
Websocket Hello World over a wifi network
Dependencies: EthernetNetIf mbed DNSResolver
main.cpp
00001 #include "mbed.h" 00002 #include "Wifly.h" 00003 #include "Websocket.h" 00004 00005 DigitalOut l1(LED1); 00006 00007 //Here, we create an instance, with pins 9 and 10 connecting to the 00008 //WiFly's TX and RX pins, and pin 21 to RESET. We are connecting to the 00009 //"mbed" network, password "password", and we are using WPA. 00010 Wifly wifly(p9, p10, p21, "mbed", "password", true); 00011 00012 //Here, we create a Websocket instance in 'rw' (read-write) mode 00013 //on the 'samux' channel 00014 Websocket ws("ws://sockets.mbed.org/ws/samux/rw", &wifly); 00015 00016 00017 int main() { 00018 char recv[40]; 00019 00020 while (1) { 00021 00022 //we connect the network 00023 while (!wifly.join()) { 00024 wifly.reset(); 00025 } 00026 00027 //we connect to the websocket server 00028 while (!ws.connect()); 00029 00030 while (1) { 00031 wait(0.5); 00032 00033 //Send Hello world 00034 ws.send("Hello World! over Wifi"); 00035 00036 // if a message is available, print it 00037 if (ws.read(recv)) { 00038 // show that we receive messages 00039 l1 = !l1; 00040 } 00041 } 00042 } 00043 }
Generated on Tue Jul 12 2022 17:44:46 by
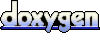