adding additional features
Embed:
(wiki syntax)
Show/hide line numbers
USBCDC.h
00001 /* Copyright (c) 2010-2011 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef USBCDC_H 00020 #define USBCDC_H 00021 00022 /* These headers are included for child class. */ 00023 #include "USBDevice/USBDevice/USBEndpoints.h" 00024 #include "USBDevice/USBDevice/USBDescriptor.h" 00025 #include "USBDevice/USBDevice/USBDevice_Types.h" 00026 00027 #include "USBDevice/USBDevice/USBDevice.h" 00028 00029 class USBCDC: public USBDevice 00030 { 00031 private: 00032 volatile uint8_t terminal_status; 00033 00034 public: 00035 00036 /* 00037 * Constructor 00038 * 00039 * @param vendor_id Your vendor_id 00040 * @param product_id Your product_id 00041 * @param product_release Your preoduct_release 00042 */ 00043 USBCDC( uint16_t vendor_id, uint16_t product_id, uint16_t product_release ); 00044 00045 uint8_t terminalIsOpen( void ); 00046 00047 protected: 00048 00049 /* 00050 * Get device descriptor. Warning: this method has to store the length of the report descriptor in reportLength. 00051 * 00052 * @returns pointer to the device descriptor 00053 */ 00054 virtual uint8_t *deviceDesc(); 00055 00056 /* 00057 * Get string product descriptor 00058 * 00059 * @returns pointer to the string product descriptor 00060 */ 00061 virtual uint8_t *stringIproductDesc(); 00062 00063 /* 00064 * Get string interface descriptor 00065 * 00066 * @returns pointer to the string interface descriptor 00067 */ 00068 virtual uint8_t *stringIinterfaceDesc(); 00069 00070 /* 00071 * Get configuration descriptor 00072 * 00073 * @returns pointer to the configuration descriptor 00074 */ 00075 virtual uint8_t *configurationDesc(); 00076 00077 /* 00078 * Send a buffer 00079 * 00080 * @param endpoint endpoint which will be sent the buffer 00081 * @param buffer buffer to be sent 00082 * @param size length of the buffer 00083 * @returns true if successful 00084 */ 00085 bool send( uint8_t *buffer, uint32_t size ); 00086 00087 /* 00088 * Read a buffer from a certain endpoint. Warning: blocking 00089 * 00090 * @param endpoint endpoint to read 00091 * @param buffer buffer where will be stored bytes 00092 * @param size the number of bytes read will be stored in *size 00093 * @param maxSize the maximum length that can be read 00094 * @returns true if successful 00095 */ 00096 bool readEP( uint8_t *buffer, uint32_t *size ); 00097 00098 /* 00099 * Read a buffer from a certain endpoint. Warning: non blocking 00100 * 00101 * @param endpoint endpoint to read 00102 * @param buffer buffer where will be stored bytes 00103 * @param size the number of bytes read will be stored in *size 00104 * @param maxSize the maximum length that can be read 00105 * @returns true if successful 00106 */ 00107 bool readEP_NB( uint8_t *buffer, uint32_t *size ); 00108 00109 virtual bool USBCallback_request(); 00110 virtual bool USBCallback_setConfiguration( uint8_t configuration ); 00111 00112 }; 00113 00114 #endif 00115
Generated on Tue Jul 12 2022 13:29:43 by
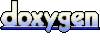