ssh lib
Embed:
(wiki syntax)
Show/hide line numbers
asn.h
00001 /* asn.h 00002 * 00003 * Copyright (C) 2006-2017 wolfSSL Inc. 00004 * 00005 * This file is part of wolfSSL. 00006 * 00007 * wolfSSL is free software; you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation; either version 2 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * wolfSSL is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with this program; if not, write to the Free Software 00019 * Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1335, USA 00020 */ 00021 00022 /*! 00023 \file wolfssl/wolfcrypt/asn.h 00024 */ 00025 00026 #ifndef WOLF_CRYPT_ASN_H 00027 #define WOLF_CRYPT_ASN_H 00028 00029 #include <wolfcrypt/types.h> 00030 00031 #ifndef NO_ASN 00032 00033 00034 #if !defined(NO_ASN_TIME) && defined(NO_TIME_H) 00035 #define NO_ASN_TIME /* backwards compatibility with NO_TIME_H */ 00036 #endif 00037 00038 #include <wolfcrypt/integer.h> 00039 00040 /* fips declare of RsaPrivateKeyDecode @wc_fips */ 00041 #if defined(HAVE_FIPS) && !defined(NO_RSA) && \ 00042 (!defined(HAVE_FIPS_VERSION) || (HAVE_FIPS_VERSION < 2)) 00043 #include <cyassl/ctaocrypt/rsa.h> 00044 #endif 00045 00046 #ifndef NO_DH 00047 #include <wolfcrypt/dh.h> 00048 #endif 00049 #ifndef NO_DSA 00050 #include <wolfcrypt/dsa.h> 00051 #endif 00052 #ifndef NO_SHA 00053 #include <wolfcrypt/sha.h> 00054 #endif 00055 #ifndef NO_MD5 00056 #include <wolfcrypt/md5.h> 00057 #endif 00058 #include <wolfcrypt/sha256.h> 00059 #include <wolfcrypt/asn_public.h> /* public interface */ 00060 00061 00062 #ifdef __cplusplus 00063 extern "C" { 00064 #endif 00065 00066 enum { 00067 ISSUER = 0, 00068 SUBJECT = 1, 00069 00070 EXTERNAL_SERIAL_SIZE = 32, 00071 00072 BEFORE = 0, 00073 AFTER = 1 00074 }; 00075 00076 /* ASN Tags */ 00077 enum ASN_Tags { 00078 ASN_BOOLEAN = 0x01, 00079 ASN_INTEGER = 0x02, 00080 ASN_BIT_STRING = 0x03, 00081 ASN_OCTET_STRING = 0x04, 00082 ASN_TAG_NULL = 0x05, 00083 ASN_OBJECT_ID = 0x06, 00084 ASN_ENUMERATED = 0x0a, 00085 ASN_UTF8STRING = 0x0c, 00086 ASN_SEQUENCE = 0x10, 00087 ASN_SET = 0x11, 00088 ASN_UTC_TIME = 0x17, 00089 ASN_OTHER_TYPE = 0x00, 00090 ASN_RFC822_TYPE = 0x01, 00091 ASN_DNS_TYPE = 0x02, 00092 ASN_DIR_TYPE = 0x04, 00093 ASN_URI_TYPE = 0x06, /* the value 6 is from GeneralName OID */ 00094 ASN_GENERALIZED_TIME = 0x18, 00095 CRL_EXTENSIONS = 0xa0, 00096 ASN_EXTENSIONS = 0xa3, 00097 ASN_LONG_LENGTH = 0x80, 00098 ASN_INDEF_LENGTH = 0x80, 00099 00100 /* ASN_Flags - Bitmask */ 00101 ASN_CONSTRUCTED = 0x20, 00102 ASN_CONTEXT_SPECIFIC = 0x80, 00103 }; 00104 00105 #define ASN_UTC_TIME_SIZE 14 00106 #define ASN_GENERALIZED_TIME_SIZE 16 00107 00108 enum DN_Tags { 00109 ASN_COMMON_NAME = 0x03, /* CN */ 00110 ASN_SUR_NAME = 0x04, /* SN */ 00111 ASN_SERIAL_NUMBER = 0x05, /* serialNumber */ 00112 ASN_COUNTRY_NAME = 0x06, /* C */ 00113 ASN_LOCALITY_NAME = 0x07, /* L */ 00114 ASN_STATE_NAME = 0x08, /* ST */ 00115 ASN_ORG_NAME = 0x0a, /* O */ 00116 ASN_ORGUNIT_NAME = 0x0b, /* OU */ 00117 ASN_EMAIL_NAME = 0x98, /* not oid number there is 97 in 2.5.4.0-97 */ 00118 00119 /* pilot attribute types 00120 * OID values of 0.9.2342.19200300.100.1.* */ 00121 ASN_USER_ID = 0x01, /* UID */ 00122 ASN_DOMAIN_COMPONENT = 0x19 /* DC */ 00123 }; 00124 00125 /* DN Tag Strings */ 00126 #define WOLFSSL_COMMON_NAME "/CN=" 00127 #define WOLFSSL_SUR_NAME "/SN=" 00128 #define WOLFSSL_SERIAL_NUMBER "/serialNumber=" 00129 #define WOLFSSL_COUNTRY_NAME "/C=" 00130 #define WOLFSSL_LOCALITY_NAME "/L=" 00131 #define WOLFSSL_STATE_NAME "/ST=" 00132 #define WOLFSSL_ORG_NAME "/O=" 00133 #define WOLFSSL_ORGUNIT_NAME "/OU=" 00134 #define WOLFSSL_DOMAIN_COMPONENT "/DC=" 00135 00136 enum ECC_TYPES { 00137 ECC_PREFIX_0 = 160, 00138 ECC_PREFIX_1 = 161 00139 }; 00140 00141 enum Misc_ASN { 00142 ASN_NAME_MAX = 256, 00143 MAX_SALT_SIZE = 64, /* MAX PKCS Salt length */ 00144 MAX_IV_SIZE = 64, /* MAX PKCS Iv length */ 00145 ASN_BOOL_SIZE = 2, /* including type */ 00146 ASN_ECC_HEADER_SZ = 2, /* String type + 1 byte len */ 00147 ASN_ECC_CONTEXT_SZ = 2, /* Content specific type + 1 byte len */ 00148 #ifdef NO_SHA 00149 KEYID_SIZE = WC_SHA256_DIGEST_SIZE, 00150 #else 00151 KEYID_SIZE = WC_SHA_DIGEST_SIZE, 00152 #endif 00153 RSA_INTS = 8, /* RSA ints in private key */ 00154 DSA_INTS = 5, /* DSA ints in private key */ 00155 MIN_DATE_SIZE = 13, 00156 MAX_DATE_SIZE = 32, 00157 ASN_GEN_TIME_SZ = 15, /* 7 numbers * 2 + Zulu tag */ 00158 MAX_ENCODED_SIG_SZ = 512, 00159 MAX_SIG_SZ = 256, 00160 MAX_ALGO_SZ = 20, 00161 MAX_SHORT_SZ = 6, /* asn int + byte len + 4 byte length */ 00162 MAX_SEQ_SZ = 5, /* enum(seq | con) + length(4) */ 00163 MAX_SET_SZ = 5, /* enum(set | con) + length(4) */ 00164 MAX_OCTET_STR_SZ = 5, /* enum(set | con) + length(4) */ 00165 MAX_EXP_SZ = 5, /* enum(contextspec|con|exp) + length(4) */ 00166 MAX_PRSTR_SZ = 5, /* enum(prstr) + length(4) */ 00167 MAX_VERSION_SZ = 5, /* enum + id + version(byte) + (header(2))*/ 00168 MAX_ENCODED_DIG_SZ = 73, /* sha512 + enum(bit or octet) + length(4) */ 00169 MAX_RSA_INT_SZ = 517, /* RSA raw sz 4096 for bits + tag + len(4) */ 00170 MAX_NTRU_KEY_SZ = 610, /* NTRU 112 bit public key */ 00171 MAX_NTRU_ENC_SZ = 628, /* NTRU 112 bit DER public encoding */ 00172 MAX_LENGTH_SZ = 4, /* Max length size for DER encoding */ 00173 MAX_RSA_E_SZ = 16, /* Max RSA public e size */ 00174 MAX_CA_SZ = 32, /* Max encoded CA basic constraint length */ 00175 MAX_SN_SZ = 35, /* Max encoded serial number (INT) length */ 00176 MAX_DER_DIGEST_SZ = MAX_ENCODED_DIG_SZ + MAX_ALGO_SZ + MAX_SEQ_SZ, /* Maximum DER digest size */ 00177 #ifdef WOLFSSL_CERT_GEN 00178 #ifdef WOLFSSL_CERT_REQ 00179 /* Max encoded cert req attributes length */ 00180 MAX_ATTRIB_SZ = MAX_SEQ_SZ * 3 + (11 + MAX_SEQ_SZ) * 2 + 00181 MAX_PRSTR_SZ + CTC_NAME_SIZE, /* 11 is the OID size */ 00182 #endif 00183 #if defined(WOLFSSL_ALT_NAMES) || defined(WOLFSSL_CERT_EXT) 00184 MAX_EXTENSIONS_SZ = 1 + MAX_LENGTH_SZ + CTC_MAX_ALT_SIZE, 00185 #else 00186 MAX_EXTENSIONS_SZ = 1 + MAX_LENGTH_SZ + MAX_CA_SZ, 00187 #endif 00188 /* Max total extensions, id + len + others */ 00189 #endif 00190 #if defined(WOLFSSL_CERT_EXT) || defined(OPENSSL_EXTRA) 00191 MAX_OID_SZ = 32, /* Max DER length of OID*/ 00192 MAX_OID_STRING_SZ = 64, /* Max string length representation of OID*/ 00193 #endif 00194 #ifdef WOLFSSL_CERT_EXT 00195 MAX_KID_SZ = 45, /* Max encoded KID length (SHA-256 case) */ 00196 MAX_KEYUSAGE_SZ = 18, /* Max encoded Key Usage length */ 00197 MAX_EXTKEYUSAGE_SZ = 12 + (6 * (8 + 2)) + 00198 CTC_MAX_EKU_OID_SZ, /* Max encoded ExtKeyUsage 00199 (SEQ/LEN + OBJID + OCTSTR/LEN + SEQ + (6 * (SEQ + OID))) */ 00200 MAX_CERTPOL_NB = CTC_MAX_CERTPOL_NB,/* Max number of Cert Policy */ 00201 MAX_CERTPOL_SZ = CTC_MAX_CERTPOL_SZ, 00202 #endif 00203 MAX_NAME_ENTRIES = 5, /* extra entries added to x509 name struct */ 00204 OCSP_NONCE_EXT_SZ = 35, /* OCSP Nonce Extension size */ 00205 MAX_OCSP_EXT_SZ = 58, /* Max OCSP Extension length */ 00206 MAX_OCSP_NONCE_SZ = 16, /* OCSP Nonce size */ 00207 EIGHTK_BUF = 8192, /* Tmp buffer size */ 00208 MAX_PUBLIC_KEY_SZ = MAX_NTRU_ENC_SZ + MAX_ALGO_SZ + MAX_SEQ_SZ * 2, 00209 /* use bigger NTRU size */ 00210 #ifdef WOLFSSL_ENCRYPTED_KEYS 00211 HEADER_ENCRYPTED_KEY_SIZE = 88,/* Extra header size for encrypted key */ 00212 #else 00213 HEADER_ENCRYPTED_KEY_SIZE = 0, 00214 #endif 00215 TRAILING_ZERO = 1, /* Used for size of zero pad */ 00216 MIN_VERSION_SZ = 3, /* Min bytes needed for GetMyVersion */ 00217 #if defined(OPENSSL_ALL) || defined(WOLFSSL_MYSQL_COMPATIBLE) || defined(WOLFSSL_NGINX) || \ 00218 defined(WOLFSSL_HAPROXY) || defined(OPENSSL_EXTRA) 00219 MAX_TIME_STRING_SZ = 25, /* Max length of formatted time string */ 00220 #endif 00221 00222 PKCS5_SALT_SZ = 8, 00223 00224 PEM_LINE_LEN = 80, /* PEM line max + fudge */ 00225 }; 00226 00227 00228 enum Oid_Types { 00229 oidHashType = 0, 00230 oidSigType = 1, 00231 oidKeyType = 2, 00232 oidCurveType = 3, 00233 oidBlkType = 4, 00234 oidOcspType = 5, 00235 oidCertExtType = 6, 00236 oidCertAuthInfoType = 7, 00237 oidCertPolicyType = 8, 00238 oidCertAltNameType = 9, 00239 oidCertKeyUseType = 10, 00240 oidKdfType = 11, 00241 oidKeyWrapType = 12, 00242 oidCmsKeyAgreeType = 13, 00243 oidPBEType = 14, 00244 oidHmacType = 15, 00245 oidIgnoreType 00246 }; 00247 00248 00249 enum Hash_Sum { 00250 MD2h = 646, 00251 MD5h = 649, 00252 SHAh = 88, 00253 SHA224h = 417, 00254 SHA256h = 414, 00255 SHA384h = 415, 00256 SHA512h = 416 00257 }; 00258 00259 00260 #if !defined(NO_DES3) || !defined(NO_AES) 00261 enum Block_Sum { 00262 #ifdef WOLFSSL_AES_128 00263 AES128CBCb = 414, 00264 #endif 00265 #ifdef WOLFSSL_AES_192 00266 AES192CBCb = 434, 00267 #endif 00268 #ifdef WOLFSSL_AES_256 00269 AES256CBCb = 454, 00270 #endif 00271 #ifndef NO_DES3 00272 DESb = 69, 00273 DES3b = 652 00274 #endif 00275 }; 00276 #endif /* !NO_DES3 || !NO_AES */ 00277 00278 00279 enum Key_Sum { 00280 DSAk = 515, 00281 RSAk = 645, 00282 NTRUk = 274, 00283 ECDSAk = 518, 00284 ED25519k = 256 00285 }; 00286 00287 00288 #ifndef NO_AES 00289 enum KeyWrap_Sum { 00290 #ifdef WOLFSSL_AES_128 00291 AES128_WRAP = 417, 00292 #endif 00293 #ifdef WOLFSSL_AES_192 00294 AES192_WRAP = 437, 00295 #endif 00296 #ifdef WOLFSSL_AES_256 00297 AES256_WRAP = 457 00298 #endif 00299 }; 00300 #endif /* !NO_AES */ 00301 00302 enum Key_Agree { 00303 dhSinglePass_stdDH_sha1kdf_scheme = 464, 00304 dhSinglePass_stdDH_sha224kdf_scheme = 188, 00305 dhSinglePass_stdDH_sha256kdf_scheme = 189, 00306 dhSinglePass_stdDH_sha384kdf_scheme = 190, 00307 dhSinglePass_stdDH_sha512kdf_scheme = 191, 00308 }; 00309 00310 00311 enum Ecc_Sum { 00312 ECC_SECP112R1_OID = 182, 00313 ECC_SECP112R2_OID = 183, 00314 ECC_SECP128R1_OID = 204, 00315 ECC_SECP128R2_OID = 205, 00316 ECC_SECP160R1_OID = 184, 00317 ECC_SECP160R2_OID = 206, 00318 ECC_SECP160K1_OID = 185, 00319 ECC_BRAINPOOLP160R1_OID = 98, 00320 ECC_SECP192R1_OID = 520, 00321 ECC_PRIME192V2_OID = 521, 00322 ECC_PRIME192V3_OID = 522, 00323 ECC_SECP192K1_OID = 207, 00324 ECC_BRAINPOOLP192R1_OID = 100, 00325 ECC_SECP224R1_OID = 209, 00326 ECC_SECP224K1_OID = 208, 00327 ECC_BRAINPOOLP224R1_OID = 102, 00328 ECC_PRIME239V1_OID = 523, 00329 ECC_PRIME239V2_OID = 524, 00330 ECC_PRIME239V3_OID = 525, 00331 ECC_SECP256R1_OID = 526, 00332 ECC_SECP256K1_OID = 186, 00333 ECC_BRAINPOOLP256R1_OID = 104, 00334 ECC_X25519_OID = 365, 00335 ECC_ED25519_OID = 256, 00336 ECC_BRAINPOOLP320R1_OID = 106, 00337 ECC_SECP384R1_OID = 210, 00338 ECC_BRAINPOOLP384R1_OID = 108, 00339 ECC_BRAINPOOLP512R1_OID = 110, 00340 ECC_SECP521R1_OID = 211, 00341 }; 00342 00343 00344 enum KDF_Sum { 00345 PBKDF2_OID = 660 00346 }; 00347 00348 00349 enum HMAC_Sum { 00350 HMAC_SHA224_OID = 652, 00351 HMAC_SHA256_OID = 653, 00352 HMAC_SHA384_OID = 654, 00353 HMAC_SHA512_OID = 655 00354 }; 00355 00356 00357 enum Extensions_Sum { 00358 BASIC_CA_OID = 133, 00359 ALT_NAMES_OID = 131, 00360 CRL_DIST_OID = 145, 00361 AUTH_INFO_OID = 69, /* id-pe 1 */ 00362 AUTH_KEY_OID = 149, 00363 SUBJ_KEY_OID = 128, 00364 CERT_POLICY_OID = 146, 00365 KEY_USAGE_OID = 129, /* 2.5.29.15 */ 00366 INHIBIT_ANY_OID = 168, /* 2.5.29.54 */ 00367 EXT_KEY_USAGE_OID = 151, /* 2.5.29.37 */ 00368 NAME_CONS_OID = 144, /* 2.5.29.30 */ 00369 PRIV_KEY_USAGE_PERIOD_OID = 130, /* 2.5.29.16 */ 00370 SUBJECT_INFO_ACCESS = 79, /* id-pe 11 */ 00371 POLICY_MAP_OID = 147, 00372 POLICY_CONST_OID = 150, 00373 ISSUE_ALT_NAMES_OID = 132, 00374 TLS_FEATURE_OID = 92 /* id-pe 24 */ 00375 }; 00376 00377 enum CertificatePolicy_Sum { 00378 CP_ANY_OID = 146 /* id-ce 32 0 */ 00379 }; 00380 00381 enum SepHardwareName_Sum { 00382 HW_NAME_OID = 79 /* 1.3.6.1.5.5.7.8.4 from RFC 4108*/ 00383 }; 00384 00385 enum AuthInfo_Sum { 00386 AIA_OCSP_OID = 116, /* 1.3.6.1.5.5.7.48.1 */ 00387 AIA_CA_ISSUER_OID = 117 /* 1.3.6.1.5.5.7.48.2 */ 00388 }; 00389 00390 enum ExtKeyUsage_Sum { /* From RFC 5280 */ 00391 EKU_ANY_OID = 151, /* 2.5.29.37.0, anyExtendedKeyUsage */ 00392 EKU_SERVER_AUTH_OID = 71, /* 1.3.6.1.5.5.7.3.1, id-kp-serverAuth */ 00393 EKU_CLIENT_AUTH_OID = 72, /* 1.3.6.1.5.5.7.3.2, id-kp-clientAuth */ 00394 EKU_CODESIGNING_OID = 73, /* 1.3.6.1.5.5.7.3.3, id-kp-codeSigning */ 00395 EKU_EMAILPROTECT_OID = 74, /* 1.3.6.1.5.5.7.3.4, id-kp-emailProtection */ 00396 EKU_TIMESTAMP_OID = 78, /* 1.3.6.1.5.5.7.3.8, id-kp-timeStamping */ 00397 EKU_OCSP_SIGN_OID = 79 /* 1.3.6.1.5.5.7.3.9, id-kp-OCSPSigning */ 00398 }; 00399 00400 00401 enum VerifyType { 00402 NO_VERIFY = 0, 00403 VERIFY = 1, 00404 VERIFY_CRL = 2, 00405 VERIFY_OCSP = 3 00406 }; 00407 00408 #ifdef WOLFSSL_CERT_EXT 00409 enum KeyIdType { 00410 SKID_TYPE = 0, 00411 AKID_TYPE = 1 00412 }; 00413 #endif 00414 00415 /* Key usage extension bits (based on RFC 5280) */ 00416 #define KEYUSE_DIGITAL_SIG 0x0080 00417 #define KEYUSE_CONTENT_COMMIT 0x0040 00418 #define KEYUSE_KEY_ENCIPHER 0x0020 00419 #define KEYUSE_DATA_ENCIPHER 0x0010 00420 #define KEYUSE_KEY_AGREE 0x0008 00421 #define KEYUSE_KEY_CERT_SIGN 0x0004 00422 #define KEYUSE_CRL_SIGN 0x0002 00423 #define KEYUSE_ENCIPHER_ONLY 0x0001 00424 #define KEYUSE_DECIPHER_ONLY 0x8000 00425 00426 /* Extended Key Usage bits (internal mapping only) */ 00427 #define EXTKEYUSE_USER 0x80 00428 #define EXTKEYUSE_OCSP_SIGN 0x40 00429 #define EXTKEYUSE_TIMESTAMP 0x20 00430 #define EXTKEYUSE_EMAILPROT 0x10 00431 #define EXTKEYUSE_CODESIGN 0x08 00432 #define EXTKEYUSE_CLIENT_AUTH 0x04 00433 #define EXTKEYUSE_SERVER_AUTH 0x02 00434 #define EXTKEYUSE_ANY 0x01 00435 00436 typedef struct DNS_entry DNS_entry; 00437 00438 struct DNS_entry { 00439 DNS_entry* next; /* next on DNS list */ 00440 int type; /* i.e. ASN_DNS_TYPE */ 00441 int len; /* actual DNS len */ 00442 char* name; /* actual DNS name */ 00443 }; 00444 00445 00446 typedef struct Base_entry Base_entry; 00447 00448 struct Base_entry { 00449 Base_entry* next; /* next on name base list */ 00450 char* name; /* actual name base */ 00451 int nameSz; /* name length */ 00452 byte type; /* Name