
smoothie port to mbed online compiler (smoothieware.org)
Embed:
(wiki syntax)
Show/hide line numbers
ConfigValue.h
00001 /* 00002 This file is part of Smoothie (http://smoothieware.org/). The motion control part is heavily based on Grbl (https://github.com/simen/grbl). 00003 Smoothie is free software: you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation, either version 3 of the License, or (at your option) any later version. 00004 Smoothie is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details. 00005 You should have received a copy of the GNU General Public License along with Smoothie. If not, see <http://www.gnu.org/licenses/>. 00006 */ 00007 00008 #ifndef CONFIGVALUE_H 00009 #define CONFIGVALUE_H 00010 00011 #include "libs/Kernel.h" 00012 #include "libs/utils.h" 00013 #include "libs/Pin.h" 00014 00015 00016 #define error(...) (fprintf(stderr, __VA_ARGS__), exit(1)) 00017 00018 using namespace std; 00019 #include <vector> 00020 #include <string> 00021 #include <stdio.h> 00022 00023 00024 class ConfigValue{ 00025 public: 00026 ConfigValue(){ 00027 this->found = false; 00028 this->default_set = false; 00029 }; 00030 00031 ConfigValue* required(){ 00032 if( this->found == true ){ 00033 return this; 00034 }else{ 00035 error("could not find config setting with checksum %u, please see http://smoothieware.org/configuring-smoothie\r\n", this->check_sum ); 00036 return NULL; 00037 } 00038 } 00039 00040 double as_number(){ 00041 if( this->found == false && this->default_set == true ){ 00042 return this->default_double; 00043 }else{ 00044 double result = atof(remove_non_number(this->value).c_str()); 00045 if( result == 0.0 && this->value.find_first_not_of("0.") != string::npos ){ 00046 error("config setting with value '%s' is not a valid number, please see http://smoothieware.org/configuring-smoothie\r\n", this->value.c_str() ); 00047 } 00048 return result; 00049 } 00050 00051 } 00052 00053 std::string as_string(){ 00054 if( this->found == false && this->default_set == true ){ 00055 return this->default_string; 00056 }else{ 00057 return this->value; 00058 } 00059 } 00060 00061 bool as_bool(){ 00062 if( this->found == false && this->default_set == true ){ 00063 return this->default_double; 00064 }else{ 00065 if( this->value.find_first_of("t1") != string::npos ){ 00066 return true; 00067 }else{ 00068 return false; 00069 } 00070 } 00071 } 00072 00073 Pin* as_pin(){ 00074 Pin* pin = new Pin(); 00075 pin->from_string(this->as_string()); 00076 return pin; 00077 } 00078 00079 ConfigValue* by_default(double val){ 00080 this->default_set = true; 00081 this->default_double = val; 00082 return this; 00083 } 00084 00085 ConfigValue* by_default(std::string val){ 00086 this->default_set = true; 00087 this->default_string = val; 00088 return this; 00089 } 00090 00091 bool has_characters( string mask ){ 00092 if( this->value.find_first_of(mask) != string::npos ){ return true; }else{ return false; } 00093 } 00094 00095 bool is_inverted(){ 00096 return this->has_characters(string("!")); 00097 } 00098 00099 string value; 00100 vector<uint16_t> check_sums; 00101 uint16_t check_sum; 00102 bool found; 00103 bool default_set; 00104 double default_double; 00105 string default_string; 00106 }; 00107 00108 00109 00110 00111 00112 00113 00114 00115 #endif
Generated on Tue Jul 12 2022 14:14:40 by
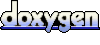