;
Dependents: Audio_Player Wav_player_RPG_menu OMNi-Robot_no_borrar
Wav_Support_File.cpp
00001 /* Wav File Support */ 00002 /* **************** */ 00003 00004 #include "mbed.h" 00005 //#include "misra_types.h" 00006 //#include "Wav_Support_File.h" 00007 00008 #define DEBUG 0 00009 00010 /* Function Prototypes */ 00011 unsigned char wave_file_check(unsigned char *wave_array, FILE *open_file); 00012 00013 00014 /* Global Variables */ 00015 struct wave_header_construction 00016 { char identification_block[5]; 00017 unsigned int length_of_file; 00018 char identifier1[5]; 00019 char identifier2[5]; 00020 unsigned int position_of_data; 00021 unsigned short format_tag; 00022 unsigned short number_of_channels; 00023 unsigned int samples_per_second; 00024 unsigned int bytes_per_second; 00025 unsigned short bytes_per_sample; 00026 unsigned short bits_per_sample; 00027 char marker[5]; 00028 unsigned int number_of_bits_in_sample; 00029 } wave_file_info; 00030 00031 00032 00033 00034 00035 /* Wave File Checker */ 00036 unsigned char wave_file_check(unsigned char *wave_array, FILE *open_file) 00037 { 00038 unsigned char brk_flag = 0u; 00039 unsigned char x = 0u; 00040 unsigned char valid_sample = 0u; 00041 short ch = 0; 00042 00043 if(DEBUG) printf("\n\rPrinting out.WAV file header...\n\r"); 00044 00045 for (x = 0; x < 0x2bu; x++) /* Read the first 0x2b bytes of the file as this is the haeder informtation relating to sample rate etc. */ 00046 { 00047 ch = fgetc(open_file); /* Get the first byte from the file */ 00048 if (ch == EOF) /* Check for eof */ 00049 { 00050 if(DEBUG) printf(" File too short to be a wave file\n\r"); /* If we have less than 0x2b then file is not a wave file. */ 00051 valid_sample = 0u; 00052 brk_flag = 1u; 00053 } 00054 else{ 00055 wave_array[x] = ch; /* Load data in to header file array */ 00056 } 00057 00058 if (brk_flag == 1u) 00059 { 00060 break; 00061 } 00062 } 00063 00064 if (brk_flag == 0u) /* OK we have downloaded the header into an Array. Lets check to see if its a valid file */ 00065 { 00066 if(DEBUG) printf(" Identification block (4 ASCII bytes)= "); /* First 4 bytes are header block */ 00067 for (x = 0u; x < 4; x++) 00068 { 00069 //putc(wave_array[x]); /* and write them on the screen */ 00070 wave_file_info.identification_block[x] = wave_array[x]; 00071 } 00072 wave_file_info.identification_block[4] = 0; /* add the string terminator on the end */ 00073 if(DEBUG) printf(" '%s'\n\r", wave_file_info.identification_block); 00074 00075 00076 if(DEBUG) printf(" Length of file (4 bytes) = "); /* Length of file. */ 00077 for (x = 4u; x < 8u; x++) 00078 { 00079 if(DEBUG) printf("%d ",wave_array[x]); 00080 } 00081 wave_file_info.length_of_file = ( ((unsigned int) wave_array[7] << 24) 00082 + ((unsigned int) wave_array[6] << 16) 00083 + ((unsigned int) wave_array[5] << 8) 00084 + ((unsigned int) wave_array[4]) ); 00085 if(DEBUG) printf(" = %d bytes\n\r", wave_file_info.length_of_file); 00086 00087 00088 if(DEBUG) printf(" Identifier (4 ASCII bytes) = "); /* indentifier string1 */ 00089 for (x = 8u; x < 12u; x++) 00090 { 00091 //putc(wave_array[x]); /* and write them on the screen */ 00092 wave_file_info.identifier1[x-8] = wave_array[x]; 00093 } 00094 wave_file_info.identification_block[4] = 0; /* add the string terminator on the end */ 00095 if(DEBUG) printf(" '%s'\n\r", wave_file_info.identifier1); 00096 00097 00098 if(DEBUG) printf(" Identifier (4 ASCII bytes) = "); /* Identifier String 2 */ 00099 for (x = 12; x < 16u; x++) 00100 { 00101 //putc(wave_array[x]); /* and write them on the screen */ 00102 wave_file_info.identifier2[x-12] = wave_array[x]; 00103 } 00104 wave_file_info.identifier2[4] = 0; /* add the string terminator on the end */ 00105 if(DEBUG) printf(" '%s'\n\r", wave_file_info.identifier2); 00106 00107 00108 if(DEBUG) printf(" Position of data (4 bytes) = "); /* Position of data */ 00109 for (x = 16u; x < 20u; x++) 00110 { 00111 if(DEBUG) printf("%d ",wave_array[x]); 00112 } 00113 wave_file_info.position_of_data = ( ((unsigned int) wave_array[19] << 24) 00114 + ((unsigned int) wave_array[18] << 16) 00115 + ((unsigned int) wave_array[17] << 8) 00116 + ((unsigned int) wave_array[16]) ); 00117 if(DEBUG) printf(" = %d\n\r", wave_file_info.position_of_data); 