wolfSSL SSL/TLS library, support up to TLS1.3
Embed:
(wiki syntax)
Show/hide line numbers
rsa.h
00001 /* rsa.h 00002 * 00003 * Copyright (C) 2006-2017 wolfSSL Inc. 00004 * 00005 * This file is part of wolfSSL. 00006 * 00007 * wolfSSL is free software; you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation; either version 2 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * wolfSSL is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with this program; if not, write to the Free Software 00019 * Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1335, USA 00020 */ 00021 00022 /* rsa.h for openSSL */ 00023 00024 00025 #ifndef WOLFSSL_RSA_H_ 00026 #define WOLFSSL_RSA_H_ 00027 00028 #include <wolfssl/openssl/bn.h> 00029 00030 00031 #ifdef __cplusplus 00032 extern "C" { 00033 #endif 00034 00035 /* Padding types */ 00036 #define RSA_PKCS1_PADDING 0 00037 #define RSA_PKCS1_OAEP_PADDING 1 00038 00039 #ifndef WOLFSSL_RSA_TYPE_DEFINED /* guard on redeclaration */ 00040 typedef struct WOLFSSL_RSA WOLFSSL_RSA; 00041 #define WOLFSSL_RSA_TYPE_DEFINED 00042 #endif 00043 00044 typedef WOLFSSL_RSA RSA; 00045 00046 struct WOLFSSL_RSA { 00047 #ifdef WC_RSA_BLINDING 00048 WC_RNG* rng; /* for PrivateDecrypt blinding */ 00049 #endif 00050 WOLFSSL_BIGNUM* n; 00051 WOLFSSL_BIGNUM* e; 00052 WOLFSSL_BIGNUM* d; 00053 WOLFSSL_BIGNUM* p; 00054 WOLFSSL_BIGNUM* q; 00055 WOLFSSL_BIGNUM* dmp1; /* dP */ 00056 WOLFSSL_BIGNUM* dmq1; /* dQ */ 00057 WOLFSSL_BIGNUM* iqmp; /* u */ 00058 void* heap; 00059 void* internal; /* our RSA */ 00060 char inSet; /* internal set from external ? */ 00061 char exSet; /* external set from internal ? */ 00062 char ownRng; /* flag for if the rng should be free'd */ 00063 }; 00064 00065 00066 WOLFSSL_API WOLFSSL_RSA* wolfSSL_RSA_new(void); 00067 WOLFSSL_API void wolfSSL_RSA_free(WOLFSSL_RSA*); 00068 00069 WOLFSSL_API int wolfSSL_RSA_generate_key_ex(WOLFSSL_RSA*, int bits, WOLFSSL_BIGNUM*, 00070 void* cb); 00071 00072 WOLFSSL_API int wolfSSL_RSA_blinding_on(WOLFSSL_RSA*, WOLFSSL_BN_CTX*); 00073 WOLFSSL_API int wolfSSL_RSA_public_encrypt(int len, const unsigned char* fr, 00074 unsigned char* to, WOLFSSL_RSA*, int padding); 00075 WOLFSSL_API int wolfSSL_RSA_private_decrypt(int len, const unsigned char* fr, 00076 unsigned char* to, WOLFSSL_RSA*, int padding); 00077 WOLFSSL_API int wolfSSL_RSA_private_encrypt(int len, unsigned char* in, 00078 unsigned char* out, WOLFSSL_RSA* rsa, int padding); 00079 00080 WOLFSSL_API int wolfSSL_RSA_size(const WOLFSSL_RSA*); 00081 WOLFSSL_API int wolfSSL_RSA_sign(int type, const unsigned char* m, 00082 unsigned int mLen, unsigned char* sigRet, 00083 unsigned int* sigLen, WOLFSSL_RSA*); 00084 WOLFSSL_API int wolfSSL_RSA_sign_ex(int type, const unsigned char* m, 00085 unsigned int mLen, unsigned char* sigRet, 00086 unsigned int* sigLen, WOLFSSL_RSA*, int); 00087 WOLFSSL_API int wolfSSL_RSA_verify(int type, const unsigned char* m, 00088 unsigned int mLen, const unsigned char* sig, 00089 unsigned int sigLen, WOLFSSL_RSA*); 00090 WOLFSSL_API int wolfSSL_RSA_public_decrypt(int flen, const unsigned char* from, 00091 unsigned char* to, WOLFSSL_RSA*, int padding); 00092 WOLFSSL_API int wolfSSL_RSA_GenAdd(WOLFSSL_RSA*); 00093 WOLFSSL_API int wolfSSL_RSA_LoadDer(WOLFSSL_RSA*, const unsigned char*, int sz); 00094 WOLFSSL_API int wolfSSL_RSA_LoadDer_ex(WOLFSSL_RSA*, const unsigned char*, int sz, int opt); 00095 00096 #define WOLFSSL_RSA_LOAD_PRIVATE 1 00097 #define WOLFSSL_RSA_LOAD_PUBLIC 2 00098 #define WOLFSSL_RSA_F4 0x10001L 00099 00100 #define RSA_new wolfSSL_RSA_new 00101 #define RSA_free wolfSSL_RSA_free 00102 00103 #define RSA_generate_key_ex wolfSSL_RSA_generate_key_ex 00104 00105 #define RSA_blinding_on wolfSSL_RSA_blinding_on 00106 #define RSA_public_encrypt wolfSSL_RSA_public_encrypt 00107 #define RSA_private_decrypt wolfSSL_RSA_private_decrypt 00108 #define RSA_private_encrypt wolfSSL_RSA_private_encrypt 00109 00110 #define RSA_size wolfSSL_RSA_size 00111 #define RSA_sign wolfSSL_RSA_sign 00112 #define RSA_verify wolfSSL_RSA_verify 00113 #define RSA_public_decrypt wolfSSL_RSA_public_decrypt 00114 00115 #define RSA_F4 WOLFSSL_RSA_F4 00116 00117 #ifdef __cplusplus 00118 } /* extern "C" */ 00119 #endif 00120 00121 #endif /* header */ 00122
Generated on Wed Jul 13 2022 01:38:42 by
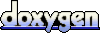