ssh lib
Embed:
(wiki syntax)
Show/hide line numbers
sha512.h
00001 /* sha512.h 00002 * 00003 * Copyright (C) 2006-2018 wolfSSL Inc. 00004 * 00005 * This file is part of wolfSSL. 00006 * 00007 * wolfSSL is free software; you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation; either version 2 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * wolfSSL is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with this program; if not, write to the Free Software 00019 * Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1335, USA 00020 */ 00021 00022 /*! 00023 \file wolfssl/wolfcrypt/sha512.h 00024 */ 00025 00026 00027 #ifndef WOLF_CRYPT_SHA512_H 00028 #define WOLF_CRYPT_SHA512_H 00029 00030 #include <wolfcrypt/types.h> 00031 00032 #if defined(WOLFSSL_SHA512) || defined(WOLFSSL_SHA384) 00033 00034 #if defined(HAVE_FIPS) && \ 00035 defined(HAVE_FIPS_VERSION) && (HAVE_FIPS_VERSION >= 2) 00036 #include <wolfcrypt/fips.h> 00037 #endif /* HAVE_FIPS_VERSION >= 2 */ 00038 00039 #if defined(HAVE_FIPS) && \ 00040 (!defined(HAVE_FIPS_VERSION) || (HAVE_FIPS_VERSION < 2)) 00041 #ifdef WOLFSSL_SHA512 00042 #define wc_Sha512 Sha512 00043 #define WC_SHA512 SHA512 00044 #define WC_SHA512_BLOCK_SIZE SHA512_BLOCK_SIZE 00045 #define WC_SHA512_DIGEST_SIZE SHA512_DIGEST_SIZE 00046 #define WC_SHA512_PAD_SIZE SHA512_PAD_SIZE 00047 #endif /* WOLFSSL_SHA512 */ 00048 #ifdef WOLFSSL_SHA384 00049 #define wc_Sha384 Sha384 00050 #define WC_SHA384 SHA384 00051 #define WC_SHA384_BLOCK_SIZE SHA384_BLOCK_SIZE 00052 #define WC_SHA384_DIGEST_SIZE SHA384_DIGEST_SIZE 00053 #define WC_SHA384_PAD_SIZE SHA384_PAD_SIZE 00054 #endif /* WOLFSSL_SHA384 */ 00055 00056 #define CYASSL_SHA512 00057 #if defined(WOLFSSL_SHA384) 00058 #define CYASSL_SHA384 00059 #endif 00060 /* for fips @wc_fips */ 00061 #include <cyassl/ctaocrypt/sha512.h> 00062 #endif 00063 00064 #ifdef __cplusplus 00065 extern "C" { 00066 #endif 00067 00068 /* avoid redefinition of structs */ 00069 #if !defined(HAVE_FIPS) || \ 00070 (defined(HAVE_FIPS_VERSION) && (HAVE_FIPS_VERSION >= 2)) 00071 00072 #ifdef WOLFSSL_ASYNC_CRYPT 00073 #include <wolfssl/wolfcrypt/async.h> 00074 #endif 00075 00076 #if defined(_MSC_VER) 00077 #define SHA512_NOINLINE __declspec(noinline) 00078 #elif defined(__GNUC__) 00079 #define SHA512_NOINLINE __attribute__((noinline)) 00080 #else 00081 #define SHA512_NOINLINE 00082 #endif 00083 00084 #ifdef WOLFSSL_SHA512 00085 00086 #if !defined(NO_OLD_SHA_NAMES) 00087 #define SHA512 WC_SHA512 00088 #endif 00089 00090 #if !defined(NO_OLD_WC_NAMES) 00091 #define Sha512 wc_Sha512 00092 #define SHA512_BLOCK_SIZE WC_SHA512_BLOCK_SIZE 00093 #define SHA512_DIGEST_SIZE WC_SHA512_DIGEST_SIZE 00094 #define SHA512_PAD_SIZE WC_SHA512_PAD_SIZE 00095 #endif 00096 00097 #endif /* WOLFSSL_SHA512 */ 00098 00099 /* in bytes */ 00100 enum { 00101 #ifdef WOLFSSL_SHA512 00102 WC_SHA512 = WC_HASH_TYPE_SHA512, 00103 #endif 00104 WC_SHA512_BLOCK_SIZE = 128, 00105 WC_SHA512_DIGEST_SIZE = 64, 00106 WC_SHA512_PAD_SIZE = 112 00107 }; 00108 00109 00110 #ifdef WOLFSSL_IMX6_CAAM 00111 #include "wolfcrypt/port/caam/wolfcaam_sha.h" 00112 #else 00113 /* wc_Sha512 digest */ 00114 typedef struct wc_Sha512 { 00115 word64 digest[WC_SHA512_DIGEST_SIZE / sizeof(word64)]; 00116 word64 buffer[WC_SHA512_BLOCK_SIZE / sizeof(word64)]; 00117 word32 buffLen; /* in bytes */ 00118 word64 loLen; /* length in bytes */ 00119 word64 hiLen; /* length in bytes */ 00120 void* heap; 00121 #ifdef USE_INTEL_SPEEDUP 00122 const byte* data; 00123 #endif 00124 #ifdef WOLFSSL_ASYNC_CRYPT 00125 WC_ASYNC_DEV asyncDev; 00126 #endif /* WOLFSSL_ASYNC_CRYPT */ 00127 #ifdef WOLFSSL_SMALL_STACK_CACHE 00128 word64* W; 00129 #endif 00130 } wc_Sha512; 00131 #endif 00132 00133 #endif /* HAVE_FIPS */ 00134 00135 #ifdef WOLFSSL_SHA512 00136 00137 WOLFSSL_API int wc_InitSha512(wc_Sha512*); 00138 WOLFSSL_API int wc_InitSha512_ex(wc_Sha512*, void*, int); 00139 WOLFSSL_API int wc_Sha512Update(wc_Sha512*, const byte*, word32); 00140 WOLFSSL_API int wc_Sha512FinalRaw(wc_Sha512*, byte*); 00141 WOLFSSL_API int wc_Sha512Final(wc_Sha512*, byte*); 00142 WOLFSSL_API void wc_Sha512Free(wc_Sha512*); 00143 00144 WOLFSSL_API int wc_Sha512GetHash(wc_Sha512*, byte*); 00145 WOLFSSL_API int wc_Sha512Copy(wc_Sha512* src, wc_Sha512* dst); 00146 00147 #endif /* WOLFSSL_SHA512 */ 00148 00149 #if defined(WOLFSSL_SHA384) 00150 00151 /* avoid redefinition of structs */ 00152 #if !defined(HAVE_FIPS) || \ 00153 (defined(HAVE_FIPS_VERSION) && (HAVE_FIPS_VERSION >= 2)) 00154 00155 #if !defined(NO_OLD_SHA_NAMES) 00156 #define SHA384 WC_SHA384 00157 #endif 00158 00159 #if !defined(NO_OLD_WC_NAMES) 00160 #define Sha384 wc_Sha384 00161 #define SHA384_BLOCK_SIZE WC_SHA384_BLOCK_SIZE 00162 #define SHA384_DIGEST_SIZE WC_SHA384_DIGEST_SIZE 00163 #define SHA384_PAD_SIZE WC_SHA384_PAD_SIZE 00164 #endif 00165 00166 /* in bytes */ 00167 enum { 00168 WC_SHA384 = WC_HASH_TYPE_SHA384, 00169 WC_SHA384_BLOCK_SIZE = WC_SHA512_BLOCK_SIZE, 00170 WC_SHA384_DIGEST_SIZE = 48, 00171 WC_SHA384_PAD_SIZE = WC_SHA512_PAD_SIZE 00172 }; 00173 00174 00175 typedef wc_Sha512 wc_Sha384; 00176 #endif /* HAVE_FIPS */ 00177 00178 WOLFSSL_API int wc_InitSha384(wc_Sha384*); 00179 WOLFSSL_API int wc_InitSha384_ex(wc_Sha384*, void*, int); 00180 WOLFSSL_API int wc_Sha384Update(wc_Sha384*, const byte*, word32); 00181 WOLFSSL_API int wc_Sha384FinalRaw(wc_Sha384*, byte*); 00182 WOLFSSL_API int wc_Sha384Final(wc_Sha384*, byte*); 00183 WOLFSSL_API void wc_Sha384Free(wc_Sha384*); 00184 00185 WOLFSSL_API int wc_Sha384GetHash(wc_Sha384*, byte*); 00186 WOLFSSL_API int wc_Sha384Copy(wc_Sha384* src, wc_Sha384* dst); 00187 00188 #endif /* WOLFSSL_SHA384 */ 00189 00190 #ifdef __cplusplus 00191 } /* extern "C" */ 00192 #endif 00193 00194 #endif /* WOLFSSL_SHA512 || WOLFSSL_SHA384 */ 00195 #endif /* WOLF_CRYPT_SHA512_H */ 00196 00197
Generated on Tue Jul 12 2022 16:58:07 by
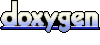