00118 00119 00120 if(DEBUG) printf(" Format Tag - Always 1 for Wave PCM (2 bytes) = "); /* format tag */ 00121 for (x = 20u; x < 22u; x++) 00122 { 00123 if(DEBUG) printf("%d ",wave_array[x]); 00124 } 00125 wave_file_info.format_tag = ( ((unsigned int) wave_array[21] << 8) 00126 + ((unsigned int) wave_array[20]) ); 00127 if(DEBUG) printf(" = %d\n\r", wave_file_info.format_tag); 00128 00129 00130 if(DEBUG) printf(" Number of channels (2 bytes) = "); /* Number of channels, mono or steroeo */ 00131 for (x = 22u; x < 24u; x++) 00132 { 00133 if(DEBUG) printf("%d ",wave_array[x]); 00134 } 00135 wave_file_info.number_of_channels = ( ((unsigned int) wave_array[23] << 8) 00136 + ((unsigned int) wave_array[22]) ); 00137 if(DEBUG) printf(" = %d channel(s)\n\r", wave_file_info.number_of_channels); 00138 00139 00140 if(DEBUG) printf(" Samples per second (4 bytes) = "); /* Samples per second */ 00141 for (x = 24u; x < 28u; x++) 00142 { 00143 if(DEBUG) printf("%d ",wave_array[x]); 00144 } 00145 wave_file_info.samples_per_second =( ((unsigned int) wave_array[27] << 24) 00146 + ((unsigned int) wave_array[26] << 16) 00147 + ((unsigned int) wave_array[25] << 8) 00148 + ((unsigned int) wave_array[24]) ); 00149 if(DEBUG) printf(" = %d samples per sec\n\r", wave_file_info.samples_per_second); 00150 00151 00152 if(DEBUG) printf(" Bytes Per Second (4 bytes) = "); /* Bytes per second */ 00153 for (x = 28u; x < 32u; x++) 00154 { 00155 if(DEBUG) printf("%d ",wave_array[x]); 00156 } 00157 wave_file_info.bytes_per_second = ( ((unsigned int) wave_array[31] << 24) 00158 + ((unsigned int) wave_array[30] << 16) 00159 + ((unsigned int) wave_array[29] << 8) 00160 + ((unsigned int) wave_array[28]) ); 00161 if(DEBUG) printf(" = %d bytes per sec\n\r", wave_file_info.bytes_per_second); 00162 00163 00164 if(DEBUG) printf(" Bytes Per Sample (2 bytes) = "); /* Bytes per sample */ 00165 for (x = 32u; x < 34u; x++) 00166 { 00167 if(DEBUG) printf("%d ",wave_array[x]); 00168 } 00169 wave_file_info.bytes_per_sample = ( ((unsigned int) wave_array[33] << 8) 00170 + ((unsigned int) wave_array[32]) ); 00171 if(DEBUG) printf(" = %d bytes\n\r", wave_file_info.bytes_per_sample); 00172 00173 00174 if(DEBUG) printf(" Bits Per Sample (2 bytes) = "); /* Bits per sample */ 00175 for (x = 34u; x < 36u; x++) 00176 { 00177 if(DEBUG) printf("%d ",wave_array[x]); 00178 } 00179 wave_file_info.bits_per_sample = ( ((unsigned int) wave_array[35] << 8) 00180 + ((unsigned int) wave_array[34]) ); 00181 if(DEBUG) printf(" = %d bits\n\r", wave_file_info.bits_per_sample); 00182 00183 00184 if(DEBUG) printf(" Marker (4 ASCII bytes) = "); /* Marker */ 00185 for (x = 36u; x < 40u; x++) 00186 { 00187 //putc(wave_array[x]); 00188 wave_file_info.marker[x-36] = wave_array[x]; 00189 } 00190 wave_file_info.marker[4] = 0; 00191 if(DEBUG) printf(" '%s'\n\r", wave_file_info.marker); 00192 00193 00194 if(DEBUG) printf(" The number of bits in the sample (4 bytes) = "); /* Number of bits per sample. */ 00195 for (x = 40u; x < 44u; x++) 00196 { 00197 if(DEBUG) printf("%d ",wave_array[x]); 00198 } 00199 wave_file_info.number_of_bits_in_sample = ( ((unsigned int) wave_array[43] << 24) 00200 + ((unsigned int) wave_array[42] << 16) 00201 + ((unsigned int) wave_array[41] << 8) 00202 + ((unsigned int) wave_array[40]) ); 00203 if(DEBUG) printf(" = %d bits\n\r", wave_file_info.number_of_bits_in_sample); 00204 00205 00206 /* Check for valid Wave file */ 00207 if (((wave_array[0] == 'R') && (wave_array[1] == 'I') && (wave_array[2] == 'F') && (wave_array[3] == 'F')) 00208 && ((wave_array[8] == 'W') && (wave_array[9] == 'A') && (wave_array[0x0a] == 'V') && (wave_array[0x0b] == 'E')) 00209 && ((wave_array[0x0c] == 'f') && (wave_array[0x0d] == 'm') && (wave_array[0x0e] == 't') && (wave_array[0x0f] == ' ')) 00210 && (wave_array[0x14] == 0x01)) valid_sample = 1u; 00211 } 00212 00213 if(DEBUG) printf("Finished examining the .WAV file header\n\r"); 00214 if (valid_sample == 1) 00215 { 00216 if(DEBUG) printf("Sample is a valid .WAV sample file\n\n\r"); 00217 } 00218 else{ 00219 if(DEBUG) printf("Sample is a NOT a valid .WAV sample file\n\n\r"); 00220 } 00221 00222 return valid_sample; 00223 }
Generated on Wed Jul 13 2022 18:13:41 by
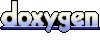