wolfSSL SSL/TLS library, support up to TLS1.3
Embed:
(wiki syntax)
Show/hide line numbers
pem.h
Go to the documentation of this file.
00001 /* pem.h 00002 * 00003 * Copyright (C) 2006-2017 wolfSSL Inc. 00004 * 00005 * This file is part of wolfSSL. 00006 * 00007 * wolfSSL is free software; you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation; either version 2 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * wolfSSL is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with this program; if not, write to the Free Software 00019 * Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1335, USA 00020 */ 00021 00022 /* pem.h for openssl */ 00023 00024 /*! 00025 \file wolfssl/openssl/pem.h 00026 */ 00027 00028 00029 #ifndef WOLFSSL_PEM_H_ 00030 #define WOLFSSL_PEM_H_ 00031 00032 #include <wolfssl/openssl/evp.h> 00033 #include <wolfssl/openssl/bio.h> 00034 #include <wolfssl/openssl/rsa.h> 00035 #include <wolfssl/openssl/dsa.h> 00036 00037 #ifdef __cplusplus 00038 extern "C" { 00039 #endif 00040 00041 /* RSA */ 00042 WOLFSSL_API 00043 int wolfSSL_PEM_write_bio_RSAPrivateKey(WOLFSSL_BIO* bio, WOLFSSL_RSA* rsa, 00044 const EVP_CIPHER* cipher, 00045 unsigned char* passwd, int len, 00046 pem_password_cb* cb, void* arg); 00047 WOLFSSL_API 00048 WOLFSSL_RSA* wolfSSL_PEM_read_bio_RSAPrivateKey(WOLFSSL_BIO* bio, 00049 WOLFSSL_RSA**, 00050 pem_password_cb* cb, 00051 void* arg); 00052 WOLFSSL_API 00053 int wolfSSL_PEM_write_mem_RSAPrivateKey(RSA* rsa, const EVP_CIPHER* cipher, 00054 unsigned char* passwd, int len, 00055 unsigned char **pem, int *plen); 00056 #if !defined(NO_FILESYSTEM) 00057 WOLFSSL_API 00058 int wolfSSL_PEM_write_RSAPrivateKey(FILE *fp, WOLFSSL_RSA *rsa, 00059 const EVP_CIPHER *enc, 00060 unsigned char *kstr, int klen, 00061 pem_password_cb *cb, void *u); 00062 WOLFSSL_API 00063 WOLFSSL_RSA *wolfSSL_PEM_read_RSAPublicKey(FILE *fp, WOLFSSL_RSA **x, 00064 pem_password_cb *cb, void *u); 00065 WOLFSSL_API 00066 int wolfSSL_PEM_write_RSAPublicKey(FILE *fp, WOLFSSL_RSA *x); 00067 00068 WOLFSSL_API 00069 int wolfSSL_PEM_write_RSA_PUBKEY(FILE *fp, WOLFSSL_RSA *x); 00070 #endif /* NO_FILESYSTEM */ 00071 00072 /* DSA */ 00073 WOLFSSL_API 00074 int wolfSSL_PEM_write_bio_DSAPrivateKey(WOLFSSL_BIO* bio, 00075 WOLFSSL_DSA* dsa, 00076 const EVP_CIPHER* cipher, 00077 unsigned char* passwd, int len, 00078 pem_password_cb* cb, void* arg); 00079 WOLFSSL_API 00080 int wolfSSL_PEM_write_mem_DSAPrivateKey(WOLFSSL_DSA* dsa, 00081 const EVP_CIPHER* cipher, 00082 unsigned char* passwd, int len, 00083 unsigned char **pem, int *plen); 00084 #if !defined(NO_FILESYSTEM) 00085 WOLFSSL_API 00086 int wolfSSL_PEM_write_DSAPrivateKey(FILE *fp, WOLFSSL_DSA *dsa, 00087 const EVP_CIPHER *enc, 00088 unsigned char *kstr, int klen, 00089 pem_password_cb *cb, void *u); 00090 WOLFSSL_API 00091 int wolfSSL_PEM_write_DSA_PUBKEY(FILE *fp, WOLFSSL_DSA *x); 00092 #endif /* NO_FILESYSTEM */ 00093 00094 /* ECC */ 00095 WOLFSSL_API 00096 int