
updated RPC command to match javascripting language
Dependencies: EthernetInterface HTTPServerExample mbed-rpc mbed-rtos mbed
Fork of EthHTTPServer by
main.cpp
00001 #include "mbed.h" 00002 #include "HTTPServer.h" 00003 #include "FsHandler.h" 00004 #include "LocalFileSystem.h" 00005 #include "RpcHandler.h" 00006 #include "mbed_rpc.h" 00007 00008 00009 00010 // Use LED1 to indicate that the main loop is still executing 00011 DigitalOut myled(LED1); 00012 // Use the serial connection 'pc' to dump debug information 00013 Serial pc(USBTX, USBRX, "pc"); 00014 // Instantiate a HTTPServer to handle incoming requests 00015 HTTPServer svr; 00016 // Instantiate a local file system handler named 'local' which will be used later to access files on the mbed. 00017 LocalFileSystem local("local"); 00018 00019 // Create the EthernetInterface. This is optional, please see the documentation of HTTP Server's start method. 00020 EthernetInterface eth; 00021 00022 //Make these variables accessible over RPC by attaching them to an RPCVariable 00023 int Request = 0; 00024 RPCVariable<int> RPCRequest(&Request, "Request"); 00025 00026 int main() { 00027 00028 HTTPFsRequestHandler::mount("/local/", "/"); // Mount /local/ filesystem as root web path / 00029 svr.addHandler<HTTPFsRequestHandler>("/"); // Serve all default HTTP requests 00030 svr.addHandler<HTTPRpcRequestHandler>("/rpc"); // Serve all RPC requests 00031 00032 00033 // Initialize the EthernetInterface and initiate a connection using default DHCP. 00034 eth.init(); 00035 eth.connect(); 00036 00037 if (!svr.start(80, ð)) { 00038 error("Server not starting !"); 00039 exit(0); 00040 } 00041 00042 while(1) { 00043 svr.poll(); 00044 myled = Request; 00045 } 00046 }
Generated on Wed Jul 20 2022 01:31:46 by
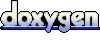