one wire driver
Dependents: 09_PT1000 10_PT1000 11_PT1000
DS2482.h
00001 // \file ds2482x_app.h \brief Dallas DS2482 I2C-to-Dallas1Wire Master Library. 00002 //***************************************************************************** 00003 // Target MCU : Atmel AVR Series 00004 //***************************************************************************** 00005 // 00006 00007 #ifndef DS2482_H 00008 #define DS2482_H 00009 00010 #include "mbed.h" 00011 00012 #define OW_MASTER_START 0 00013 #define OW_MASTER_DEBUG 0 00014 00015 // constants/macros/typdefs 00016 #define OW_I2C_DEVICE 0x30 // < Change this to the address of your 1-Wire master 00017 00018 #define FALSE 0 00019 #define TRUE 1 00020 00021 #define NAK 0 00022 #define ACK 1 00023 00024 #define I2C_READ 1 00025 #define I2C_WRITE 0 00026 00027 #define POLL_LIMIT 100 00028 00029 // Maximale Anzahl der Bausteine am Bus 00030 #define OW_MAX_DEVICES 16 00031 #define OW_SECOND_PORT 8 00032 00033 // Memory definitions 00034 #define OW_SCRATCHPAD_BYTES 9 00035 #define OW_ROM_CODE_BYTES 8 00036 #define OW_CRC_BYTE 8 00037 00038 00039 //----------------------------------------------------------------------------- 00040 00041 // Commands 00042 #define OW_CMD_SEARCH_ROM 0xF0 // Command Search ROM code 00043 #define OW_CMD_READ_ROM 0x33 // Command Read ROM code 00044 #define OW_CMD_MATCH_ROM 0x55 // Command Match ROM code 00045 #define OW_CMD_SKIP_ROM 0xCC // Command Skip ROM code 00046 #define OW_CMD_ALARM_SEARCH 0xEC // Command Alarm Search 00047 00048 #define OW_CMD_CONVERT_T 0x44 // Command Initiate temperature conversion 00049 #define OW_CMD_WRITE_SCRATCHPAD 0x4E // Command Write scratchpad 00050 #define OW_CMD_READ_SCRATCHPAD 0xBE // Command Read scratchpad 00051 #define OW_CMD_COPY_SCRATCHPAD 0x48 // Command Copy scratchpad 00052 #define OW_CMD_RECALL_EE 0xB8 // Command Recall Th and Tl from EEPROM 00053 #define OW_CMD_READ_POWER_SUPPLY 0xB4 // Command Signal power supply mode 00054 00055 // Error codes 00056 #define OW_RESET_ERROR 0x01 00057 #define OW_CRC_ERROR 0x02 00058 00059 // DS2482 command defines 00060 #define DS2482_CMD_DRST 0xF0 //< DS2482 Device Reset 00061 #define DS2482_CMD_WCFG 0xD2 //< DS2482 Write Configuration 00062 #define DS2482_CMD_CHSL 0xC3 //< DS2482 Channel Select 00063 #define DS2482_CMD_SRP 0xE1 //< DS2482 Set Read Pointer 00064 #define DS2482_CMD_1WRS 0xB4 //< DS2482 1-Wire Reset 00065 #define DS2482_CMD_1WWB 0xA5 //< DS2482 1-Wire Write Byte 00066 #define DS2482_CMD_1WRB 0x96 //< DS2482 1-Wire Read Byte 00067 #define DS2482_CMD_1WSB 0x87 //< DS2482 1-Wire Single Bit 00068 #define DS2482_CMD_1WT 0x78 //< DS2482 1-Wire Triplet 00069 00070 // DS2482 status register bit defines 00071 #define DS2482_STATUS_1WB 0x01 //< DS2482 Status 1-Wire Busy 00072 #define DS2482_STATUS_PPD 0x02 //< DS2482 Status Presence Pulse Detect 00073 #define DS2482_STATUS_SD 0x04 //< DS2482 