if( match == false ){ 00136 //printf("continue\r\n"); 00137 continue; 00138 } 00139 result = this->config_cache[i]; 00140 break; 00141 } 00142 00143 if( !cache_preloaded ){ 00144 this->config_cache_clear(); 00145 } 00146 return result; 00147 } 00148 00149 00150
Generated on Tue Jul 12 2022 14:14:39 by
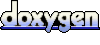