
A simple program to read an analoge channel on the Freedom Kl25Z using the ticker object to control timing. The data is written into a circular buffer, which is read by the main loop and sent down the serial line.
Dependencies: FastAnalogIn mbed
main.cpp
00001 #include "mbed.h" 00002 #include "FastAnalogIn.h" 00003 00004 /* 00005 Simple program which uses a ticker object to record and analoge value and store it in a cicular buffer. 00006 The ticker delay sets the sampling rate. 00007 00008 The FastAnalogIn Library is used (http://mbed.org/users/Sissors/code/FastAnalogIn/) to rapidly sample 00009 a single channel. 00010 00011 In the main loop a busy loop is used to send read the data from the cicular buffer and send down the serial line 00012 00013 00014 */ 00015 00016 //size of cicular buffer 00017 #define BuffSize 255 00018 00019 using namespace mbed; 00020 00021 FastAnalogIn Ain(PTC2); 00022 00023 Serial pc(USBTX, USBRX); 00024 Timer t; 00025 Ticker tock; 00026 00027 //set the delay used by the ticker 00028 float delay = 0.01; 00029 00030 //some circular buffers 00031 00032 float data[BuffSize]; 00033 float time_[BuffSize]; 00034 long i =0; 00035 int j=0; 00036 long k =0; 00037 00038 void ana() 00039 { 00040 if(i<k+BuffSize) 00041 {j = i%BuffSize; 00042 //time_[j]=t.read(); 00043 data[j]=Ain.read(); 00044 time_[j]=t.read(); 00045 i++; 00046 } 00047 } 00048 00049 00050 int main() 00051 { 00052 pc.baud(57600); 00053 t.start(); 00054 tock.attach(&ana,delay); 00055 while (true) { 00056 if(k<i) 00057 { 00058 pc.printf("%f,%f\n",time_[k%BuffSize],data[k%BuffSize]); 00059 k++; 00060 } 00061 00062 00063 } 00064 }
Generated on Fri Jul 15 2022 22:26:03 by
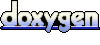