mDNS
Embed:
(wiki syntax)
Show/hide line numbers
dns-sd.h
00001 /* 00002 Edited by JB RYU, KOR - 2015 00003 00004 DNS-headers and values. 00005 00006 Actually, Defines for DNS-type-values are not necessary. 00007 LWIP NETIF has defines for DNS formats already. 00008 */ 00009 00010 #ifndef DNS_SD_H_ 00011 #define DNS_SD_H_ 00012 00013 #include <cstdint> 00014 00015 #ifdef __cplusplus 00016 extern "C" { 00017 #endif 00018 00019 #define UPPERBYTE(word)\ 00020 (unsigned char)(word >> 8) 00021 #define LOWERBYTE(word)\ 00022 (unsigned char)(word) 00023 00024 /** \internal The DNS message header. */ 00025 #define DNS_FLAG1_RESPONSE 0x80 00026 #define DNS_FLAG1_OPCODE_STATUS 0x10 00027 #define DNS_FLAG1_OPCODE_INVERSE 0x08 00028 #define DNS_FLAG1_OPCODE_STANDARD 0x00 00029 #define DNS_FLAG1_AUTHORATIVE 0x04 00030 #define DNS_FLAG1_TRUNC 0x02 00031 #define DNS_FLAG1_RD 0x01 00032 #define DNS_FLAG2_RA 0x80 00033 #define DNS_FLAG2_ERR_MASK 0x0f 00034 #define DNS_FLAG2_ERR_NONE 0x00 00035 #define DNS_FLAG2_ERR_NAME 0x03 00036 00037 /** \internal The DNS question message structure. */ 00038 struct dns_question 00039 { 00040 uint16_t type; 00041 uint16_t obj; 00042 }; 00043 00044 struct dns_hdr 00045 { 00046 uint16_t id; 00047 uint8_t flags1, flags2; 00048 uint16_t numquestions; 00049 uint16_t numanswers; 00050 uint16_t numauthrr; 00051 uint16_t numextrarr; 00052 }; 00053 00054 /** \internal The DNS answer message structure. */ 00055 struct dns_answer 00056 { 00057 /* DNS answer record starts with either a domain name or a pointer 00058 * to a name already present somewhere in the packet. */ 00059 uint16_t type; 00060 uint16_t obj; 00061 uint16_t ttl[2]; 00062 uint16_t len; 00063 #if NET_IPV6 00064 uint8_t ipaddr[16]; 00065 #else 00066 uint8_t ipaddr[4]; 00067 #endif 00068 }; 00069 00070 #define DNS_TYPE_A 1 00071 #define DNS_TYPE_CNAME 5 00072 #define DNS_TYPE_PTR 12 00073 #define DNS_TYPE_MX 15 00074 #define DNS_TYPE_TXT 16 00075 #define DNS_TYPE_AAAA 28 00076 #define DNS_TYPE_SRV 33 00077 #define DNS_TYPE_ANY 255 00078 #define DNS_TYPE_NSEC 47 00079 00080 #if NET_IPV6 00081 #define NATIVE_DNS_TYPE DNS_TYPE_AAAA /* IPv6 */ 00082 #else 00083 #define NATIVE_DNS_TYPE DNS_TYPE_A /* IPv4 */ 00084 #endif 00085 00086 #define DNS_CLASS_IN 0x0001 00087 #define DNS_CASH_FLUSH 0x8000 00088 #define DNS_CLASS_ANY 0z00ff 00089 00090 /* all entities include its length */ 00091 #define MAX_DOMAIN_LEN 64 00092 #define MAX_INSTANCE_NAME_LEN 64 00093 #define MAX_SUBTYPE_NAME_LEN 69 00094 #define MAX_SERVICE_NAME_LEN 22 00095 #define MAX_SERVICE_DOMAIN_LEN 64 00096 #define MAX_PARENT_DOMAIN_LEN 100 //.local so... 00097 #define MDNS_P_DOMAIN_LEN 5 00098 00099 #define PTR_QUERY_NAME_LEN 18 00100 #pragma pack (push, 1) 00101 typedef struct 00102 { 00103 /* DNS answer record starts with either a domain name or a pointer 00104 * to a name already present somewhere in the packet. */ 00105 uint16_t type; 00106 uint16_t obj; 00107 uint16_t ttl[2]; 00108 uint16_t len; 00109 char name[MAX_INSTANCE_NAME_LEN 00110 + MAX_SERVICE_NAME_LEN + MDNS_P_DOMAIN_LEN]; 00111 }PTR_ANS; 00112 00113 typedef struct 00114 { 00115 uint16_t type; 00116 uint16_t obj; 00117 uint16_t ttl[2]; 00118 uint16_t len; 00119 uint16_t pri; 00120 uint16_t wei; 00121 uint16_t port; 00122 char name[MAX_DOMAIN_LEN 00123 + MDNS_P_DOMAIN_LEN]; 00124 }SRV_ANS; 00125 00126 typedef struct 00127 { 00128 uint16_t type; 00129 uint16_t obj; 00130 uint16_t ttl[2]; 00131 uint16_t len; 00132 char txt[1024]; 00133 }TXT_ANS; 00134 00135 typedef struct 00136 { 00137 uint8_t len_txtvers; 00138 char txtvers[10]; 00139 uint8_t len_dcap; 00140 char dcap[10]; 00141 uint8_t len_path; 00142 char path[10]; 00143 uint8_t len_https; 00144 char https[10]; 00145 uint8_t len_level; 00146 char level[10]; 00147 }__SEP_TXT; 00148 00149 typedef struct 00150 { 00151 uint8_t inst_len; 00152 char instance[63]; //RYU-PC 00153 uint8_t serv_len; 00154 char service[16]; //_http 00155 uint8_t trl_len; 00156 char trl[4]; //_tcp 00157 uint8_t domain_len; 00158 char domain[5]; //local 00159 __SEP_TXT txt; 00160 }__SD_DOMAIN; 00161 #pragma pack (pop) 00162 00163 #define N_CONTIKI_SERVICES 1 00164 typedef struct 00165 { 00166 int numbers; 00167 __SD_DOMAIN elements[N_CONTIKI_SERVICES]; 00168 }MY_SD_DOMAINS; 00169 00170 typedef struct 00171 { 00172 int numbers; 00173 uint8_t reqs[10]; 00174 }QR_MAP; 00175 00176 #ifdef __cplusplus 00177 } 00178 #endif 00179 00180 #endif 00181
Generated on Sat Jul 23 2022 00:40:12 by
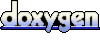