base type (DNS or RFC822) */ 00453 }; 00454 00455 #define DOMAIN_COMPONENT_MAX 10 00456 00457 struct DecodedName { 00458 char* fullName; 00459 int fullNameLen; 00460 int entryCount; 00461 int cnIdx; 00462 int cnLen; 00463 int snIdx; 00464 int snLen; 00465 int cIdx; 00466 int cLen; 00467 int lIdx; 00468 int lLen; 00469 int stIdx; 00470 int stLen; 00471 int oIdx; 00472 int oLen; 00473 int ouIdx; 00474 int ouLen; 00475 int emailIdx; 00476 int emailLen; 00477 int uidIdx; 00478 int uidLen; 00479 int serialIdx; 00480 int serialLen; 00481 int dcIdx[DOMAIN_COMPONENT_MAX]; 00482 int dcLen[DOMAIN_COMPONENT_MAX]; 00483 int dcNum; 00484 int dcMode; 00485 }; 00486 00487 enum SignatureState { 00488 SIG_STATE_BEGIN, 00489 SIG_STATE_HASH, 00490 SIG_STATE_KEY, 00491 SIG_STATE_DO, 00492 SIG_STATE_CHECK, 00493 }; 00494 00495 00496 #ifdef HAVE_PK_CALLBACKS 00497 #ifdef HAVE_ECC 00498 typedef int (*wc_CallbackEccVerify)( 00499 const unsigned char* sig, unsigned int sigSz, 00500 const unsigned char* hash, unsigned int hashSz, 00501 const unsigned char* keyDer, unsigned int keySz, 00502 int* result, void* ctx); 00503 #endif 00504 #ifndef NO_RSA 00505 typedef int (*wc_CallbackRsaVerify)( 00506 unsigned char* sig, unsigned int sigSz, 00507 unsigned char** out, 00508 const unsigned char* keyDer, unsigned int keySz, 00509 void* ctx); 00510 #endif 00511 #endif /* HAVE_PK_CALLBACKS */ 00512 00513 struct SignatureCtx { 00514 void* heap; 00515 byte* digest; 00516 #ifndef NO_RSA 00517 byte* out; 00518 byte* plain; 00519 #endif 00520 #if defined(HAVE_ECC) || defined(HAVE_ED25519) 00521 int verify; 00522 #endif 00523 union { 00524 #ifndef NO_RSA 00525 struct RsaKey* rsa; 00526 #endif 00527 #ifdef HAVE_ECC 00528 struct ecc_key* ecc; 00529 #endif 00530 #ifdef HAVE_ED25519 00531 struct ed25519_key* ed25519; 00532 #endif 00533 void* ptr; 00534 } key; 00535 int devId; 00536 int state; 00537 int typeH; 00538 int digestSz; 00539 word32 keyOID; 00540 #ifdef WOLFSSL_ASYNC_CRYPT 00541 WC_ASYNC_DEV* asyncDev; 00542 void* asyncCtx; 00543 #endif 00544 00545 #ifdef HAVE_PK_CALLBACKS 00546 #ifdef HAVE_ECC 00547 wc_CallbackEccVerify pkCbEcc; 00548 void* pkCtxEcc; 00549 #endif 00550 #ifndef NO_RSA 00551 wc_CallbackRsaVerify pkCbRsa; 00552 void* pkCtxRsa; 00553 #endif 00554 #endif /* HAVE_PK_CALLBACKS */ 00555 }; 00556 00557 enum CertSignState { 00558 CERTSIGN_STATE_BEGIN, 00559 CERTSIGN_STATE_DIGEST, 00560 CERTSIGN_STATE_ENCODE, 00561 CERTSIGN_STATE_DO, 00562 }; 00563 00564 struct CertSignCtx { 00565 byte* sig; 00566 byte* digest; 00567 #ifndef NO_RSA 00568 byte* encSig; 00569 int encSigSz; 00570 #endif 00571 int state; /* enum CertSignState */ 00572 }; 00573 00574 00575 typedef struct DecodedCert DecodedCert; 00576 typedef struct DecodedName DecodedName; 00577 typedef struct Signer Signer; 00578 #ifdef WOLFSSL_TRUST_PEER_CERT 00579 typedef struct TrustedPeerCert TrustedPeerCert; 00580 #endif /* WOLFSSL_TRUST_PEER_CERT */ 00581 typedef struct SignatureCtx SignatureCtx; 00582 typedef struct CertSignCtx CertSignCtx; 00583 00584 00585 struct DecodedCert { 00586 byte* publicKey; 00587 word32 pubKeySize; 00588 int pubKeyStored; 00589 word32 certBegin; /* offset to start of cert */ 00590 word32 sigIndex; /* offset to start of signature */ 00591 word32 sigLength; /* length of signature */ 00592 word32 signatureOID; /* sum of algorithm object id */ 00593 word32 keyOID; /* sum of key algo object id */ 00594 int version; /* cert version, 1 or 3 */ 00595 DNS_entry* altNames; /* alt names list of dns entries */ 00596 #ifndef IGNORE_NAME_CONSTRAINTS 00597 DNS_entry* altEmailNames; /* alt names list of RFC822 entries */ 00598 Base_entry* permittedNames; /* Permitted name bases */ 00599 Base_entry* excludedNames; /* Excluded name bases */ 00600 #endif /* IGNORE_NAME_CONSTRAINTS */ 00601 byte subjectHash[KEYID_SIZE]; /* hash of all Names */ 00602 byte issuerHash[KEYID_SIZE]; /* hash of all Names */ 00603 #ifdef HAVE_OCSP 00604 byte issuerKeyHash[KEYID_SIZE]; /* hash of the public Key */ 00605 #endif /* HAVE_OCSP */ 00606 byte* signature; /* not owned, points into raw cert */ 00607 char* subjectCN; /* CommonName */ 00608 int subjectCNLen; /* CommonName Length */ 00609 char subjectCNEnc; /* CommonName Encoding */ 00610 char issuer[ASN_NAME_MAX]; /* full name including common name */ 00611 char subject[ASN_NAME_MAX]; /* full name including common name */ 00612 int verify; /* Default to yes, but could be off */ 00613 byte* source; /* byte buffer holder cert, NOT owner */ 00614 word32 srcIdx; /* current offset into buffer */ 00615 word32 maxIdx; /* max offset based on init size */ 00616 void* heap; /* for