ssh lib
Embed:
(wiki syntax)
Show/hide line numbers
blake2-int.h
00001 /* 00002 BLAKE2 reference source code package - reference C implementations 00003 00004 Written in 2012 by Samuel Neves <sneves@dei.uc.pt> 00005 00006 To the extent possible under law, the author(s) have dedicated all copyright 00007 and related and neighboring rights to this software to the public domain 00008 worldwide. This software is distributed without any warranty. 00009 00010 You should have received a copy of the CC0 Public Domain Dedication along with 00011 this software. If not, see <http://creativecommons.org/publicdomain/zero/1.0/>. 00012 */ 00013 /* blake2-int.h 00014 * 00015 * Copyright (C) 2006-2017 wolfSSL Inc. 00016 * 00017 * This file is part of wolfSSL. 00018 * 00019 * wolfSSL is free software; you can redistribute it and/or modify 00020 * it under the terms of the GNU General Public License as published by 00021 * the Free Software Foundation; either version 2 of the License, or 00022 * (at your option) any later version. 00023 * 00024 * wolfSSL is distributed in the hope that it will be useful, 00025 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00026 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00027 * GNU General Public License for more details. 00028 * 00029 * You should have received a copy of the GNU General Public License 00030 * along with this program; if not, write to the Free Software 00031 * Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1335, USA 00032 */ 00033 00034 00035 00036 00037 #ifndef WOLFCRYPT_BLAKE2_INT_H 00038 #define WOLFCRYPT_BLAKE2_INT_H 00039 00040 #include <wolfcrypt/types.h> 00041 00042 00043 #if defined(_MSC_VER) 00044 #define ALIGN(x) __declspec(align(x)) 00045 #elif defined(__GNUC__) 00046 #define ALIGN(x) __attribute__((aligned(x))) 00047 #else 00048 #define ALIGN(x) 00049 #endif 00050 00051 00052 #if defined(__cplusplus) 00053 extern "C" { 00054 #endif 00055 00056 enum blake2s_constant 00057 { 00058 BLAKE2S_BLOCKBYTES = 64, 00059 BLAKE2S_OUTBYTES = 32, 00060 BLAKE2S_KEYBYTES = 32, 00061 BLAKE2S_SALTBYTES = 8, 00062 BLAKE2S_PERSONALBYTES = 8 00063 }; 00064 00065 enum blake2b_constant 00066 { 00067 BLAKE2B_BLOCKBYTES = 128, 00068 BLAKE2B_OUTBYTES = 64, 00069 BLAKE2B_KEYBYTES = 64, 00070 BLAKE2B_SALTBYTES = 16, 00071 BLAKE2B_PERSONALBYTES = 16 00072 }; 00073 00074 #pragma pack(push, 1) 00075 typedef struct __blake2s_param 00076 { 00077 byte digest_length; /* 1 */ 00078 byte key_length; /* 2 */ 00079 byte fanout; /* 3 */ 00080 byte depth; /* 4 */ 00081 word32 leaf_length; /* 8 */ 00082 byte node_offset[6];/* 14 */ 00083 byte node_depth; /* 15 */ 00084 byte inner_length; /* 16 */ 00085 /* byte reserved[0]; */ 00086 byte salt[BLAKE2B_SALTBYTES]; /* 24 */ 00087 byte personal[BLAKE2S_PERSONALBYTES]; /* 32 */ 00088 } blake2s_param; 00089 00090 ALIGN( 64 ) typedef struct __blake2s_state 00091 { 00092 word32 h[8]; 00093 word32 t[2]; 00094 word32 f[2]; 00095 byte buf[2 * BLAKE2S_BLOCKBYTES]; 00096 word64 buflen; 00097 byte last_node; 00098 } blake2s_state ; 00099 00100 typedef struct __blake2b_param 00101 { 00102 byte digest_length; /* 1 */ 00103 byte key_length; /* 2 */ 00104 byte fanout; /* 3 */ 00105 byte depth; /* 4 */ 00106 word32 leaf_length; /* 8 */ 00107 word64 