First Release
Embed:
(wiki syntax)
Show/hide line numbers
USBJoystick.cpp
00001 /* Copyright (c) 2010-2011 mbed.org, MIT License 00002 * Modified Mouse code for Joystick - WH 2012 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 * and associated documentation files (the "Software"), to deal in the Software without 00006 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00007 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00008 * Software is furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in all copies or 00011 * substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 00020 #include "stdint.h" 00021 #include "USBJoystick.h" 00022 00023 bool USBJoystick::update(int16_t x, int16_t y, int16_t z, int16_t rz, uint32_t buttons, uint8_t stick) 00024 { 00025 HID_REPORT report; 00026 int idx = 0; 00027 00028 _x = x; 00029 _y = y; 00030 _z = z; 00031 _rz = rz; 00032 _buttons = buttons; 00033 _stick = stick; 00034 00035 unsigned char hatswitch; 00036 if (_stick & JOYSTICK_UP) { 00037 hatswitch = 0; 00038 if (_stick & JOYSTICK_RIGHT) hatswitch = 1; 00039 if (_stick & JOYSTICK_LEFT) hatswitch = 7; 00040 } else if (_stick & JOYSTICK_RIGHT) { 00041 hatswitch = 2; 00042 if (_stick & JOYSTICK_DOWN) hatswitch = 3; 00043 } else if (_stick & JOYSTICK_DOWN) { 00044 hatswitch = 4; 00045 if (_stick & JOYSTICK_LEFT) hatswitch = 5; 00046 } else if (_stick & JOYSTICK_LEFT) { 00047 hatswitch = 6; 00048 } else { 00049 hatswitch = 0xf; 00050 } 00051 00052 // Fill the report according to the Joystick Descriptor 00053 report.data[idx++] = _buttons & 0xff; 00054 report.data[idx++] = (_buttons>>8) & 0xff; 00055 report.data[idx++] = hatswitch & 0x0f; 00056 report.data[idx++] = _x + 0x80; 00057 report.data[idx++] = _y + 0x80; 00058 report.data[idx++] = _z + 0x80; 00059 report.data[idx++] = _rz + 0x80; 00060 00061 report.data[idx++] = 0; 00062 report.data[idx++] = 0; 00063 report.data[idx++] = 0; 00064 report.data[idx++] = 0; 00065 report.data[idx++] = 0; 00066 report.data[idx++] = 0; 00067 report.data[idx++] = 0; 00068 report.data[idx++] = 0; 00069 report.data[idx++] = 0; 00070 report.data[idx++] = 0; 00071 report.data[idx++] = 0; 00072 report.data[idx++] = 0; 00073 report.length = idx; 00074 00075 return send(&report); 00076 } 00077 00078 bool USBJoystick::update() 00079 { 00080 HID_REPORT report; 00081 int idx = 0; 00082 00083 unsigned char hatswitch; 00084 if (_stick & JOYSTICK_UP) { 00085 hatswitch = 0; 00086 if (_stick & JOYSTICK_RIGHT) hatswitch = 1; 00087 if (_stick & JOYSTICK_LEFT) hatswitch = 7; 00088 } else if (_stick & JOYSTICK_RIGHT) { 00089 hatswitch = 2; 00090 if (_stick & JOYSTICK_DOWN) hatswitch = 3; 00091 } else if (_stick & JOYSTICK_DOWN) { 00092 hatswitch = 4; 00093 if (_stick & JOYSTICK_LEFT) hatswitch = 5; 00094 } else if (_stick & JOYSTICK_LEFT) { 00095 hatswitch = 6; 00096 } else { 00097 hatswitch = 0xf; 00098 } 00099 00100 // Fill the report according to the Joystick Descriptor 00101 report.data[idx++] = _buttons & 0xff; 00102 report.data[idx++] = (_buttons>>8) & 0xff; 00103 report.data[idx++] = hatswitch & 0x0f; 00104 report.data[idx++] = _x + 0x80; 00105 report.data[idx++] = _y + 0x80; 00106 report.data[idx++] = _z + 0x80; 00107 report.data[idx++] = _rz + 0x80; 00108 00109 report.data[idx++] = 0; 00110 report.data[idx++] = 0; 00111 report.data[idx++] = 0; 00112 report.data[idx++] = 0; 00113 report.data[idx++] = 0; 00114 report.data[idx++] = 0; 00115 report.data[idx++] = 0; 00116 report.data[idx++] = 0; 00117 report.data[idx++] = 0; 00118 report.data[idx++] = 0; 00119 report.data[idx++] = 0; 00120 report.data[idx++] = 0; 00121 report.length = idx; 00122 00123 return send(&report); 00124 } 00125 00126 00127 00128 void