First Release
Embed:
(wiki syntax)
Show/hide line numbers
NiseKabuto.h
00001 #pragma once 00002 /** Class: In_CyberStick 00003 * 00004 * Use CyberStick(CZ-8NJ2) as fake XE-1AP(Kabutogani) MD mode 00005 * Use CyberStick(CZ-8NJ2) as SS Multi Controller 00006 * Use CyberStick(CZ-8NJ2) as PC-Engine Analog Controller 00007 * Use CyberStick(CZ-8NJ2) Digital mode as Famicom controller 00008 * Use CyberStick(CZ-8NJ2) as PlayStation3 USB Joystick 00009 * 00010 * Example: 00011 * 00012 */ 00013 #include "In_CyberStick.h" 00014 #include "In_MD6B.h" 00015 #include "Out_MD.h" 00016 #include "Out_SSMulCon.h" 00017 #include "Out_PCE.h" 00018 #include "Out_FC.h" 00019 #include "Out_PS3USB.h" 00020 #include "InputStatus.h" 00021 00022 static volatile int _Timer0Counter; 00023 00024 class NiseKabuto 00025 { 00026 public: 00027 // Public constants 00028 static const char CONFIG_INMODE_CYBERSTICK_ANALOG = 0; 00029 static const char CONFIG_INMODE_CYBERSTICK_DIGITAL = 1; 00030 static const char CONFIG_INMODE_MD6B = 2; 00031 00032 static const char CONFIGPIN_OUTMODE_PS3USB = 0; 00033 static const char CONFIGPIN_OUTMODE_SSMULCON = 1; 00034 static const char CONFIGPIN_OUTMODE_PCE = 2; 00035 static const char CONFIGPIN_OUTMODE_MD = 3; // changed!! 00036 static const char CONFIGPIN_OUTMODE_FC = 4; 00037 00038 // Public Method/Constructor 00039 NiseKabuto(PinName inputPins[], PinName outputPins[], PinName configurePins[]); 00040 void Show(void); 00041 00042 private: 00043 // Variables 00044 char _InputType; 00045 char _OutputType; 00046 In_CyberStick *_In_CyberStick; 00047 In_MD6B *_In_MD6B; 00048 Out_MD *_Out_MD; 00049 Out_SSMulCon *_Out_SSMulCon; 00050 Out_PCE *_Out_PCE; 00051 Out_FC *_Out_FC; 00052 Out_PS3USB *_Out_PS3USB; 00053 InputStatus _InputStatus; 00054 BusInOut _ConfigSwitch; 00055 00056 // Private Method 00057 void InitInterruptPriority(void); 00058 00059 void InitInput_CyberStick (PinName inputPins[]); 00060 void InitInput_MD6B (PinName inputPins[]); 00061 void InitOutput_MD (PinName outputPins[]); 00062 void InitOutput_SSMulCon (PinName outputPins[]); 00063 void InitOutput_PCE (PinName outputPins[]); 00064 void InitOutput_FC (PinName outputPins[]); 00065 void InitOutput_PS3USB (void); 00066 00067 public: 00068 static void WaitUs(uint32_t us); 00069 }; 00070
Generated on Thu Jul 14 2022 14:49:55 by
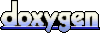