wolfSSL_PEM_write_bio_ECPrivateKey(WOLFSSL_BIO* bio, WOLFSSL_EC_KEY* ec, 00097 const EVP_CIPHER* cipher, 00098 unsigned char* passwd, int len, 00099 pem_password_cb* cb, void* arg); 00100 WOLFSSL_API 00101 int wolfSSL_PEM_write_mem_ECPrivateKey(WOLFSSL_EC_KEY* key, 00102 const EVP_CIPHER* cipher, 00103 unsigned char* passwd, int len, 00104 unsigned char **pem, int *plen); 00105 #if !defined(NO_FILESYSTEM) 00106 WOLFSSL_API 00107 int wolfSSL_PEM_write_ECPrivateKey(FILE *fp, WOLFSSL_EC_KEY *key, 00108 const EVP_CIPHER *enc, 00109 unsigned char *kstr, int klen, 00110 pem_password_cb *cb, void *u); 00111 WOLFSSL_API 00112 int wolfSSL_PEM_write_EC_PUBKEY(FILE *fp, WOLFSSL_EC_KEY *key); 00113 #endif /* NO_FILESYSTEM */ 00114 00115 /* EVP_KEY */ 00116 WOLFSSL_API 00117 WOLFSSL_EVP_PKEY* wolfSSL_PEM_read_bio_PrivateKey(WOLFSSL_BIO* bio, 00118 WOLFSSL_EVP_PKEY**, 00119 pem_password_cb* cb, 00120 void* arg); 00121 WOLFSSL_API 00122 int wolfSSL_PEM_write_bio_PrivateKey(WOLFSSL_BIO* bio, WOLFSSL_EVP_PKEY* key, 00123 const WOLFSSL_EVP_CIPHER* cipher, 00124 unsigned char* passwd, int len, 00125 pem_password_cb* cb, void* arg); 00126 00127 #if !defined(NO_FILESYSTEM) 00128 WOLFSSL_API 00129 WOLFSSL_EVP_PKEY *wolfSSL_PEM_read_PUBKEY(FILE *fp, EVP_PKEY **x, 00130 pem_password_cb *cb, void *u); 00131 WOLFSSL_API 00132 WOLFSSL_X509 *wolfSSL_PEM_read_X509(FILE *fp, WOLFSSL_X509 **x, 00133 pem_password_cb *cb, void *u); 00134 WOLFSSL_API 00135 WOLFSSL_EVP_PKEY *wolfSSL_PEM_read_PrivateKey(FILE *fp, WOLFSSL_EVP_PKEY **x, 00136 pem_password_cb *cb, void *u); 00137 #endif /* NO_FILESYSTEM */ 00138 00139 #define PEM_read_X509 wolfSSL_PEM_read_X509 00140 #define PEM_read_PrivateKey wolfSSL_PEM_read_PrivateKey 00141 #define PEM_write_bio_PrivateKey wolfSSL_PEM_write_bio_PrivateKey 00142 /* RSA */ 00143 #define PEM_write_bio_RSAPrivateKey wolfSSL_PEM_write_bio_RSAPrivateKey 00144 #define PEM_read_bio_RSAPrivateKey wolfSSL_PEM_read_bio_RSAPrivateKey 00145 #define PEM_write_RSAPrivateKey wolfSSL_PEM_write_RSAPrivateKey 00146 #define PEM_write_RSA_PUBKEY wolfSSL_PEM_write_RSA_PUBKEY 00147 #define PEM_write_RSAPublicKey wolfSSL_PEM_write_RSAPublicKey 00148 #define PEM_read_RSAPublicKey wolfSSL_PEM_read_RSAPublicKey 00149 /* DSA */ 00150 #define PEM_write_bio_DSAPrivateKey wolfSSL_PEM_write_bio_DSAPrivateKey 00151 #define PEM_write_DSAPrivateKey wolfSSL_PEM_write_DSAPrivateKey 00152 #define PEM_write_DSA_PUBKEY wolfSSL_PEM_write_DSA_PUBKEY 00153 /* ECC */ 00154 #define PEM_write_bio_ECPrivateKey wolfSSL_PEM_write_bio_ECPrivateKey 00155 #define PEM_write_EC_PUBKEY wolfSSL_PEM_write_EC_PUBKEY 00156 #define PEM_write_ECPrivateKey wolfSSL_PEM_write_ECPrivateKey 00157 /* EVP_KEY */ 00158 #define PEM_read_bio_PrivateKey wolfSSL_PEM_read_bio_PrivateKey 00159 #define PEM_read_PUBKEY wolfSSL_PEM_read_PUBKEY 00160 00161 #ifdef __cplusplus 00162 } /* extern "C" */ 00163 #endif 00164 00165 #endif /* WOLFSSL_PEM_H_ */ 00166 00167
Generated on Wed Jul 13 2022 01:38:42 by
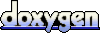