
Demo example on FRDM-64F https://ide.mbed.com/compiler/#nav:/rzr-example-mbed;
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /// SPDX-License-Indentifier: ISC 00002 /// Copyright: 2020+ Philippe Coval <https://purl.org/rzr/> 00003 /// URL: https://os.mbed.com/users/rzrfreefr/code/rzr-example-mbed/ 00004 00005 #include "mbed.h" 00006 00007 #include <EthernetInterface.h> 00008 #include <USBKeyboard.h> 00009 #include <FXOS8700Q.h> 00010 #include "Utils.h" 00011 00012 //#define CONFIG_LOG 1 00013 //#define CONFIG_NET 1 00014 #define CONFIG_USB 1 00015 00016 #if defined(CONFIG_LOG) && CONFIG_LOG 00017 # define logf printf 00018 #else 00019 # define logf if (false) printf 00020 #endif 00021 00022 00023 int main() 00024 { 00025 logf("#{ %s\n", __FILE__); 00026 00027 DigitalOut led(LED1); 00028 led = false; //on 00029 00030 I2C i2c(PTE25, PTE24); 00031 motion_data_units_t acc_data; 00032 motion_data_counts_t acc_raw; 00033 00034 float vorigin[] = {0, 0, 0}; 00035 float vacc[] = {0, 0, 0}; 00036 const int len = 16; 00037 float vhist[len][3]; 00038 00039 float vmean[] = {0, 0, 0}; 00040 float vmin[] = {0, 0, 0}; 00041 float vmax[] = {0, 0, 0}; 00042 00043 // cis: 10 < 12 < 13Log < 15 < 20N < 25< 35N < 40 < ~50 < 60 < !75 00044 // pin: 12? < 50 00045 float threshold = 1./25; 00046 float vthreshold[] = {threshold, threshold, threshold}; 00047 const float delay = 1000.f / 25 / len; 00048 00049 #if 0 00050 int keymap[3][2] = { 00051 { RIGHT_ARROW, LEFT_ARROW }, 00052 { DOWN_ARROW, UP_ARROW }, 00053 { KEY_PAGE_DOWN, KEY_PAGE_UP } 00054 }; 00055 #elif 1 00056 int keymap[3][2] = { 00057 { KEY_RCTRL, KEY_CTRL }, 00058 { DOWN_ARROW, UP_ARROW }, 00059 { KEY_PAGE_DOWN, KEY_PAGE_UP } 00060 }; 00061 #elif 0 // https://github.com/ARMmbed/mbed-os/blob/c6094f7b36dc0e90a6a7271870333fbba475286c/drivers/usb/source/USBKeyboard.cpp 00062 int keymap[3][2] = { 00063 { 0x4f, 0x50 }, 00064 { 0x51, 0x52 }, 00065 { 0x4b, 0x4e } 00066 }; 00067 #endif 00068 00069 00070 FXOS8700QAccelerometer acc(i2c, FXOS8700CQ_SLAVE_ADDR1); // Proper Ports and I2C Address for K64F Freedom board 00071 #if defined(CONFIG_USB) && CONFIG_USB 00072 logf("# usb:\n"); 00073 USBKeyboard usb; ///< https://os.mbed.com/docs/mbed-os/v6.2/apis/usbkeyboard.html 00074 #endif 00075 00076 #if defined(CONFIG_NET) && CONFIG_NET 00077 EthernetInterface eth; ///< https://os.mbed.com/docs/mbed-os/v6.2/apis/ethernet.html 00078 eth.set_dhcp(true); 00079 eth.connect(); 00080 logf("\n# MAC address: %s\n", eth.get_mac_address()); 00081 SocketAddress a; 00082 eth.get_ip_address(&a); 00083 char* addr = a.get_ip_address() || "127.0.0.1"; 00084 logf("# IP address: %s\n", addr); 00085 #endif 00086 00087 acc.enable(); 00088 acc.getAxis(acc_data); 00089 vorigin[0] = acc_data.x; 00090 vorigin[1] = acc_data.y; 00091 vorigin[2] = acc_data.z; 00092 00093 logf("\r\n\n# FXOS8700Q: Who Am I= %X\r\n", acc.whoAmI()); 00094 00095 int key = 0; 00096 for(int iter=0; iter<len; ++iter) { 00097 if (false) led = !led; 00098 acc.getAxis(acc_data); 00099 vacc[0] = acc_data.x - vorigin[0]; 00100 vacc[1] = acc_data.y - vorigin[1]; 00101 vacc[2] = acc_data.z - vorigin[2]; 00102 logf("{\"accel\":[%.2f,%.2f,%.2f]}\r\n", vacc[0], vacc[1], vacc[2]); 00103 00104 #if defined(CONFIG_USB) && CONFIG_USB 00105 usb.connect(); 00106 00107 if (key == 0) { 00108 for(int i=2; i>=0; i--) { 00109 if (vacc[i] > vthreshold[i]) { 00110 key = keymap[i][0]; 00111 } else if (vacc[i] < -vthreshold[i]) { 00112 key = keymap[i][1]; 00113 } 00114 } 00115 00116 if (key != 0) { 00117 led = false; //on 00118 logf("# key: %0X\n", key); 00119 #if 0 00120 Thread thread; 00121 thread.start(idle); 00122 #endif 00123 00124 if (!true) { 00125 usb.key_code(key, key); 00126 } else if (false) { 00127 usb.key_code(key); 00128 } else { 00129 Utils::keyboard_key_code(usb, key, key); 00130 } 00131 } 00132 iter=0; 00133 continue; 00134 } 00135 #endif 00136 00137 for (int i=0; i<3; i++) { 00138 vhist[iter][i] = vacc[i]; 00139 } 00140 00141 if (iter==len-1) { 00142 led = false; // heart beat 00143 led = true; 00144 00145 for (int i=0; i<3; i++) { 00146 vmean[i] = 0; 00147 vmin[i] = vmax[i] = vhist[iter][i]; 00148 } 00149 00150 for (iter=0; iter<len; iter++) { 00151 for (int i=0; i<3; i++) { 00152 vmean[i] += vhist[iter][i]; 00153 if (vmin[i] > vhist[iter][i]) { 00154 vmin[i] = vhist[iter][i]; 00155 } 00156 if (vmax[i] < vhist[iter][i]) { 00157 vmax[i] = vhist[iter][i]; 00158 } 00159 } 00160 } 00161 for (int i=0; i<3; i++) { 00162 vmean[i] /= len; 00163 } 00164 00165 bool reset = true; 00166 for (int k=0; k<3; k++) { 00167 if (abs(vmax[k] - vmin[k]) > threshold*.8) { 00168 reset &= false; 00169 } 00170 } 00171 if (reset) { 00172 logf("\r\n# Reset origin\n"); 00173 vorigin[0] = acc_data.x; 00174 vorigin[1] = acc_data.y; 00175 vorigin[2] = acc_data.z; 00176 00177 key = 0; // unbounce 00178 led = true; //off 00179 } 00180 iter=0; 00181 } 00182 ThisThread::sleep_for(delay); 00183 } 00184 logf("#} %s\n", __FILE__); 00185 }
Generated on Tue Aug 2 2022 06:03:56 by
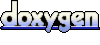