
CANfestival - an open source CANopen framework
Embed:
(wiki syntax)
Show/hide line numbers
data.h
00001 /* 00002 This file is part of CanFestival, a library implementing CanOpen Stack. 00003 00004 Copyright (C): Edouard TISSERANT and Francis DUPIN 00005 00006 See COPYING file for copyrights details. 00007 00008 This library is free software; you can redistribute it and/or 00009 modify it under the terms of the GNU Lesser General Public 00010 License as published by the Free Software Foundation; either 00011 version 2.1 of the License, or (at your option) any later version. 00012 00013 This library is distributed in the hope that it will be useful, 00014 but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00016 Lesser General Public License for more details. 00017 00018 You should have received a copy of the GNU Lesser General Public 00019 License along with this library; if not, write to the Free Software 00020 Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA 00021 */ 00022 00023 #ifndef __data_h__ 00024 #define __data_h__ 00025 00026 #ifdef __cplusplus 00027 extern "C" { 00028 #endif 00029 00030 /* declaration of CO_Data type let us include all necessary headers 00031 struct struct_CO_Data can then be defined later 00032 */ 00033 typedef struct struct_CO_Data CO_Data; 00034 00035 #include "applicfg.h" 00036 #include "def.h" 00037 #include "canopen_can.h" 00038 #include "objdictdef.h" 00039 #include "objacces.h" 00040 #include "sdo.h" 00041 #include "pdo.h" 00042 #include "states.h" 00043 #include "lifegrd.h" 00044 #include "sync.h" 00045 #include "nmtSlave.h" 00046 #include "nmtMaster.h" 00047 #include "emcy.h" 00048 #ifdef CO_ENABLE_LSS 00049 #include "lss.h" 00050 #endif 00051 00052 /** 00053 * @ingroup od 00054 * @brief This structure contains all necessary informations to define a CANOpen node 00055 */ 00056 struct struct_CO_Data { 00057 /* Object dictionary */ 00058 UNS8 *bDeviceNodeId; 00059 const indextable *objdict; 00060 s_PDO_status *PDO_status; 00061 const quick_index *firstIndex; 00062 const quick_index *lastIndex; 00063 const UNS16 *ObjdictSize; 00064 const UNS8 *iam_a_slave; 00065 valueRangeTest_t valueRangeTest; 00066 00067 /* SDO */ 00068 s_transfer transfers[SDO_MAX_SIMULTANEOUS_TRANSFERTS]; 00069 /* s_sdo_parameter *sdo_parameters; */ 00070 00071 /* State machine */ 00072 e_nodeState nodeState; 00073 s_state_communication CurrentCommunicationState; 00074 initialisation_t initialisation; 00075 preOperational_t preOperational; 00076 operational_t operational; 00077 stopped_t stopped; 00078 void (*NMT_Slave_Node_Reset_Callback)(CO_Data*); 00079 void (*NMT_Slave_Communications_Reset_Callback)(CO_Data*); 00080 00081 /* NMT-heartbeat */ 00082 UNS8 *ConsumerHeartbeatCount; 00083 UNS32 *ConsumerHeartbeatEntries; 00084 TIMER_HANDLE *ConsumerHeartBeatTimers; 00085 UNS16 *ProducerHeartBeatTime; 00086 TIMER_HANDLE ProducerHeartBeatTimer; 00087 heartbeatError_t heartbeatError; 00088 e_nodeState NMTable[NMT_MAX_NODE_ID]; 00089 00090 /* SYNC */ 00091 TIMER_HANDLE syncTimer; 00092 UNS32 *COB_ID_Sync; 00093 UNS32 *Sync_Cycle_Period; 00094 /*UNS32 *Sync_window_length;;*/ 00095 post_sync_t post_sync; 00096 post_TPDO_t post_TPDO; 00097 post_SlaveBootup_t