First Release
Embed:
(wiki syntax)
Show/hide line numbers
Out_PCE.h
00001 /** Class: Out_PCE 00002 * 00003 * Output class for PC-Engine Analog/Digital stick 00004 * 00005 */ 00006 00007 // ゲームごとによって微妙なタイミングの差異があると思われる。 00008 // XHE-3実機があればもっと調査できたかもしれない。 00009 00010 #include "mbed.h" 00011 #include "InputStatus.h" 00012 00013 class Out_PCE 00014 { 00015 public: 00016 /** Constructor: Out_PCE 00017 * 00018 * Parameters: 00019 * pn_1Y - DigitalOut for 1Y(Up/trigI) (PCE pad connector's pin2) 00020 * pn_2Y - DigitalOut for 2Y(Right/trigII) (pin3) 00021 * pn_3Y - DigitalOut for 3Y(Down/Select) (pin4) 00022 * pn_4Y - DigitalOut for 4Y(Left/Run) (pin5) 00023 * pn_DSEL - InterruptIn for DATASEL (pin6) 00024 * pn_ST - InterruptIn for /STROBE (pin7) 00025 * pn_POWDETECT - InterruptIn for PowerDetect (pin1) 00026 * inputStatus - Input status 00027 */ 00028 00029 Out_PCE( 00030 PinName pn_1Y, PinName pn_2Y, PinName pn_3Y, PinName pn_4Y, 00031 PinName pn_DSEL, PinName pn_ST, PinName pn_POWDETECT, 00032 InputStatus *inputStatus 00033 ); 00034 00035 private: 00036 // Private constants 00037 // ---- Analog ---- 00038 static const int TRANSFERSPEED_MAX__MICROSEC = 50; // from AJOY_SUB.DOC 00039 static const int TRANSFERSPEED_1_2__MICROSEC = 96; 00040 static const int TRANSFERSPEED_1_3__MICROSEC = 144; 00041 static const int TRANSFERSPEED_1_4__MICROSEC = 192; 00042 static const int MODECHECKPERIOD__MICROSEC = 5000; 00043 // ---- Digital ---- 00044 static const int DIGITALPAD_PHASECOUNT_MAX = 10; 00045 static const int DIGITALPAD_STATERENEWINTERVAL__MICROSEC= 5000; 00046 00047 // mbed pins 00048 DigitalOut _OUT_1Y; 00049 DigitalOut _OUT_2Y; 00050 DigitalOut _OUT_3Y; 00051 DigitalOut _OUT_4Y; 00052 InterruptIn _INTR_DSEL; 00053 InterruptIn _INTR_ST; 00054 InterruptIn _INTR_POWDETECT; 00055 00056 // Variable 00057 volatile char _OutputMode; 00058 InputStatus *_InputStatus; 00059 volatile char _NowWriting; 00060 volatile char _DataSelectStatus; 00061 00062 volatile char _PhaseCounter; 00063 Ticker _PhaseChangeTicker; 00064 int _TransferSpeed; 00065 00066 volatile char _Buttons; 00067 volatile char _Ch0; 00068 volatile char _Ch1; 00069 volatile char _Ch2; 00070 00071 volatile char _RapidFireValue; 00072 volatile char _PhaseData[12]; 00073 00074 Ticker _PCEPowerCheckTicker; 00075 volatile int _PCEActiveCounter; // 不要? 00076 00077 Ticker _DigitalPadPhase0SetTicker; 00078 00079 Ticker _ModeCheckTicker; 00080 00081 // Private Method 00082 void Initialize(void); 00083 void InitializePowerChecker(void); 00084 void SetOutputPinsValue(char dat); 00085 void SetOutputPinsValue2(char dat); 00086 void RenewPins(void); 00087 00088 void DSelFallISR(void); 00089 void DSelRiseISR(void); 00090 void StrobeFallISR(void); 00091 void StrobeRiseISR(void); 00092 00093 void AttachTicker(void); 00094 void DetachTicker(void); 00095 void ChangePhase(void); 00096 00097 void Enable_DigitalPadPhase0SetTicker(void); 00098 void Digital_TickerMethod(void); 00099 void Digital_SetDataOfPhase(char phaseCounter); 00100 // void InitInterruptPriority(void); 00101 void ModeChecker(void); 00102 00103 // 00104 // for InputControll 00105 // 00106 public: 00107 void SetupInputControll(void (*startInputFunction)(void), void (*stopInputFunction)(void)); 00108 class CDummy; 00109 template<class T> 00110 void SetupInputControll(T* inputInstance, void (T::*startInputMethod)(void), void (T::*stopInputMethod)(void)) 00111 { 00112 _InputInstance = (CDummy*) inputInstance; 00113 StartInputMethod = (void (CDummy::*)(void)) startInputMethod; 00114 StopInputMethod = (void (CDummy::*)(void)) stopInputMethod; 00115 } 00116 00117 private: 00118 CDummy* _InputInstance; 00119 void (CDummy::*StartInputMethod)(void); 00120 void (CDummy::*StopInputMethod)(void); 00121 void (*StartInputFunction)(void); 00122 void (*StopInputFunction)(void); 00123 void EnableInput(void); 00124 void DisableInput(void); 00125 00126 }; 00127 00128
Generated on Thu Jul 14 2022 14:49:55 by
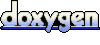