First Release
Embed:
(wiki syntax)
Show/hide line numbers
Cfunc_PCEDigital.cpp
00001 #include "mbed.h" 00002 #include "Cfunc_PCEDigital.h" 00003 00004 // mbed pins 00005 DigitalOut *_OUT_1Y; 00006 DigitalOut *_OUT_2Y; 00007 DigitalOut *_OUT_3Y; 00008 DigitalOut *_OUT_4Y; 00009 //BusOut *_OUT_BUS; 00010 InterruptIn *_INTR_DSEL; 00011 InterruptIn *_INTR_ST; 00012 00013 // File local variables 00014 static volatile char *_PhaseData; //PhaseData[12] 00015 static volatile char *_pRapidFireValue; 00016 static volatile char *_pTemp; 00017 00018 // Static variables 00019 static const int DIGITALPAD_PHASECOUNT_MAX = 10; 00020 00021 // File local functions 00022 void DSelRiseISR(void); 00023 //void StrobeRiseISR(void); 00024 static void SetOutputPinsValue(char dat); 00025 00026 00027 00028 void Cfunc_PCEDigital_Initialize( 00029 DigitalOut *out_1Y, 00030 DigitalOut *out_2Y, 00031 DigitalOut *out_3Y, 00032 DigitalOut *out_4Y, 00033 //BusOut *out_bus, 00034 InterruptIn *intr_DSEL, 00035 InterruptIn *intr_ST, 00036 volatile char *pPhaseData, 00037 volatile char *pRapidFireValue, 00038 volatile char *pTemp 00039 /* 00040 volatile char *pCh0, 00041 volatile char *pCh1, 00042 volatile char *pCh2, 00043 volatile int *pButtons, 00044 volatile char *pInputDeviceType 00045 */ 00046 ) 00047 { 00048 // 入出力pin 00049 _OUT_1Y = out_1Y; 00050 _OUT_2Y = out_2Y; 00051 _OUT_3Y = out_3Y; 00052 _OUT_4Y = out_4Y; 00053 //_OUT_BUS = out_bus; 00054 _INTR_DSEL = intr_DSEL; 00055 _INTR_ST = intr_ST; 00056 00057 // PhaseDataポインタ 00058 _PhaseData = pPhaseData; 00059 00060 _pRapidFireValue = pRapidFireValue; 00061 00062 _pTemp =pTemp; 00063 00064 // Inetrrupt Setting 00065 // _INTR_DSEL->rise(&DSelRiseISR); 00066 _INTR_ST->rise(&DSelRiseISR); 00067 00068 // Initialize pin status 00069 SetOutputPinsValue(0x00); 00070 00071 } 00072 00073 00074 void DSelRiseISR(void) 00075 { 00076 //printf("I\r\n"); 00077 ////////////////////////////////////// 00078 char dselStatus =0; 00079 //char loopCounter = 200; 00080 int loopCounter = 200; 00081 00082 // Phase0(DSEL=1)の期間、待機 00083 while( (_INTR_DSEL->read()) ) 00084 { 00085 00086 loopCounter--; 00087 if( loopCounter<0 ) 00088 { 00089 break; 00090 } 00091 00092 } 00093 00094 00095 // ただちにPhase1に移行 00096 // Set data for current phase 00097 SetOutputPinsValue(_PhaseData[1]); 00098 00099 // 開始 00100 for(int i=2; i< DIGITALPAD_PHASECOUNT_MAX; i++ ) 00101 { 00102 loopCounter = 200; 00103 00104 while( _INTR_DSEL->read()==dselStatus ) 00105 { 00106 00107 loopCounter--; 00108 if( loopCounter<0 ) 00109 { 00110 break; 00111 } 00112 00113 } 00114 00115 // Set data for current phase 00116 SetOutputPinsValue(_PhaseData[i]); 00117 00118 00119 dselStatus = !dselStatus; 00120 } 00121 00122 loopCounter = 200; 00123 while( _INTR_DSEL->read()==dselStatus ) 00124 { 00125 loopCounter--; 00126 if( loopCounter<0 ) 00127 { 00128 break; 00129 } 00130 } 00131 SetOutputPinsValue(_PhaseData[0]); 00132 00133 *_pRapidFireValue = !(*_pRapidFireValue); 00134 //printf("O\r\n"); 00135 (*_pTemp)++; // デバッグ用 00136 00137 //NVIC_EnableIRQ(TIMER3_IRQn); // Tickerルーチンが呼ばれなくなる問題 00138 //LPC_TIM3->IR = 0xff; // clear interrupt flags 00139 //LPC_TIM3->TCR = 1; // Enable Timer 悪化 00140 } 00141 00142 00143 00144 void SetOutputPinsValue(char dat) 00145 { 00146 _OUT_1Y->write( dat & 1 ); 00147 _OUT_2Y->write( (dat & 2) >> 1 ); 00148 _OUT_3Y->write( (dat & 4) >> 2 ); 00149 _OUT_4Y->write( (dat & 8) >> 3 ); 00150 //_OUT_BUS->write(dat); 00151 }
Generated on Thu Jul 14 2022 14:49:55 by
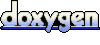