post_SlaveBootup; 00098 post_SlaveStateChange_t post_SlaveStateChange; 00099 00100 /* General */ 00101 UNS8 toggle; 00102 CAN_PORT canHandle; 00103 scanIndexOD_t scanIndexOD; 00104 storeODSubIndex_t storeODSubIndex; 00105 00106 /* DCF concise */ 00107 const indextable* dcf_odentry; 00108 UNS8* dcf_cursor; 00109 UNS32 dcf_entries_count; 00110 UNS8 dcf_request; 00111 00112 /* EMCY */ 00113 e_errorState error_state; 00114 UNS8 error_history_size; 00115 UNS8* error_number; 00116 UNS32* error_first_element; 00117 UNS8* error_register; 00118 UNS32* error_cobid; 00119 s_errors error_data[EMCY_MAX_ERRORS]; 00120 post_emcy_t post_emcy; 00121 00122 #ifdef CO_ENABLE_LSS 00123 /* LSS */ 00124 lss_transfer_t lss_transfer; 00125 lss_StoreConfiguration_t lss_StoreConfiguration; 00126 #endif 00127 }; 00128 00129 #define NMTable_Initializer Unknown_state, 00130 00131 #ifdef SDO_DYNAMIC_BUFFER_ALLOCATION 00132 #define s_transfer_Initializer {\ 00133 0, /* nodeId */\ 00134 0, /* wohami */\ 00135 SDO_RESET, /* state */\ 00136 0, /* toggle */\ 00137 0, /* abortCode */\ 00138 0, /* index */\ 00139 0, /* subIndex */\ 00140 0, /* count */\ 00141 0, /* offset */\ 00142 {0}, /* data (static use, so that all the table is initialize at 0)*/\ 00143 NULL, /* dynamicData */ \ 00144 0, /* dynamicDataSize */ \ 00145 0, /* dataType */\ 00146 -1, /* timer */\ 00147 NULL /* Callback */\ 00148 }, 00149 #else 00150 #define s_transfer_Initializer {\ 00151 0, /* nodeId */\ 00152 0, /* wohami */\ 00153 SDO_RESET, /* state */\ 00154 0, /* toggle */\ 00155 0, /* abortCode */\ 00156 0, /* index */\ 00157 0, /* subIndex */\ 00158 0, /* count */\ 00159 0, /* offset */\ 00160 {0}, /* data (static use, so that all the table is initialize at 0)*/\ 00161 0, /* dataType */\ 00162 -1, /* timer */\ 00163 NULL /* Callback */\ 00164 }, 00165 #endif //SDO_DYNAMIC_BUFFER_ALLOCATION 00166 00167 #define ERROR_DATA_INITIALIZER \ 00168 {\ 00169 0, /* errCode */\ 00170 0, /* errRegMask */\ 00171 0 /* active */\ 00172 }, 00173 00174 #ifdef CO_ENABLE_LSS 00175 00176 #ifdef CO_ENABLE_LSS_FS 00177 #define lss_fs_Initializer \ 00178 ,0, /* IDNumber */\ 00179 128, /* BitChecked */\ 00180 0, /* LSSSub */\ 00181 0, /* LSSNext */\ 00182 0, /* LSSPos */\ 00183 LSS_FS_RESET, /* FastScan_SM */\ 00184 -1, /* timerFS */\ 00185 {{0,0,0,0},{0,0,0,0}} /* lss_fs_transfer */ 00186 #else 00187 #define lss_fs_Initializer 00188 #endif 00189 00190 #define lss_Initializer {\ 00191 LSS_RESET, /* state */\ 00192 0, /* command */\ 00193 LSS_WAITING_MODE, /* mode */\ 00194 0, /* dat1 */\ 00195 0, /* dat2 */\ 00196 0, /* NodeID */\ 00197 0, /* addr_sel_match */\ 00198 0, /* addr_ident_match */\ 00199 "none", /* BaudRate */\ 00200 0, /* SwitchDelay */\ 00201 SDELAY_OFF, /* SwitchDelayState */\ 00202 NULL, /* canHandle_t */\ 00203 -1, /* TimerMSG */\ 00204 -1, /* TimerSDELAY */\ 00205 NULL, /* Callback */\ 00206 0 /* LSSanswer */\ 00207 lss_fs_Initializer /*FastScan service initialization */\ 00208 },\ 00209 NULL /* _lss_StoreConfiguration*/ 00210 #else 00211 #define lss_Initializer 00212 #endif 00213 00214 00215 /* A macro to initialize the data in client app.