user memory overrides */ 00617 byte serial[EXTERNAL_SERIAL_SIZE]; /* raw serial number */ 00618 int serialSz; /* raw serial bytes stored */ 00619 byte* extensions; /* not owned, points into raw cert */ 00620 int extensionsSz; /* length of cert extensions */ 00621 word32 extensionsIdx; /* if want to go back and parse later */ 00622 byte* extAuthInfo; /* Authority Information Access URI */ 00623 int extAuthInfoSz; /* length of the URI */ 00624 byte* extCrlInfo; /* CRL Distribution Points */ 00625 int extCrlInfoSz; /* length of the URI */ 00626 byte extSubjKeyId[KEYID_SIZE]; /* Subject Key ID */ 00627 byte extAuthKeyId[KEYID_SIZE]; /* Authority Key ID */ 00628 byte pathLength; /* CA basic constraint path length */ 00629 word16 extKeyUsage; /* Key usage bitfield */ 00630 byte extExtKeyUsage; /* Extended Key usage bitfield */ 00631 00632 #if defined(OPENSSL_EXTRA) || defined(OPENSSL_EXTRA_X509_SMALL) 00633 byte* extExtKeyUsageSrc; 00634 word32 extExtKeyUsageSz; 00635 word32 extExtKeyUsageCount; 00636 byte* extAuthKeyIdSrc; 00637 word32 extAuthKeyIdSz; 00638 byte* extSubjKeyIdSrc; 00639 word32 extSubjKeyIdSz; 00640 #endif 00641 00642 #if defined(HAVE_ECC) || defined(HAVE_ED25519) 00643 word32 pkCurveOID; /* Public Key's curve OID */ 00644 #endif /* HAVE_ECC */ 00645 byte* beforeDate; 00646 int beforeDateLen; 00647 byte* afterDate; 00648 int afterDateLen; 00649 #ifdef HAVE_PKCS7 00650 byte* issuerRaw; /* pointer to issuer inside source */ 00651 int issuerRawLen; 00652 #endif 00653 #ifndef IGNORE_NAME_CONSTRAINT 00654 byte* subjectRaw; /* pointer to subject inside source */ 00655 int subjectRawLen; 00656 #endif 00657 #ifdef WOLFSSL_CERT_GEN 00658 /* easy access to subject info for other sign */ 00659 char* subjectSN; 00660 int subjectSNLen; 00661 char subjectSNEnc; 00662 char* subjectC; 00663 int subjectCLen; 00664 char subjectCEnc; 00665 char* subjectL; 00666 int subjectLLen; 00667 char subjectLEnc; 00668 char* subjectST; 00669 int subjectSTLen; 00670 char subjectSTEnc; 00671 char* subjectO; 00672 int subjectOLen; 00673 char subjectOEnc; 00674 char* subjectOU; 00675 int subjectOULen; 00676 char subjectOUEnc; 00677 char* subjectEmail; 00678 int subjectEmailLen; 00679 #endif /* WOLFSSL_CERT_GEN */ 00680 #if defined(OPENSSL_EXTRA) || defined(OPENSSL_EXTRA_X509_SMALL) 00681 DecodedName issuerName; 00682 DecodedName subjectName; 00683 #endif /* OPENSSL_EXTRA */ 00684 #ifdef WOLFSSL_SEP 00685 int deviceTypeSz; 00686 byte* deviceType; 00687 int hwTypeSz; 00688 byte* hwType; 00689 int hwSerialNumSz; 00690 byte* hwSerialNum; 00691 #endif /* WOLFSSL_SEP */ 00692 #ifdef WOLFSSL_CERT_EXT 00693 char extCertPolicies[MAX_CERTPOL_NB][MAX_CERTPOL_SZ]; 00694 int extCertPoliciesNb; 00695 #endif /* WOLFSSL_CERT_EXT */ 00696 00697 Signer* ca; 00698 SignatureCtx sigCtx; 00699 00700 /* Option Bits */ 00701 byte subjectCNStored : 1; /* have we saved a copy we own */ 00702 byte extSubjKeyIdSet : 1; /* Set when the SKID was read from cert */ 00703 byte extAuthKeyIdSet : 1; /* Set when the AKID was read from cert */ 00704 #ifndef IGNORE_NAME_CONSTRAINTS 00705 byte extNameConstraintSet : 1; 00706 #endif 00707 byte isCA : 1; /* CA basic constraint true */ 00708 byte pathLengthSet : 1; /* CA basic const path length set */ 00709 byte weOwnAltNames : 1; /* altNames haven't been given to copy */ 00710 byte extKeyUsageSet : 1; 00711 byte extExtKeyUsageSet : 1; /* Extended Key Usage set */ 00712 byte extCRLdistSet : 1; 00713 byte extAuthInfoSet : 1; 00714 byte extBasicConstSet : 1; 00715 byte extSubjAltNameSet : 1; 00716 byte inhibitAnyOidSet : 1; 00717 #ifdef WOLFSSL_SEP 00718 byte extCertPolicySet : 1; 00719 #endif 00720 #if defined(OPENSSL_EXTRA) || defined(OPENSSL_EXTRA_X509_SMALL) 00721 byte extCRLdistCrit : 1; 00722 byte extAuthInfoCrit : 1; 00723 byte extBasicConstCrit : 1; 00724 byte extSubjAltNameCrit : 1; 00725 byte extAuthKeyIdCrit : 1; 00726 #ifndef IGNORE_NAME_CONSTRAINTS 00727 byte extNameConstraintCrit : 1; 00728 #endif 00729 byte extSubjKeyIdCrit : 1; 00730 byte extKeyUsageCrit : 1; 00731 byte extExtKeyUsageCrit : 1; 00732 #endif /* OPENSSL_EXTRA */ 00733 #ifdef WOLFSSL_SEP 00734 byte extCertPolicyCrit : 1; 00735 #endif 00736 00737 }; 00738 00739 00740 #ifdef NO_SHA 00741 #define SIGNER_DIGEST_SIZE WC_SHA256_DIGEST_SIZE 00742 #else 00743 #define SIGNER_DIGEST_SIZE WC_SHA_DIGEST_SIZE 00744 #endif 00745 00746 /* CA Signers */ 00747 /* if change layout change PERSIST_CERT_CACHE functions too */ 00748 struct Signer { 00749 word32 pubKeySize; 00750 word32 keyOID; /* key type */ 00751 word16 keyUsage; 00752 byte pathLength; 00753 byte pathLengthSet; 00754 byte* publicKey; 