
OV7670 + 23LC1024 + Bluetooth
Dependencies: FastPWM MODSERIAL mbed
spi_ram.h
00001 #include "mbed.h" 00002 00003 #ifndef SPI_RAM_H 00004 #define SPI_RAM_H 00005 00006 // Mode codes 00007 #define BYTE_MODE 0x00 00008 #define SEQUENTIAL_MODE 0x40 00009 00010 // Command codes 00011 #define READ 0x03 00012 #define WRITE 0x02 00013 #define READ_STATUS 0x05 00014 #define WRITE_STATUS 0x01 00015 00016 // Underlying SPI constants 00017 #define TFE 0x02 00018 #define TNF 0x02 00019 #define RNE 0x04 00020 00021 class SRAM { 00022 public: 00023 00024 SRAM(SPI& spiDef, PinName csiPin); 00025 00026 char readStatus(); 00027 void writeStatus(char status); 00028 void startWriteSequence(); 00029 void startReadSequence(); 00030 void writeSequence0(char c); 00031 void writeSequence1(char c); 00032 char readSequence0(); 00033 char readSequence1(); 00034 void stopSequence(); 00035 00036 private: 00037 SPI& spi; 00038 DigitalOut csi; 00039 void prepareCommand(char command, int address); 00040 void select(); 00041 void deselect(); 00042 }; 00043 00044 #endif
Generated on Sun Jul 31 2022 14:40:01 by
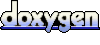