*/ 00216 /* CO_Data structure */ 00217 #define CANOPEN_NODE_DATA_INITIALIZER(NODE_PREFIX) {\ 00218 /* Object dictionary*/\ 00219 & NODE_PREFIX ## _bDeviceNodeId, /* bDeviceNodeId */\ 00220 NODE_PREFIX ## _objdict, /* objdict */\ 00221 NODE_PREFIX ## _PDO_status, /* PDO_status */\ 00222 & NODE_PREFIX ## _firstIndex, /* firstIndex */\ 00223 & NODE_PREFIX ## _lastIndex, /* lastIndex */\ 00224 & NODE_PREFIX ## _ObjdictSize, /* ObjdictSize */\ 00225 & NODE_PREFIX ## _iam_a_slave, /* iam_a_slave */\ 00226 NODE_PREFIX ## _valueRangeTest, /* valueRangeTest */\ 00227 \ 00228 /* SDO, structure s_transfer */\ 00229 {\ 00230 REPEAT_SDO_MAX_SIMULTANEOUS_TRANSFERTS_TIMES(s_transfer_Initializer)\ 00231 },\ 00232 \ 00233 /* State machine*/\ 00234 Unknown_state, /* nodeState */\ 00235 /* structure s_state_communication */\ 00236 {\ 00237 0, /* csBoot_Up */\ 00238 0, /* csSDO */\ 00239 0, /* csEmergency */\ 00240 0, /* csSYNC */\ 00241 0, /* csHeartbeat */\ 00242 0, /* csPDO */\ 00243 0 /* csLSS */\ 00244 },\ 00245 _initialisation, /* initialisation */\ 00246 _preOperational, /* preOperational */\ 00247 _operational, /* operational */\ 00248 _stopped, /* stopped */\ 00249 NULL, /* NMT node reset callback */\ 00250 NULL, /* NMT communications reset callback */\ 00251 \ 00252 /* NMT-heartbeat */\ 00253 & NODE_PREFIX ## _highestSubIndex_obj1016, /* ConsumerHeartbeatCount */\ 00254 NODE_PREFIX ## _obj1016, /* ConsumerHeartbeatEntries */\ 00255 NODE_PREFIX ## _heartBeatTimers, /* ConsumerHeartBeatTimers */\ 00256 & NODE_PREFIX ## _obj1017, /* ProducerHeartBeatTime */\ 00257 TIMER_NONE, /* ProducerHeartBeatTimer */\ 00258 _heartbeatError, /* heartbeatError */\ 00259 \ 00260 {REPEAT_NMT_MAX_NODE_ID_TIMES(NMTable_Initializer)},\ 00261 /* is well initialized at "Unknown_state". Is it ok ? (FD)*/\ 00262 \ 00263 /* SYNC */\ 00264 TIMER_NONE, /* syncTimer */\ 00265 & NODE_PREFIX ## _obj1005, /* COB_ID_Sync */\ 00266 & NODE_PREFIX ## _obj1006, /* Sync_Cycle_Period */\ 00267 /*& NODE_PREFIX ## _obj1007, */ /* Sync_window_length */\ 00268 _post_sync, /* post_sync */\ 00269 _post_TPDO, /* post_TPDO */\ 00270 _post_SlaveBootup, /* post_SlaveBootup */\ 00271 _post_SlaveStateChange, /* post_SlaveStateChange */\ 00272 \ 00273 /* General */\ 00274 0, /* toggle */\ 00275 NULL, /* canSend */\ 00276 NODE_PREFIX ## _scanIndexOD, /* scanIndexOD */\ 00277 _storeODSubIndex, /* storeODSubIndex */\ 00278 /* DCF concise */\ 00279 NULL, /*dcf_odentry*/\ 00280 NULL, /*dcf_cursor*/\ 00281 1, /*dcf_entries_count*/\ 00282 0, /* dcf_request*/\ 00283 \ 00284 /* EMCY */\ 00285 Error_free, /* error_state */\ 00286 sizeof(NODE_PREFIX ## _obj1003) / sizeof(NODE_PREFIX ## _obj1003[0]), /* error_history_size */\ 00287 & NODE_PREFIX ## _highestSubIndex_obj1003, /* error_number */\ 00288 & NODE_PREFIX ## _obj1003[0], /* error_first_element */\ 00289 & NODE_PREFIX ## _obj1001, /* error_register */\ 00290 & NODE_PREFIX ## _obj1014, /* error_cobid */\ 00291 /* error_data: structure s_errors */\ 00292 {\ 00293 REPEAT_EMCY_MAX_ERRORS_TIMES(ERROR_DATA_INITIALIZER)\ 00294 },\ 00295 _post_emcy, /* post_emcy */\ 00296 /* LSS */\ 00297 lss_Initializer\ 00298 } 00299 00300 #ifdef __cplusplus 00301 }; 00302 #endif 00303 00304 #endif /* __data_h__ */ 00305 00306
Generated on Tue Jul 12 2022 17:24:12 by
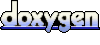