adding additional features
Embed:
(wiki syntax)
Show/hide line numbers
USBSerial.cpp
00001 /* Copyright (c) 2010-2011 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "stdint.h" 00020 #include "USBSerial.h" 00021 #include <stdarg.h> 00022 00023 USBSerial::USBSerial( uint16_t vendor_id, uint16_t product_id, uint16_t product_release ) 00024 : USBCDC( vendor_id, product_id, product_release ) 00025 , buf( 128 ) 00026 #ifdef USB_ERRATA_WORKAROUND 00027 , _connect( P0_6 ) 00028 , _vbus( P0_3 ) 00029 #endif 00030 { 00031 #ifdef USB_ERRATA_WORKAROUND 00032 _connect = 1; 00033 #endif 00034 return; 00035 } 00036 00037 int USBSerial::_putc( int c ) 00038 { 00039 send( ( uint8_t * )&c, 1 ); 00040 return 1; 00041 } 00042 00043 int USBSerial::_getc() 00044 { 00045 uint8_t c; 00046 00047 while ( buf.isEmpty() ); 00048 00049 buf.dequeue( &c ); 00050 return c; 00051 } 00052 00053 void USBSerial::flush( void ) 00054 { 00055 while( available() ) 00056 { 00057 _getc(); 00058 } 00059 00060 return; 00061 } 00062 00063 void USBSerial::printf( const char *format, ... ) 00064 { 00065 static char buf[128]; 00066 uint32_t len = 0; 00067 va_list arg; 00068 00069 if ( strlen( format ) < 64 ) 00070 { 00071 va_start( arg, format ); 00072 vsprintf( buf, format, arg ); 00073 va_end( arg ); 00074 // write to the peripheral 00075 len = strlen( buf ); 00076 send( ( uint8_t * )buf, len ); 00077 // and erase the buffer conents 00078 memset( buf, 0, len ); 00079 } 00080 00081 return; 00082 } 00083 00084 bool USBSerial::writeBlock( uint8_t *buf, uint16_t size ) 00085 { 00086 if( size > MAX_PACKET_SIZE_EPBULK ) 00087 { 00088 return false; 00089 } 00090 00091 if( !send( buf, size ) ) 00092 { 00093 return false; 00094 } 00095 00096 return true; 00097 } 00098 00099 bool USBSerial::writeBlock( const char *buf ) 00100 { 00101 uint16_t cnt = 0; 00102 00103 while( buf[cnt++] != 0 ); 00104 00105 return writeBlock( ( uint8_t * )buf, cnt ); 00106 } 00107 00108 bool USBSerial::EP2_OUT_callback() 00109 { 00110 uint8_t c[65]; 00111 uint32_t size = 0; 00112 //we read the packet received and put it on the circular buffer 00113 readEP( c, &size ); 00114 00115 for ( int i = 0; i < size; i++ ) 00116 { 00117 buf.queue( c[i] ); 00118 } 00119 00120 //call a potential handler 00121 rx.call(); 00122 // We reactivate the endpoint to receive next characters 00123 readStart( EPBULK_OUT, MAX_PACKET_SIZE_EPBULK ); 00124 return true; 00125 } 00126 00127 uint8_t USBSerial::available() 00128 { 00129 return buf.available(); 00130 } 00131 00132 #ifdef USB_ERRATA_WORKAROUND 00133 void USBSerial::connect( void ) 00134 { 00135 _connect = 0; 00136 USBHAL::connect(); 00137 return; 00138 } 00139 void USBSerial::disconnect( void ) 00140 { 00141 _connect = 1; 00142 USBHAL::disconnect(); 00143 return; 00144 } 00145 bool USBSerial::vbusDetected( void ) 00146 { 00147 return _vbus; 00148 } 00149 #endif 00150 00151
Generated on Tue Jul 12 2022 13:29:43 by
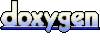