USBJoystick::_init() 00129 { 00130 _x = 0; 00131 _y = 0; 00132 _z = 0; 00133 _rz = 0; 00134 _buttons = 0; 00135 _stick = 0; 00136 } 00137 00138 00139 uint8_t * USBJoystick::reportDesc() 00140 { 00141 static uint8_t reportDescriptor[] = 00142 { 00143 0x05, 0x01, // USAGE_PAGE (Generic Desktop) 00144 0x09, 0x05, // USAGE (Gamepad) 00145 0xa1, 0x01, // COLLECTION (Application) 00146 00147 0x15, 0x00, // LOGICAL_MINIMUM (0) 00148 0x25, 0x01, // LOGICAL_MAXIMUM (1) 00149 0x35, 0x00, // PHYSICAL_MINIMUM (0) 00150 0x45, 0x01, // PHYSICAL_MAXIMUM (1) 00151 0x75, 0x01, // REPORT_SIZE (1) 00152 0x95, 0x0d, // REPORT_COUNT (13) 00153 0x05, 0x09, // USAGE_PAGE (Button) 00154 0x19, 0x01, // USAGE_MINIMUM (Button 1) 00155 0x29, 0x0d, // USAGE_MAXIMUM (Button 13) 00156 0x81, 0x02, // INPUT (Data,Var,Abs) 00157 0x95, 0x03, // REPORT_COUNT (3) 00158 0x81, 0x01, // INPUT (Cnst,Ary,Abs) 00159 0x05, 0x01, // USAGE_PAGE (Generic Desktop) 00160 0x25, 0x07, // LOGICAL_MAXIMUM (7) 00161 0x46, 0x3b, 0x01, // PHYSICAL_MAXIMUM (315) 00162 0x75, 0x04, // REPORT_SIZE (4) 00163 0x95, 0x01, // REPORT_COUNT (1) 00164 0x65, 0x14, // UNIT (Eng Rot:Angular Pos) 00165 0x09, 0x39, // USAGE (Hat switch) 00166 0x81, 0x42, // INPUT (Data,Var,Abs,Null) 00167 0x65, 0x00, // UNIT (None) 00168 0x95, 0x01, // REPORT_COUNT (1) 00169 0x81, 0x01, // INPUT (Cnst,Ary,Abs) 00170 00171 0x26, 0xff, 0x00, // LOGICAL_MAXIMUM (255) 00172 0x46, 0xff, 0x00, // PHYSICAL_MAXIMUM (255) 00173 0x09, 0x30, // USAGE (X) 00174 0x09, 0x31, // USAGE (Y) 00175 0x09, 0x32, // USAGE (Z) 00176 0x09, 0x35, // USAGE (Rz) 00177 0x75, 0x08, // REPORT_SIZE (8) 00178 0x95, 0x04, // REPORT_COUNT (4) 00179 0x81, 0x02, // INPUT (Data,Var,Abs) 00180 00181 0x06, 0x00, 0xff, // USAGE_PAGE (Vendor Specific) 00182 0x09, 0x20, // Unknown 00183 0x09, 0x21, // Unknown 00184 0x09, 0x22, // Unknown 00185 0x09, 0x23, // Unknown 00186 0x09, 0x24, // Unknown 00187 0x09, 0x25, // Unknown 00188 0x09, 0x26, // Unknown 00189 0x09, 0x27, // Unknown 00190 0x09, 0x28, // Unknown 00191 0x09, 0x29, // Unknown 00192 0x09, 0x2a, // Unknown 00193 0x09, 0x2b, // Unknown 00194 0x95, 0x0c, // REPORT_COUNT (12) 00195 0x81, 0x02, // INPUT (Data,Var,Abs) 00196 0x0a, 0x21, 0x26, // Unknown 00197 0x95, 0x08, // REPORT_COUNT (8) 00198 0xb1, 0x02, // FEATURE (Data,Var,Abs) 00199 00200 0xc0, // END_COLLECTION 00201 00202 /* 00203 USAGE_PAGE(1), 0x01, 00204 USAGE(1), 0x04, 00205 COLLECTION(1), 0x01, 00206 USAGE(1), 0x01, 00207 COLLECTION(1), 0x00, 00208 00209 USAGE_PAGE(1), 0x09, 00210 USAGE_MINIMUM(1), 0x01, 00211 USAGE_MAXIMUM(1), 0x0C, 00212 LOGICAL_MINIMUM(1), 0x00, 00213 LOGICAL_MAXIMUM(1), 0x01, 00214 PHYSICAL_MINIMUM(1), 0x00, 00215 PHYSICAL_MAXIMUM(1), 0x01, 00216 REPORT_SIZE(1), 0x01, 00217 REPORT_COUNT(1), 0x0C, 00218 INPUT(1), 0x02, 00219 00220 USAGE_PAGE(1), 0x01, 00221 USAGE(1), 0x39, 00222 LOGICAL_MINIMUM(1), 0x00, 00223 LOGICAL_MAXIMUM(1), 0x07, 00224 PHYSICAL_MINIMUM(1), 0x00, 00225 PHYSICAL_MAXIMUM(2), 0x3B, 0x01, 00226 UNIT(1), 0x14, 00227 REPORT_SIZE(1), 0x04, 00228 REPORT_COUNT(1), 0x01, 00229 INPUT(1), 0x42, 00230 00231 USAGE_PAGE(1), 0x01, 00232 USAGE(1), 0x30, 00233 USAGE(1), 0x31, 00234 USAGE(1), 0x32, 00235 USAGE(1), 0x35, 00236 LOGICAL_MINIMUM(1), 0x00, 00237 LOGICAL_MAXIMUM(2), 0xff, 0x00, 00238 PHYSICAL_MINIMUM(1), 0x00, 00239 PHYSICAL_MAXIMUM(2), 0xff, 0x00, 00240 UNIT(2), 0x00, 0x00, 00241 REPORT_SIZE(1), 0x08, 00242 REPORT_COUNT(1), 0x04, 00243 INPUT(1), 0x02, 00244 00245 END_COLLECTION(0), 00246 END_COLLECTION(0), 00247 */ 00248 }; 00249 00250 reportLength = sizeof(reportDescriptor); 00251 return reportDescriptor; 00252 } 00253 00254
Generated on Thu Jul 14 2022 14:49:55 by
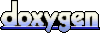