eeprom adding
Fork of SEEED_CAN by
Embed:
(wiki syntax)
Show/hide line numbers
seeed_can_spi.h
00001 /* seeed_can_spi.h 00002 * Copyright (c) 2013 Sophie Dexter 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef _SEEED_CAN_SPI_H_ 00017 #define _SEEED_CAN_SPI_H_ 00018 00019 #include "seeed_can_defs.h" 00020 00021 #ifdef __cplusplus 00022 extern "C" { 00023 #endif 00024 00025 /** CAN driver typedefs 00026 */ 00027 /// Type definition to hold a Seeed Studios CAN-BUS Shield connections and resources structure 00028 struct Seeed_MCP_CAN_Shield { 00029 SPI spi; 00030 DigitalOut ncs; 00031 InterruptIn irq; 00032 Seeed_MCP_CAN_Shield(SPI _spi_, DigitalOut _ncs_, InterruptIn _irq_) : 00033 spi(_spi_), 00034 ncs(_ncs_), 00035 irq(_irq_) 00036 {} 00037 }; 00038 typedef struct Seeed_MCP_CAN_Shield mcp_can_t; 00039 00040 /** mcp2515 spi instructions 00041 */ 00042 void mcpReset(mcp_can_t *obj); // reset the MCP2515 CAN controller chip 00043 00044 uint8_t mcpRead(mcp_can_t *obj, // read from a single MCP2512 register 00045 const uint8_t address); 00046 void mcpReadMultiple(mcp_can_t *obj, // read multiple, sequential, registers into an array 00047 const uint8_t address, 00048 uint8_t values[], 00049 const uint8_t n); 00050 void mcpReadBuffer(mcp_can_t *obj, // read the specified receive buffer into an array 00051 const uint8_t command, 00052 uint8_t values[], 00053 const uint8_t n); 00054 void mcpWrite(mcp_can_t *obj, // write to a single MCP2512 register 00055 const uint8_t address, 00056 const uint8_t value); 00057 void mcpWriteMultiple(mcp_can_t *obj, // write an array into consecutive MCP2515 registers 00058 const uint8_t address, 00059 const uint8_t values[], 00060 const uint8_t n); 00061 void mcpWriteBuffer(mcp_can_t *obj, // write an array into the specified transmit buffer 00062 const uint8_t command, 00063 uint8_t values[], 00064 const uint8_t n); 00065 void mcpBufferRTS(mcp_can_t *obj, const uint8_t command); // initiate transmission of the specified MCP2515 transmit buffer 00066 uint8_t mcpStatus(mcp_can_t *obj); // read the MCP2515's status register 00067 uint8_t mcpReceiveStatus(mcp_can_t *obj); // read mcp2515's receive status register 00068 void mcpBitModify(mcp_can_t *obj, // modify bits of a register specified by a mask 00069 const uint8_t address, 00070 const uint8_t mask, 00071 const uint8_t data); 00072 00073 #ifdef __cplusplus 00074 }; 00075 #endif 00076 00077 #endif // SEEED_CAN_SPI_H
Generated on Tue Jul 12 2022 19:07:56 by
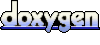