node_offset; /* 16 */ 00108 byte node_depth; /* 17 */ 00109 byte inner_length; /* 18 */ 00110 byte reserved[14]; /* 32 */ 00111 byte salt[BLAKE2B_SALTBYTES]; /* 48 */ 00112 byte personal[BLAKE2B_PERSONALBYTES]; /* 64 */ 00113 } blake2b_param; 00114 00115 ALIGN( 64 ) typedef struct __blake2b_state 00116 { 00117 word64 h[8]; 00118 word64 t[2]; 00119 word64 f[2]; 00120 byte buf[2 * BLAKE2B_BLOCKBYTES]; 00121 word64 buflen; 00122 byte last_node; 00123 } blake2b_state; 00124 00125 typedef struct __blake2sp_state 00126 { 00127 blake2s_state S[8][1]; 00128 blake2s_state R[1]; 00129 byte buf[8 * BLAKE2S_BLOCKBYTES]; 00130 word64 buflen; 00131 } blake2sp_state; 00132 00133 typedef struct __blake2bp_state 00134 { 00135 blake2b_state S[4][1]; 00136 blake2b_state R[1]; 00137 byte buf[4 * BLAKE2B_BLOCKBYTES]; 00138 word64 buflen; 00139 } blake2bp_state; 00140 #pragma pack(pop) 00141 00142 /* Streaming API */ 00143 int blake2s_init( blake2s_state *S, const byte outlen ); 00144 int blake2s_init_key( blake2s_state *S, const byte outlen, const void *key, const byte keylen ); 00145 int blake2s_init_param( blake2s_state *S, const blake2s_param *P ); 00146 int blake2s_update( blake2s_state *S, const byte *in, word64 inlen ); 00147 int blake2s_final( blake2s_state *S, byte *out, byte outlen ); 00148 00149 int blake2b_init( blake2b_state *S, const byte outlen ); 00150 int blake2b_init_key( blake2b_state *S, const byte outlen, const void *key, const byte keylen ); 00151 int blake2b_init_param( blake2b_state *S, const blake2b_param *P ); 00152 int blake2b_update( blake2b_state *S, const byte *in, word64 inlen ); 00153 int blake2b_final( blake2b_state *S, byte *out, byte outlen ); 00154 00155 int blake2sp_init( blake2sp_state *S, const byte outlen ); 00156 int blake2sp_init_key( blake2sp_state *S, const byte outlen, const void *key, const byte keylen ); 00157 int blake2sp_update( blake2sp_state *S, const byte *in, word64 inlen ); 00158 int blake2sp_final( blake2sp_state *S, byte *out, byte outlen ); 00159 00160 int blake2bp_init( blake2bp_state *S, const byte outlen ); 00161 int blake2bp_init_key( blake2bp_state *S, const byte outlen, const void *key, const byte keylen ); 00162 int blake2bp_update( blake2bp_state *S, const byte *in, word64 inlen ); 00163 int blake2bp_final( blake2bp_state *S, byte *out, byte outlen ); 00164 00165 /* Simple API */ 00166 int blake2s( byte *out, const void *in, const void *key, const byte outlen, const word64 inlen, byte keylen ); 00167 int blake2b( byte *out, const void *in, const void *key, const byte outlen, const word64 inlen, byte keylen ); 00168 00169 int blake2sp( byte *out, const void *in, const void *key, const byte outlen, const word64 inlen, byte keylen ); 00170 int blake2bp( byte *out, const void *in, const void *key, const byte outlen, const word64 inlen, byte keylen ); 00171 00172 static WC_INLINE int blake2( byte *out, const void *in, const void *key, const byte outlen, const word64 inlen, byte keylen ) 00173 { 00174 return blake2b( out, in, key, outlen, inlen, keylen ); 00175 } 00176 00177 00178 00179 #if defined(__cplusplus) 00180 } 00181 #endif 00182 00183 #endif /* WOLFCRYPT_BLAKE2_INT_H */ 00184 00185
Generated on Tue Jul 12 2022 16:58:05 by
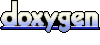