
wheelchair code for driver assitance
Fork of wheelchairalexa by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "wheelchair.h" 00002 00003 AnalogIn x(A0); 00004 AnalogIn y(A1); 00005 00006 DigitalOut on(D12); 00007 DigitalOut off(D11); 00008 DigitalOut up(D2); 00009 DigitalOut down(D3); 00010 00011 bool manual = false; 00012 bool leftBound = false; 00013 bool rightBound = false; 00014 bool straightBound = false; 00015 00016 Serial pc(USBTX, USBRX, 9600); 00017 Timer t; 00018 00019 Wheelchair smart(xDir,yDir, &pc, &t); 00020 00021 00022 int main(void) 00023 { 00024 smart.stop(); 00025 while(1) { 00026 pc.printf("turning on joystick\n"); 00027 manual = true; 00028 t.reset(); 00029 while(manual) { 00030 smart.move(x,y,leftBound,rightBound,straightBound); 00031 if( pc.readable()) { 00032 char d = pc.getc(); 00033 if( d == 'l') { 00034 leftBound = true; 00035 } 00036 00037 if (d == 'e') { 00038 leftBound = false; 00039 } 00040 00041 if( d == 'f') { 00042 straightBound = true; 00043 } 00044 00045 if (d == 'o') { 00046 straightBound = false; 00047 } 00048 00049 if( d == 'r') { 00050 rightBound = true; 00051 } 00052 00053 if( d == 'i') { 00054 rightBound = false; 00055 } 00056 00057 if( d == 'm') { 00058 pc.printf("turning off joystick\n"); 00059 manual = false; 00060 } 00061 } 00062 } 00063 } 00064 00065 wait(process); 00066 } 00067 00068 00069
Generated on Thu Jul 21 2022 11:57:26 by
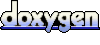