
A smart remote using the sparkfun IR transmitter and receiver. The program also uses a web server to show the buttons on a mobile platform.
Dependencies: EthernetInterface HTTPServer RemoteIR SDFileSystem mbed-rpc mbed-rtos mbed
Fork of SmartRemoteClean by
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetInterface.h" 00003 #include "HTTPServer.h" 00004 #include "FsHandler.h" 00005 #include "RpcHandler.h" 00006 #include "rtos.h" 00007 #include <string> 00008 00009 DigitalOut led1(LED1); 00010 DigitalOut led2(LED2); 00011 00012 //Start IR 00013 #include "ReceiverIR.h" 00014 #include "TransmitterIR.h" 00015 #include "IR.h" 00016 //END IR 00017 00018 00019 // Start DB 00020 #include <stdio.h> 00021 #include <stdlib.h> 00022 #include "SDFileSystem.h" 00023 #include "db.h" 00024 // End DB 00025 00026 00027 // Start RPC 00028 #include "RPCVariable.h" 00029 int Request = 0; 00030 int Learn = 0; 00031 char Learn_name0; 00032 char Learn_name1; 00033 char Learn_name2; 00034 char Learn_name3; 00035 char Learn_name4; 00036 char Learn_name5; 00037 char Learn_name6; 00038 char Learn_name7; 00039 char Learn_name8; 00040 char Learn_name9; 00041 00042 //Make these variables accessible over RPC by attaching them to an RPCVariable 00043 RPCVariable<int> RPCRequest(&Request, "Request"); 00044 RPCVariable<int> RPCLearn(&Learn, "Learn"); 00045 RPCVariable<char> RPCLearner0(&Learn_name0, "Learn_name0"); 00046 RPCVariable<char> RPCLearner1(&Learn_name1, "Learn_name1"); 00047 RPCVariable<char> RPCLearner2(&Learn_name2, "Learn_name2"); 00048 RPCVariable<char> RPCLearner3(&Learn_name3, "Learn_name3"); 00049 RPCVariable<char> RPCLearner4(&Learn_name4, "Learn_name4"); 00050 RPCVariable<char> RPCLearner5(&Learn_name5, "Learn_name5"); 00051 RPCVariable<char> RPCLearner6(&Learn_name6, "Learn_name6"); 00052 RPCVariable<char> RPCLearner7(&Learn_name7, "Learn_name7"); 00053 RPCVariable<char> RPCLearner8(&Learn_name8, "Learn_name8"); 00054 RPCVariable<char> RPCLearner9(&Learn_name9, "Learn_name9"); 00055 // End RPC 00056 00057 00058 Serial pc(USBTX, USBRX, "pc"); 00059 00060 // Instantiate a HTTPServer to handle incoming requests 00061 HTTPServer svr; 00062 // Instantiate a local file system handler named 'local' which will be used later to access files on the mbed. 00063 LocalFileSystem local("local"); 00064 00065 SDFileSystem sd(p5, p6, p7, p8, "sd"); // the pinout on the mbed 00066 00067 int main() 00068 { 00069 00070 printf("Setting up Apache...\n \r"); 00071 00072 HTTPFsRequestHandler::mount("/local/", "/"); 00073 HTTPFsRequestHandler::mount("/sd/", "/sd/"); 00074 svr.addHandler<HTTPFsRequestHandler>("/"); 00075 svr.addHandler<HTTPRpcRequestHandler>("/rpc"); 00076 00077 EthernetInterface eth; 00078 eth.init(); //Use DHCP 00079 eth.connect(); 00080 00081 // Now start the server on port 80. 