Voltage Monitor Class widh LED Alert
Embed:
(wiki syntax)
Show/hide line numbers
VoltageMonitor.h
00001 /* 00002 * Voltage Monitor Class 00003 * 00004 * 2017.11.26 00005 * 00006 */ 00007 00008 #ifndef _VOLTAGE_MONIOR_H_ 00009 #define _VOLTAGE_MONIOR_H_ 00010 00011 #include "mbed.h" 00012 00013 class VoltageMonitor 00014 { 00015 public: 00016 enum { 00017 VM_NORMAL = 0, 00018 VM_UNDER = -1, 00019 VM_OVER = 1 00020 }; 00021 00022 //--------------------------------------------------------------------- 00023 // パラメータ 00024 // _ain: 電圧監視用ADC 00025 // _vdd: VDDの電圧値(実測値を指定) 00026 // _loThreshold: 電圧低下閾値 00027 // _hiThreshold: 過電圧閾値 00028 // _pLed: 警告用LED (NULL: LEDを使用しない) 00029 VoltageMonitor(AnalogIn* _pAin, float _vdd, float _loThreshold, float _hiThreshold, DigitalOut* _pLed=NULL) : 00030 pAin(_pAin), 00031 vdd(_vdd), 00032 loThreshold(_loThreshold), 00033 hiThreshold(_hiThreshold), 00034 pLed(_pLed), 00035 status(VM_NORMAL) 00036 { 00037 if (pLed != NULL) { 00038 *pLed = 1; 00039 } 00040 }; 00041 00042 ~VoltageMonitor() {}; 00043 00044 //--------------------------------------------------------------------- 00045 // 返り値 00046 // -1: 電圧低下 00047 // 0: 正常 00048 // 1: 過電圧 00049 int check() 00050 { 00051 float vMeas = pAin->read() * vdd; 00052 00053 int st; 00054 if (vMeas < loThreshold) st = VM_UNDER; 00055 else if (vMeas > hiThreshold) st = VM_OVER; 00056 else st = VM_NORMAL; 00057 00058 #if UART_TRACE 00059 printf("VoltageMonitor:\t%.3f\t %d\r\n", vMeas, st); 00060 //printf("%.3f\r\n", vMeas); 00061 #endif 00062 00063 if (st != status) { 00064 status = st; 00065 if (pLed != NULL) { 00066 switch (status) { 00067 case VM_UNDER: 00068 t.attach(this, &VoltageMonitor::blinkLed, 0.5); 00069 break; 00070 case VM_OVER: 00071 t.attach(this, &VoltageMonitor::blinkLed, 0.1); 00072 break; 00073 case VM_NORMAL: 00074 t.detach(); 00075 *pLed = 1; 00076 } 00077 } 00078 } 00079 00080 return status; 00081 }; 00082 00083 private: 00084 AnalogIn* pAin; 00085 float vdd; 00086 float loThreshold; 00087 float hiThreshold; 00088 DigitalOut* pLed; 00089 Ticker t; 00090 int status; 00091 00092 void blinkLed() { 00093 if (pLed != NULL) { 00094 *pLed = !*pLed; 00095 } 00096 }; 00097 }; 00098 00099 #endif //_VOLTAGE_MONIOR_H_
Generated on Sun Jul 17 2022 10:23:40 by
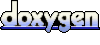