
USBKeyboard example with media keys
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "USBKeyboard.h" 00003 00004 USBKeyboard keyboard; 00005 00006 //Bus of buttons 00007 BusInOut buttons(p21, p22, p23, p24, p25, p26, p29); 00008 00009 int main(void) { 00010 uint8_t p_bus = 0; 00011 00012 while (1) { 00013 //if the bus of buttons has changed, send a report 00014 if (buttons.read() != p_bus) { 00015 p_bus = buttons.read(); 00016 if(p_bus & 0x01) 00017 keyboard.mediaControl(KEY_MUTE); 00018 if(p_bus & 0x02) 00019 keyboard.mediaControl(KEY_VOLUME_DOWN); 00020 if(p_bus & 0x04) 00021 keyboard.mediaControl(KEY_VOLUME_UP); 00022 if(p_bus & 0x08) 00023 keyboard.mediaControl(KEY_NEXT_TRACK); 00024 if(p_bus & 0x10) 00025 keyboard.mediaControl(KEY_PLAY_PAUSE); 00026 if(p_bus & 0x20) 00027 keyboard.mediaControl(KEY_PREVIOUS_TRACK); 00028 if(p_bus & 0x40) 00029 keyboard.printf("Hello World\r\n"); 00030 } 00031 wait(0.01); 00032 } 00033 }
Generated on Mon Jul 18 2022 12:50:00 by
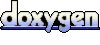