ssh
Embed:
(wiki syntax)
Show/hide line numbers
log.c
00001 /* log.c 00002 * 00003 * Copyright (C) 2014-2016 wolfSSL Inc. 00004 * 00005 * This file is part of wolfSSH. 00006 * 00007 * wolfSSH is free software; you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation; either version 3 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * wolfSSH is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with wolfSSH. If not, see <http://www.gnu.org/licenses/>. 00019 */ 00020 00021 00022 /* 00023 * The log module contains the interface to the logging function. When 00024 * debugging is enabled and turned on, the logger will output to STDOUT. 00025 * A custom logging callback may be installed. 00026 */ 00027 00028 00029 #ifdef HAVE_CONFIG_H 00030 #include <config.h> 00031 #endif 00032 00033 #include <wolfssh/ssh.h> 00034 #include <wolfssh/log.h> 00035 #include <wolfssh/error.h> 00036 00037 #include <stdlib.h> 00038 #include <stdio.h> 00039 #include <stdarg.h> 00040 #ifndef NO_TIMESTAMP 00041 //#include <time.h> 00042 #endif 00043 00044 //#include "esp_log.h" 00045 00046 00047 #ifndef WOLFSSH_DEFAULT_LOG_WIDTH 00048 #define WOLFSSH_DEFAULT_LOG_WIDTH 120 00049 #endif 00050 00051 00052 static const char *TAG = "WOLFSSH"; 00053 00054 00055 static void DefaultLoggingCb(enum wolfSSH_LogLevel, const char *const); 00056 00057 static wolfSSH_LoggingCb logFunction = DefaultLoggingCb; 00058 static enum wolfSSH_LogLevel logLevel = WS_LOG_USER; // esp32: max 00059 #ifdef DEBUG_WOLFSSH 00060 static int logEnable = 0; 00061 #endif 00062 00063 00064 /* turn debugging on if supported */ 00065 void wolfSSH_Debugging_ON(void) 00066 { 00067 #ifdef DEBUG_WOLFSSH 00068 logEnable = 1; 00069 #endif 00070 } 00071 00072 00073 /* turn debugging off */ 00074 void wolfSSH_Debugging_OFF(void) 00075 { 00076 #ifdef DEBUG_WOLFSSH 00077 logEnable = 0; 00078 #endif 00079 } 00080 00081 00082 /* set logging callback function */ 00083 void wolfSSH_SetLoggingCb(wolfSSH_LoggingCb logF) 00084 { 00085 if (logF) 00086 logFunction = logF; 00087 } 00088 00089 00090 int wolfSSH_LogEnabled(void) 00091 { 00092 #ifdef DEBUG_WOLFSSH 00093 return logEnable; 00094 #else 00095 return 0; 00096 #endif 00097 } 00098 00099 00100 /* log level string */ 00101 static const char* GetLogStr(enum wolfSSH_LogLevel level) 00102 { 00103 switch (level) { 00104 case WS_LOG_INFO: 00105 return "INFO"; 00106 00107 case WS_LOG_WARN: 00108 return "WARNING"; 00109 00110 case WS_LOG_ERROR: 00111 return "ERROR"; 00112 00113 case WS_LOG_DEBUG: 00114 return "DEBUG"; 00115 00116 case WS_LOG_USER: 00117 return "USER"; 00118 00119 default: 00120 return "UNKNOWN"; 00121 } 00122 } 00123 00124 00125 void DefaultLoggingCb(enum wolfSSH_LogLevel level, const char *const msgStr) 00126 { 00127 char timeStr[80]; 00128 timeStr[0] = '\0'; 00129 #ifndef NO_TIMESTAMP 00130 { 00131 time_t current; 00132 struct tm local; 00133 00134 current = time(NULL); 00135 if (WLOCALTIME(¤t, &local)) { 00136 /* make pretty */ 00137 strftime(timeStr, sizeof(timeStr), "%b %d %T %Y: ", &local); 00138 } 00139 } 00140 #endif /* NO_TIMESTAMP */ 00141 //fprintf(stdout, "%s[%s] %s\n", timeStr, GetLogStr(level), msgStr); 00142 //ESP_LOGI(TAG, "%s[%s] %s\n", timeStr, GetLogStr(level), msgStr); 00143 } 00144 00145 00146 /* our default logger */ 00147 void wolfSSH_Log(enum wolfSSH_LogLevel level, const char *const fmt, ...) 00148 { 00149 va_list vlist; 00150 char msgStr[WOLFSSH_DEFAULT_LOG_WIDTH]; 00151 00152 if (level < logLevel) 00153 return; /* don't need to output */ 00154 00155 /* format msg */ 00156 va_start(vlist, fmt); 00157 WVSNPRINTF(msgStr, sizeof(msgStr), fmt, vlist); 00158 va_end(vlist); 00159 00160 if (logFunction) 00161 logFunction(level, msgStr); 00162 }
Generated on Tue Jul 12 2022 21:46:52 by
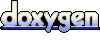