00755 int nameLen; 00756 char* name; /* common name */ 00757 #ifndef IGNORE_NAME_CONSTRAINTS 00758 Base_entry* permittedNames; 00759 Base_entry* excludedNames; 00760 #endif /* IGNORE_NAME_CONSTRAINTS */ 00761 byte subjectNameHash[SIGNER_DIGEST_SIZE]; 00762 /* sha hash of names in certificate */ 00763 #ifndef NO_SKID 00764 byte subjectKeyIdHash[SIGNER_DIGEST_SIZE]; 00765 /* sha hash of names in certificate */ 00766 #endif 00767 #ifdef WOLFSSL_SIGNER_DER_CERT 00768 DerBuffer* derCert; 00769 #endif 00770 Signer* next; 00771 }; 00772 00773 00774 #ifdef WOLFSSL_TRUST_PEER_CERT 00775 /* used for having trusted peer certs rather then CA */ 00776 struct TrustedPeerCert { 00777 int nameLen; 00778 char* name; /* common name */ 00779 #ifndef IGNORE_NAME_CONSTRAINTS 00780 Base_entry* permittedNames; 00781 Base_entry* excludedNames; 00782 #endif /* IGNORE_NAME_CONSTRAINTS */ 00783 byte subjectNameHash[SIGNER_DIGEST_SIZE]; 00784 /* sha hash of names in certificate */ 00785 #ifndef NO_SKID 00786 byte subjectKeyIdHash[SIGNER_DIGEST_SIZE]; 00787 /* sha hash of names in certificate */ 00788 #endif 00789 word32 sigLen; 00790 byte* sig; 00791 struct TrustedPeerCert* next; 00792 }; 00793 #endif /* WOLFSSL_TRUST_PEER_CERT */ 00794 00795 00796 /* for testing or custom openssl wrappers */ 00797 #if defined(WOLFSSL_TEST_CERT) || defined(OPENSSL_EXTRA) || \ 00798 defined(OPENSSL_EXTRA_X509_SMALL) 00799 #define WOLFSSL_ASN_API WOLFSSL_API 00800 #else 00801 #define WOLFSSL_ASN_API WOLFSSL_LOCAL 00802 #endif 00803 00804 WOLFSSL_ASN_API int wc_BerToDer(const byte* ber, word32 berSz, byte* der, 00805 word32* derSz); 00806 00807 WOLFSSL_ASN_API void FreeAltNames(DNS_entry*, void*); 00808 #ifndef IGNORE_NAME_CONSTRAINTS 00809 WOLFSSL_ASN_API void FreeNameSubtrees(Base_entry*, void*); 00810 #endif /* IGNORE_NAME_CONSTRAINTS */ 00811 WOLFSSL_ASN_API void InitDecodedCert(DecodedCert*, byte*, word32, void*); 00812 WOLFSSL_ASN_API void FreeDecodedCert(DecodedCert*); 00813 WOLFSSL_ASN_API int ParseCert(DecodedCert*, int type, int verify, void* cm); 00814 00815 WOLFSSL_LOCAL int DecodePolicyOID(char *o, word32 oSz, byte *in, word32 inSz); 00816 WOLFSSL_LOCAL int ParseCertRelative(DecodedCert*,int type,int verify,void* cm); 00817 WOLFSSL_LOCAL int DecodeToKey(DecodedCert*, int verify); 00818 00819 WOLFSSL_LOCAL const byte* OidFromId(word32 id, word32 type, word32* oidSz); 00820 WOLFSSL_LOCAL Signer* MakeSigner(void*); 00821 WOLFSSL_LOCAL void FreeSigner(Signer*, void*); 00822 WOLFSSL_LOCAL void FreeSignerTable(Signer**, int, void*); 00823 #ifdef WOLFSSL_TRUST_PEER_CERT 00824 WOLFSSL_LOCAL void FreeTrustedPeer(TrustedPeerCert*, void*); 00825 WOLFSSL_LOCAL void FreeTrustedPeerTable(TrustedPeerCert**, int, void*); 00826 #endif /* WOLFSSL_TRUST_PEER_CERT */ 00827 00828 WOLFSSL_ASN_API int ToTraditional(byte* buffer, word32 length); 00829 WOLFSSL_LOCAL int ToTraditionalInline(const byte* input, word32* inOutIdx, 00830 word32 length); 00831 WOLFSSL_LOCAL int ToTraditionalEnc(byte* buffer, word32 length,const char*,int); 00832 WOLFSSL_ASN_API int UnTraditionalEnc(byte* key, word32 keySz, byte* out, 00833 word32* outSz, const char* password, int passwordSz, int vPKCS, 00834 int vAlgo, byte* salt, word32 saltSz, int itt, WC_RNG* rng, void* heap); 00835 WOLFSSL_LOCAL int DecryptContent(byte* input, word32 sz,const char* psw,int pswSz); 00836 WOLFSSL_LOCAL int EncryptContent(byte* input, word32 sz, byte* out, word32* outSz, 00837 const char* password,int passwordSz, int vPKCS, int vAlgo, 00838 byte* salt, word32 saltSz, int itt, WC_RNG* rng, void* heap); 00839 WOLFSSL_LOCAL int wc_GetKeyOID(byte* key, word32 keySz, const byte** curveOID, 00840 word32* oidSz, int* algoID, void* heap); 00841 00842 typedef struct tm wolfssl_tm; 00843 #if defined(OPENSSL_ALL) || defined(WOLFSSL_MYSQL_COMPATIBLE) || defined(OPENSSL_EXTRA) || \ 00844 defined(WOLFSSL_NGINX) || defined(WOLFSSL_HAPROXY) 00845 WOLFSSL_LOCAL int GetTimeString(byte* date, int format, char* buf, int len); 00846 #endif 00847 WOLFSSL_LOCAL int ExtractDate(const unsigned char* date, unsigned char format, 00848 wolfssl_tm* certTime, int* idx); 00849 WOLFSSL_LOCAL int ValidateDate(const byte* date, byte format, int dateType); 00850 00851 /* ASN.1 helper functions */ 00852 #ifdef WOLFSSL_CERT_GEN 00853 WOLFSSL_ASN_API int SetName(byte* output, word32 outputSz, CertName* name); 00854 #endif 00855 WOLFSSL_LOCAL int GetShortInt(const byte* input, word32* inOutIdx, int* number, 00856 word32 maxIdx); 00857 WOLFSSL_LOCAL char* GetSigName(int oid); 00858 WOLFSSL_LOCAL int GetLength(const byte* input, word32* inOutIdx, int* len, 00859 