C027 Interface
Fork of C027Interface by
Embed:
(wiki syntax)
Show/hide line numbers
C027Interface.cpp
00001 /* C027 implementation of NetworkInterfaceAPI 00002 * Copyright (c) 2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "C027Interface.h" 00018 #include "mbed.h" 00019 00020 00021 // C027Interface implementation 00022 C027Interface::C027Interface(const char *simpin, bool debug) 00023 : _debug(debug) 00024 { 00025 strcpy(_pin, simpin); 00026 } 00027 00028 int C027Interface::connect(const char *apn, const char *username, const char *password) 00029 { 00030 // create the modem 00031 _mdm = new MDMSerial; 00032 if (_debug) { 00033 _mdm->setDebug(4); 00034 } else { 00035 _mdm->setDebug(0); 00036 } 00037 00038 // initialize the modem 00039 MDMParser::DevStatus devStatus = {}; 00040 MDMParser::NetStatus netStatus = {}; 00041 bool mdmOk = _mdm->init(_pin, &devStatus); 00042 if (_debug) { 00043 _mdm->dumpDevStatus(&devStatus); 00044 } 00045 00046 if (mdmOk) { 00047 // wait until we are connected 00048 mdmOk = _mdm->registerNet(&netStatus); 00049 if (_debug) { 00050 _mdm->dumpNetStatus(&netStatus); 00051 } 00052 } 00053 00054 if (mdmOk) { 00055 // join the internet connection 00056 MDMParser::IP ip = _mdm->join(apn, username, password); 00057 _ip_address.set_ip_bytes(&ip, NSAPI_IPv4); 00058 mdmOk = (ip != NOIP); 00059 } 00060 00061 return mdmOk ? 0 : NSAPI_ERROR_DEVICE_ERROR; 00062 } 00063 00064 int C027Interface::disconnect() 00065 { 00066 if (!_mdm->disconnect()) { 00067 return NSAPI_ERROR_DEVICE_ERROR; 00068 } 00069 00070 return 0; 00071 } 00072 00073 const char *C027Interface::get_ip_address() 00074 { 00075 return _ip_address.get_ip_address(); 00076 } 00077 00078 const char *C027Interface::get_mac_address() 00079 { 00080 return 0; 00081 } 00082 00083 struct c027_socket { 00084 int socket; 00085 MDMParser::IpProtocol proto; 00086 MDMParser::IP ip; 00087 int port; 00088 }; 00089 00090 int C027Interface::socket_open(void **handle, nsapi_protocol_t proto) 00091 { 00092 MDMParser::IpProtocol mdmproto = (proto == NSAPI_UDP) ? MDMParser::IPPROTO_UDP : MDMParser::IPPROTO_TCP; 00093 int fd = _mdm->socketSocket(mdmproto); 00094 if (fd < 0) { 00095 return NSAPI_ERROR_NO_SOCKET; 00096 } 00097 00098 _mdm->socketSetBlocking(fd, 10000); 00099 struct c027_socket *socket = new struct c027_socket; 00100 if (!socket) { 00101 return NSAPI_ERROR_NO_SOCKET; 00102 } 00103 00104 socket->socket = fd; 00105 socket->proto = mdmproto; 00106 *handle = socket; 00107 return 0; 00108 } 00109 00110 int C027Interface::socket_close(void *handle) 00111 { 00112 struct c027_socket *socket = (struct c027_socket *)handle; 00113 _mdm->socketFree(socket->socket); 00114 00115 delete socket; 00116 return 0; 00117 } 00118 00119 int C027Interface::socket_bind(void *handle, const SocketAddress &address) 00120 { 00121 return NSAPI_ERROR_UNSUPPORTED; 00122 } 00123 00124 int C027Interface::socket_listen(void *handle, int backlog) 00125 { 00126 return NSAPI_ERROR_UNSUPPORTED; 00127 } 00128 00129 int C027Interface::socket_connect(void *handle, const SocketAddress &addr) 00130 { 00131 struct c027_socket *socket = (struct c027_socket *)handle; 00132 00133 if (!_mdm->socketConnect(socket->socket, addr.get_ip_address(), addr.get_port())) { 00134 return NSAPI_ERROR_DEVICE_ERROR; 00135 } 00136 00137 return 0; 00138 } 00139 00140 int C027Interface::socket_accept(void **handle, void *server) 00141 { 00142 return NSAPI_ERROR_UNSUPPORTED; 00143 } 00144 00145 int C027Interface::socket_send(void *handle, const void *data, unsigned size) 00146 { 00147 struct c027_socket *socket = (struct c027_socket *)handle; 00148 00149 int sent = _mdm->socketSend(socket->socket, (const char *)data, size); 00150 if (sent == SOCKET_ERROR) { 00151 return NSAPI_ERROR_DEVICE_ERROR; 00152 } 00153 00154 return sent; 00155 } 00156 00157 int C027Interface::socket_recv(void *handle, void *data, unsigned size) 00158 { 00159 struct c027_socket *socket = (struct c027_socket *)handle; 00160 if (!_mdm->socketReadable(socket->socket)) { 00161 return NSAPI_ERROR_WOULD_BLOCK; 00162 } 00163 00164 int recv = _mdm->socketRecv(socket->socket, (char *)data, size); 00165 if (recv == SOCKET_ERROR) { 00166 return NSAPI_ERROR_DEVICE_ERROR; 00167 } 00168 00169 return recv; 00170 } 00171 00172 int C027Interface::socket_sendto(void *handle, const SocketAddress &addr, const void *data, unsigned size) 00173 { 00174 struct c027_socket *socket = (struct c027_socket *)handle; 00175 00176 int sent = _mdm->socketSendTo(socket->socket, 00177 *(MDMParser::IP *)addr.get_ip_bytes(), addr.get_port(), 00178 (const char *)data, size); 00179 00180 if (sent == SOCKET_ERROR) { 00181 return NSAPI_ERROR_DEVICE_ERROR; 00182 } 00183 00184 return sent; 00185 } 00186 00187 int C027Interface::socket_recvfrom(void *handle, SocketAddress *addr, void *data, unsigned size) 00188 { 00189 struct c027_socket *socket = (struct c027_socket *)handle; 00190 if (!_mdm->socketReadable(socket->socket)) { 00191 return NSAPI_ERROR_WOULD_BLOCK; 00192 } 00193 00194 MDMParser::IP ip; 00195 int port; 00196 00197 int recv = _mdm->socketRecvFrom(socket->socket, &ip, &port, (char *)data, size); 00198 if (recv == SOCKET_ERROR) { 00199 return NSAPI_ERROR_DEVICE_ERROR; 00200 } 00201 00202 if (addr) { 00203 addr->set_ip_bytes(&ip, NSAPI_IPv4); 00204 addr->set_port(port); 00205 } 00206 00207 return recv; 00208 } 00209 00210 void C027Interface::_run() 00211 { 00212 while (true) { 00213 _callback(_data); 00214 wait(0.25f); 00215 } 00216 } 00217 00218 void C027Interface::socket_attach(void *handle, void (*callback)(void *), void *data) 00219 { 00220 _callback = callback; 00221 _data = data; 00222 _thread.start(this, &C027Interface::_run); 00223 }
Generated on Tue Jul 12 2022 23:44:17 by
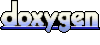