ssh lib
Embed:
(wiki syntax)
Show/hide line numbers
sp.h
00001 /* sp.h 00002 * 00003 * Copyright (C) 2006-2017 wolfSSL Inc. 00004 * 00005 * This file is part of wolfSSL. 00006 * 00007 * wolfSSL is free software; you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation; either version 2 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * wolfSSL is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with this program; if not, write to the Free Software 00019 * Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1335, USA 00020 */ 00021 00022 00023 #ifndef WOLF_CRYPT_SP_H 00024 #define WOLF_CRYPT_SP_H 00025 00026 #include <wolfcrypt/types.h> 00027 00028 #if defined(WOLFSSL_HAVE_SP_RSA) || defined(WOLFSSL_HAVE_SP_DH) || \ 00029 defined(WOLFSSL_HAVE_SP_ECC) 00030 00031 #include <stdint.h> 00032 00033 #include <wolfcrypt/integer.h> 00034 #include <wolfcrypt/sp_int.h> 00035 00036 #include <wolfcrypt/ecc.h> 00037 00038 #if defined(_MSC_VER) 00039 #define SP_NOINLINE __declspec(noinline) 00040 #elif defined(__GNUC__) 00041 #define SP_NOINLINE __attribute__((noinline)) 00042 #else 00043 #define 5P_NOINLINE 00044 #endif 00045 00046 00047 #ifdef __cplusplus 00048 extern "C" { 00049 #endif 00050 00051 #ifdef WOLFSSL_HAVE_SP_RSA 00052 00053 WOLFSSL_LOCAL int sp_RsaPublic_2048(const byte* in, word32 inLen, 00054 mp_int* em, mp_int* mm, byte* out, word32* outLen); 00055 WOLFSSL_LOCAL int sp_RsaPrivate_2048(const byte* in, word32 inLen, 00056 mp_int* dm, mp_int* pm, mp_int* qm, mp_int* dpm, mp_int* dqm, mp_int* qim, 00057 mp_int* mm, byte* out, word32* outLen); 00058 00059 WOLFSSL_LOCAL int sp_RsaPublic_3072(const byte* in, word32 inLen, 00060 mp_int* em, mp_int* mm, byte* out, word32* outLen); 00061 WOLFSSL_LOCAL int sp_RsaPrivate_3072(const byte* in, word32 inLen, 00062 mp_int* dm, mp_int* pm, mp_int* qm, mp_int* dpm, mp_int* dqm, mp_int* qim, 00063 mp_int* mm, byte* out, word32* outLen); 00064 00065 #endif /* WOLFSSL_HAVE_SP_RSA */ 00066 00067 #ifdef WOLFSSL_HAVE_SP_DH 00068 00069 WOLFSSL_LOCAL int sp_ModExp_2048(mp_int* base, mp_int* exp, mp_int* mod, 00070 mp_int* res); 00071 WOLFSSL_LOCAL int sp_ModExp_3072(mp_int* base, mp_int* exp, mp_int* mod, 00072 mp_int* res); 00073 00074 WOLFSSL_LOCAL int sp_DhExp_2048(mp_int* base, const byte* exp, word32 expLen, 00075 mp_int* mod, byte* out, word32* outLen); 00076 WOLFSSL_LOCAL int sp_DhExp_3072(mp_int* base, const byte* exp, word32 expLen, 00077 mp_int* mod, byte* out, word32* outLen); 00078 00079 #endif /* WOLFSSL_HAVE_SP_DH */ 00080 00081 #ifdef WOLFSSL_HAVE_SP_ECC 00082 00083 int sp_ecc_mulmod_256(mp_int* km, ecc_point* gm, ecc_point* rm, int map, 00084 void* heap); 00085 int sp_ecc_mulmod_base_256(mp_int* km, ecc_point* rm, int map, void* heap); 00086 00087 int sp_ecc_make_key_256(WC_RNG* rng, mp_int* priv, ecc_point* pub, void* heap); 00088 int sp_ecc_secret_gen_256(mp_int* priv, ecc_point* pub, byte* out, 00089 word32* outlen, void* heap); 00090 int sp_ecc_sign_256(const byte* hash, word32 hashLen, WC_RNG* rng, mp_int* priv, 00091 mp_int* rm, mp_int* sm, void* heap); 00092 int sp_ecc_verify_256(const byte* hash, word32 hashLen, mp_int* pX, mp_int* pY, 00093 mp_int* pZ, mp_int* r, mp_int* sm, int* res, void* heap); 00094 int sp_ecc_is_point_256(mp_int* pX, mp_int* pY); 00095 int sp_ecc_check_key_256(mp_int* pX, mp_int* pY, mp_int* privm, void* heap); 00096 int sp_ecc_proj_add_point_256(mp_int* pX, mp_int* pY, mp_int* pZ, 00097 mp_int* qX, mp_int* qY, mp_int* qZ, 00098 mp_int* rX, mp_int* rY, mp_int* rZ); 00099 int sp_ecc_proj_dbl_point_256(mp_int* pX, mp_int* pY, mp_int* pZ, 00100 mp_int* rX, mp_int* rY, mp_int* rZ); 00101 int sp_ecc_map_256(mp_int* pX, mp_int* pY, mp_int* pZ); 00102 int sp_ecc_uncompress_256(mp_int* xm, int odd, mp_int* ym); 00103 00104 #endif /*ifdef WOLFSSL_HAVE_SP_ECC */ 00105 00106 00107 #ifdef __cplusplus 00108 } /* extern "C" */ 00109 #endif 00110 00111 #endif /* WOLFSSL_HAVE_SP_RSA || WOLFSSL_HAVE_SP_DH || WOLFSSL_HAVE_SP_ECC */ 00112 00113 #endif /* WOLF_CRYPT_SP_H */ 00114 00115
Generated on Tue Jul 12 2022 16:58:07 by
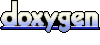