word32 maxIdx); 00860 WOLFSSL_LOCAL int GetSequence(const byte* input, word32* inOutIdx, int* len, 00861 word32 maxIdx); 00862 WOLFSSL_LOCAL int GetSet(const byte* input, word32* inOutIdx, int* len, 00863 word32 maxIdx); 00864 WOLFSSL_LOCAL int GetMyVersion(const byte* input, word32* inOutIdx, 00865 int* version, word32 maxIdx); 00866 WOLFSSL_LOCAL int GetInt(mp_int* mpi, const byte* input, word32* inOutIdx, 00867 word32 maxIdx); 00868 #ifdef HAVE_OID_ENCODING 00869 WOLFSSL_LOCAL int EncodeObjectId(const word16* in, word32 inSz, 00870 byte* out, word32* outSz); 00871 #endif 00872 #ifdef HAVE_OID_DECODING 00873 WOLFSSL_LOCAL int DecodeObjectId(const byte* in, word32 inSz, 00874 word16* out, word32* outSz); 00875 #endif 00876 WOLFSSL_LOCAL int GetObjectId(const byte* input, word32* inOutIdx, word32* oid, 00877 word32 oidType, word32 maxIdx); 00878 WOLFSSL_LOCAL int GetAlgoId(const byte* input, word32* inOutIdx, word32* oid, 00879 word32 oidType, word32 maxIdx); 00880 WOLFSSL_LOCAL word32 SetLength(word32 length, byte* output); 00881 WOLFSSL_LOCAL word32 SetSequence(word32 len, byte* output); 00882 WOLFSSL_LOCAL word32 SetOctetString(word32 len, byte* output); 00883 WOLFSSL_LOCAL word32 SetImplicit(byte tag,byte number,word32 len,byte* output); 00884 WOLFSSL_LOCAL word32 SetExplicit(byte number, word32 len, byte* output); 00885 WOLFSSL_LOCAL word32 SetSet(word32 len, byte* output); 00886 WOLFSSL_LOCAL word32 SetAlgoID(int algoOID,byte* output,int type,int curveSz); 00887 WOLFSSL_LOCAL int SetMyVersion(word32 version, byte* output, int header); 00888 WOLFSSL_LOCAL int SetSerialNumber(const byte* sn, word32 snSz, byte* output, 00889 int maxSnSz); 00890 WOLFSSL_LOCAL int GetSerialNumber(const byte* input, word32* inOutIdx, 00891 byte* serial, int* serialSz, word32 maxIdx); 00892 WOLFSSL_LOCAL int GetNameHash(const byte* source, word32* idx, byte* hash, 00893 int maxIdx); 00894 WOLFSSL_LOCAL int wc_CheckPrivateKey(byte* key, word32 keySz, DecodedCert* der); 00895 WOLFSSL_LOCAL int RsaPublicKeyDerSize(RsaKey* key, int with_header); 00896 00897 #ifdef HAVE_ECC 00898 /* ASN sig helpers */ 00899 WOLFSSL_LOCAL int StoreECC_DSA_Sig(byte* out, word32* outLen, mp_int* r, 00900 mp_int* s); 00901 WOLFSSL_LOCAL int DecodeECC_DSA_Sig(const byte* sig, word32 sigLen, 00902 mp_int* r, mp_int* s); 00903 #endif 00904 00905 WOLFSSL_LOCAL void InitSignatureCtx(SignatureCtx* sigCtx, void* heap, int devId); 00906 WOLFSSL_LOCAL void FreeSignatureCtx(SignatureCtx* sigCtx); 00907 00908 #ifndef NO_CERTS 00909 00910 WOLFSSL_LOCAL int PemToDer(const unsigned char* buff, long sz, int type, 00911 DerBuffer** pDer, void* heap, EncryptedInfo* info, 00912 int* eccKey); 00913 WOLFSSL_LOCAL int AllocDer(DerBuffer** der, word32 length, int type, void* heap); 00914 WOLFSSL_LOCAL void FreeDer(DerBuffer** der); 00915 00916 #endif /* !NO_CERTS */ 00917 00918 #ifdef WOLFSSL_CERT_GEN 00919 00920 enum cert_enums { 00921 NAME_ENTRIES = 8, 00922 JOINT_LEN = 2, 00923 EMAIL_JOINT_LEN = 9, 00924 PILOT_JOINT_LEN = 10, 00925 RSA_KEY = 10, 00926 NTRU_KEY = 11, 00927 ECC_KEY = 12, 00928 ED25519_KEY = 13 00929 }; 00930 00931 #endif /* WOLFSSL_CERT_GEN */ 00932 00933 00934 00935 /* for pointer use */ 00936 typedef struct CertStatus CertStatus; 00937 00938 #ifdef HAVE_OCSP 00939 00940 enum Ocsp_Response_Status { 00941 OCSP_SUCCESSFUL = 0, /* Response has valid confirmations */ 00942 OCSP_MALFORMED_REQUEST = 1, /* Illegal confirmation request */ 00943 OCSP_INTERNAL_ERROR = 2, /* Internal error in issuer */ 00944 OCSP_TRY_LATER = 3, /* Try again later */ 00945 OCSP_SIG_REQUIRED = 5, /* Must sign the request (4 is skipped) */ 00946 OCSP_UNAUTHROIZED = 6 /* Request unauthorized */ 00947 }; 00948 00949 00950 enum Ocsp_Cert_Status { 00951 CERT_GOOD = 0, 00952 CERT_REVOKED = 1, 00953 CERT_UNKNOWN = 2 00954 }; 00955 00956 00957 enum Ocsp_Sums { 00958 OCSP_BASIC_OID = 117, 00959 OCSP_NONCE_OID = 118 00960 }; 00961 00962 #ifdef OPENSSL_EXTRA 00963 enum Ocsp_Verify_Error { 00964 OCSP_VERIFY_ERROR_NONE = 0, 00965 OCSP_BAD_ISSUER = 1 00966 }; 00967 #endif 00968 00969 00970 typedef struct OcspRequest OcspRequest; 00971 typedef struct OcspResponse OcspResponse; 00972 00973 00974 struct CertStatus { 00975 CertStatus* next; 00976 00977 byte serial[EXTERNAL_SERIAL_SIZE]; 00978 int serialSz; 00979 00980 int status; 00981 00982 byte thisDate[MAX_DATE_SIZE]; 00983 byte nextDate[MAX_DATE_SIZE]; 00984 byte thisDateFormat; 00985 byte nextDateFormat; 00986 #if defined(OPENSSL_ALL) || defined(WOLFSSL_NGINX) || defined(WOLFSSL_HAPROXY) 00987 byte* thisDateAsn; 00988 byte* nextDateAsn; 00989 #endif 00990 00991 byte* rawOcspResponse; 