00082 if (!svr.start(80, ð)) { 00083 error("Server not starting !"); 00084 exit(0); 00085 } 00086 00087 printf("IP: %s\n \r", eth.getIPAddress()); 00088 printf("Setup OK\n \r"); 00089 00090 00091 00092 00093 // DB Init 00094 mkdir("/sd/SmartRemote", 0777); 00095 00096 char tuple_code[128]; 00097 char tuple_name[128]; 00098 char tuple_bitlength[128]; 00099 char tuple_format[128]; 00100 char temp[20]; 00101 int n; 00102 //End DB init 00103 00104 00105 //IR Init 00106 uint8_t buf1[32]; 00107 uint8_t buf2[32]; 00108 int bitlength1; 00109 int bitlength2; 00110 char tempstr[3]; 00111 RemoteIR::Format format; 00112 memset(buf1, 0x00, sizeof(buf1)); 00113 memset(buf2, 0x00, sizeof(buf2)); 00114 //END IR Init 00115 00116 printf("Listening...\n \r"); 00117 00118 00119 Timer tm; 00120 tm.start(); 00121 //Listen indefinitely 00122 while(true) { 00123 svr.poll(); 00124 if(tm.read()>.5) { 00125 tm.start(); 00126 } 00127 if (Learn) { 00128 // Debug LED 00129 led1 = 1; 00130 00131 // Receive the code 00132 { 00133 bitlength1 = receive(&format, buf1, sizeof(buf1)); 00134 if (bitlength1 < 0) { 00135 continue; 00136 } 00137 display_status("RECV", bitlength1); 00138 display_data(buf1, bitlength1); 00139 //display_format(format); 00140 } 00141 // Reset 00142 led1 = 0; 00143 Learn = 0; 00144 00145 00146 00147 // Set up the variables 00148 sprintf(tuple_name, "%c%c%c%c%c%c%c%c%c%c", Learn_name0,Learn_name1,Learn_name2,Learn_name3,Learn_name4,Learn_name5,Learn_name6,Learn_name7,Learn_name8,Learn_name9); 00149 //sprintf(tuple_code, "%X", buf1); 00150 sprintf(tuple_bitlength, "%d", bitlength1); 00151 sprintf(tuple_format, "%d", format); 00152 for (int i = 0; i < 10; i++) { 00153 if (tuple_name[i] == '~') tuple_name[i] = ' '; 00154 } 00155 00156 const int n = bitlength1 / 8 + (((bitlength1 % 8) != 0) ? 1 : 0); 00157 strcpy(tuple_code, ""); 00158 for (int i = 0; i < n; i++) { 00159 sprintf(temp, "%02X", buf1[i]); 00160 strcat(tuple_code, temp); 00161 } 00162 00163 // Insert into DB 00164 db_insert_tuple(tuple_name, tuple_code, tuple_bitlength, tuple_format); 00165 00166 } 00167 00168 if ( Request != 0) { 00169 led2 = 1; 00170 db_find_tuple(Request, tuple_name, tuple_code, tuple_bitlength, tuple_format); 00171 00172 n = atoi(tuple_bitlength) / 8 + (((atoi(tuple_bitlength) % 8) != 0) ? 1 : 0); 00173 memset(buf1, 0x00, sizeof(buf1)); 00174 int j = 0 ; 00175 for (int i = 0; i < 2*n; i+=2) { 00176 00177 // printf("%02X", buf[i]); 00178 // buf1[i] = (uint8_t)(atoi( tuple_code.substr(i, 2) )); 00179 sprintf(tempstr,"%c%c", tuple_code[i],tuple_code[i+1] ); 00180 printf("%s - ", tempstr); 00181 printf("%02X\n", (uint8_t)strtol(tempstr,NULL,16) ); 00182 buf1[j] = (uint8_t)strtol(tempstr,NULL,16); 00183 j++; 00184 } 00185 display_data(buf1,atoi(tuple_bitlength)); 00186 00187 { 00188 RemoteIR::Format f = static_cast<RemoteIR::Format>(atoi(tuple_format)); 00189 bitlength1 = transmit(f, buf1, atoi(tuple_bitlength)); 00190 if (bitlength1 < 0) { 00191 continue; 00192 } 00193 display_status("TRAN", bitlength1); 00194 //display_data(buf1, bitlength1); 00195 //display_format(format); 00196 } 00197 led2 = 0; 00198 Request = 0; 00199 00200 } 00201 00202 } 00203 00204 return 0; 00205 } 00206
Generated on Wed Jul 13 2022 20:07:14 by
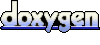