00992 word32 rawOcspResponseSz; 00993 }; 00994 00995 00996 struct OcspResponse { 00997 int responseStatus; /* return code from Responder */ 00998 00999 byte* response; /* Pointer to beginning of OCSP Response */ 01000 word32 responseSz; /* length of the OCSP Response */ 01001 01002 byte producedDate[MAX_DATE_SIZE]; 01003 /* Date at which this response was signed */ 01004 byte producedDateFormat; /* format of the producedDate */ 01005 byte* issuerHash; 01006 byte* issuerKeyHash; 01007 01008 byte* cert; 01009 word32 certSz; 01010 01011 byte* sig; /* Pointer to sig in source */ 01012 word32 sigSz; /* Length in octets for the sig */ 01013 word32 sigOID; /* OID for hash used for sig */ 01014 01015 CertStatus* status; /* certificate status to fill out */ 01016 01017 byte* nonce; /* pointer to nonce inside ASN.1 response */ 01018 int nonceSz; /* length of the nonce string */ 01019 01020 byte* source; /* pointer to source buffer, not owned */ 01021 word32 maxIdx; /* max offset based on init size */ 01022 01023 #ifdef OPENSSL_EXTRA 01024 int verifyError; 01025 #endif 01026 }; 01027 01028 01029 struct OcspRequest { 01030 byte issuerHash[KEYID_SIZE]; 01031 byte issuerKeyHash[KEYID_SIZE]; 01032 byte* serial; /* copy of the serial number in source cert */ 01033 int serialSz; 01034 byte* url; /* copy of the extAuthInfo in source cert */ 01035 int urlSz; 01036 01037 byte nonce[MAX_OCSP_NONCE_SZ]; 01038 int nonceSz; 01039 void* heap; 01040 void* ssl; 01041 }; 01042 01043 01044 WOLFSSL_LOCAL void InitOcspResponse(OcspResponse*, CertStatus*, byte*, word32); 01045 WOLFSSL_LOCAL int OcspResponseDecode(OcspResponse*, void*, void* heap, int); 01046 01047 WOLFSSL_LOCAL int InitOcspRequest(OcspRequest*, DecodedCert*, byte, void*); 01048 WOLFSSL_LOCAL void FreeOcspRequest(OcspRequest*); 01049 WOLFSSL_LOCAL int EncodeOcspRequest(OcspRequest*, byte*, word32); 01050 WOLFSSL_LOCAL word32 EncodeOcspRequestExtensions(OcspRequest*, byte*, word32); 01051 01052 01053 WOLFSSL_LOCAL int CompareOcspReqResp(OcspRequest*, OcspResponse*); 01054 01055 01056 #endif /* HAVE_OCSP */ 01057 01058 01059 /* for pointer use */ 01060 typedef struct RevokedCert RevokedCert; 01061 01062 #ifdef HAVE_CRL 01063 01064 struct RevokedCert { 01065 byte serialNumber[EXTERNAL_SERIAL_SIZE]; 01066 int serialSz; 01067 RevokedCert* next; 01068 }; 01069 01070 typedef struct DecodedCRL DecodedCRL; 01071 01072 struct DecodedCRL { 01073 word32 certBegin; /* offset to start of cert */ 01074 word32 sigIndex; /* offset to start of signature */ 01075 word32 sigLength; /* length of signature */ 01076 word32 signatureOID; /* sum of algorithm object id */ 01077 byte* signature; /* pointer into raw source, not owned */ 01078 byte issuerHash[SIGNER_DIGEST_SIZE]; /* issuer hash */ 01079 byte crlHash[SIGNER_DIGEST_SIZE]; /* raw crl data hash */ 01080 byte lastDate[MAX_DATE_SIZE]; /* last date updated */ 01081 byte nextDate[MAX_DATE_SIZE]; /* next update date */ 01082 byte lastDateFormat; /* format of last date */ 01083 byte nextDateFormat; /* format of next date */ 01084 RevokedCert* certs; /* revoked cert list */ 01085 int totalCerts; /* number on list */ 01086 void* heap; 01087 }; 01088 01089 WOLFSSL_LOCAL void InitDecodedCRL(DecodedCRL*, void* heap); 01090 WOLFSSL_LOCAL int VerifyCRL_Signature(SignatureCtx* sigCtx, 01091 const byte* toBeSigned, word32 tbsSz, 01092 const byte* signature, word32 sigSz, 01093 word32 signatureOID, Signer *ca, 01094 void* heap); 01095 WOLFSSL_LOCAL int ParseCRL(DecodedCRL*, const byte* buff, word32 sz, void* cm); 01096 WOLFSSL_LOCAL void FreeDecodedCRL(DecodedCRL*); 01097 01098 01099 #endif /* HAVE_CRL */ 01100 01101 01102 #ifdef __cplusplus 01103 } /* extern "C" */ 01104 #endif 01105 01106 #endif /* !NO_ASN */ 01107 01108 01109 #if !defined(NO_ASN) || !defined(NO_PWDBASED) 01110 01111 #ifndef MAX_KEY_SIZE 01112 #define MAX_KEY_SIZE 64 /* MAX PKCS Key length */ 01113 #endif 01114 #ifndef MAX_UNICODE_SZ 01115 #define MAX_UNICODE_SZ 256 01116 #endif 01117 01118 enum PBESTypes { 01119 PBE_MD5_DES = 0, 01120 PBE_SHA1_RC4_128 = 1, 01121 PBE_SHA1_DES = 2, 01122 PBE_SHA1_DES3 = 3, 01123 PBE_AES256_CBC = 4, 01124 01125 PBE_SHA1_RC4_128_SUM = 657, 01126 PBE_SHA1_DES3_SUM = 659, 01127 PBES2 = 13 /* algo ID */ 01128 }; 01129 01130 enum PKCSTypes { 01131 PKCS5v2 = 6, /* PKCS #5 v2.0 */ 01132 PKCS12v1 = 12, /* PKCS #12 */ 01133 PKCS5 = 5, /* PKCS oid tag */ 01134 PKCS8v0 = 0, /* default PKCS#8 version */ 01135 }; 01136 01137 #endif /* !NO_ASN || !NO_PWDBASED */ 01138 01139 #endif /* WOLF_CRYPT_ASN_H */ 01140
Generated on Tue Jul 12 2022 16:58:05 by
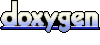