Status Short Detected 00074 #define DS2482_STATUS_LL 0x08 //< DS2482 Status 1-Wire Logic Level 00075 #define DS2482_STATUS_RST 0x10 //< DS2482 Status Device Reset 00076 #define DS2482_STATUS_SBR 0x20 //< DS2482 Status Single Bit Result 00077 #define DS2482_STATUS_TSB 0x40 //< DS2482 Status Triplet Second Bit 00078 #define DS2482_STATUS_DIR 0x80 //< DS2482 Status Branch Direction Taken 00079 00080 // DS2482 configuration register bit defines 00081 #define DS2482_CFG_APU 0x01 //< DS2482 Config Active Pull-Up 00082 #define DS2482_CFG_PPM 0x02 //< DS2482 Config Presence Pulse Masking 00083 #define DS2482_CFG_SPU 0x04 //< DS2482 Config Strong Pull-Up 00084 #define DS2482_CFG_1WS 0x08 //< DS2482 Config 1-Wire Speed 00085 00086 // DS2482 channel selection code defines 00087 #define DS2482_CH_IO0 0xF0 //< DS2482 Select Channel IO0 00088 #define DS2482_CH_IO1 0xE1 //< DS2482 Select Channel IO1 00089 #define DS2482_CH_IO2 0xD2 //< DS2482 Select Channel IO2 00090 #define DS2482_CH_IO3 0xC3 //< DS2482 Select Channel IO3 00091 #define DS2482_CH_IO4 0xB4 //< DS2482 Select Channel IO4 00092 #define DS2482_CH_IO5 0xA5 //< DS2482 Select Channel IO5 00093 #define DS2482_CH_IO6 0x96 //< DS2482 Select Channel IO6 00094 #define DS2482_CH_IO7 0x87 //< DS2482 Select Channel IO7 00095 00096 // DS2482 read pointer code defines 00097 #define DS2482_READPTR_SR 0xF0 //< DS2482 Status Register 00098 #define DS2482_READPTR_RDR 0xE1 //< DS2482 Read Data Register 00099 #define DS2482_READPTR_CSR 0xD2 //< DS2482 Channel Selection Register 00100 #define DS2482_READPTR_CR 0xC3 //< DS2482 Configuration Register 00101 00102 00103 // API mode bit flags 00104 #define MODE_STANDARD 0x00 00105 #define MODE_OVERDRIVE 0x01 00106 #define MODE_STRONG 0x02 00107 00108 // One Wire 00109 #define OW_MATCH_ROM 0x55 00110 #define OW_SKIP_ROM 0xCC 00111 #define OW_CONVERT_TEMP 0x44 00112 #define OW_WR_SCRATCHPAD 0x4E 00113 #define OW_RD_SCRATCHPAD 0xBE 00114 #define OW_SEARCH_ROM 0xF0 00115 00116 #define DS1820_LSB 0 00117 #define DS1820_MSB 1 00118 00119 // ============================================================================ 00120 00121 class DS2482 00122 { 00123 public: 00124 00125 // ROM code structure 00126 typedef struct sOW_ROM_CODE_ITEM 00127 { 00128 uint8_t adr; // Adresse fuer den Baustein 00129 uint8_t status; // Status fuer den Wandler 00130 // bit 1 bis 4 0 = inaktiv 00131 // 1 = Baustein erkannt 00132 // 2 = wird als trigger verwendet 00133 // 3 = Messung duchgeführt 00134 // 4 = Übertagungsfehler 00135 // bit 8 ist fuerBuskennung 00136 int16_t result; // Ablage fuer Temperaturwert 00137 float value; // Ablage fuer Stromwert 00138 int16_t value_2; // Ablage fuer Spannungwert 00139 uint8_t rom[8]; // 8 Bytes for ROM code 00140 } tOW_ROM_CODE_ITEM; 00141 00142 typedef struct sOW 00143 { 00144 uint8_t devices; // Number of devices 00145 uint8_t device_table_index; 00146 tOW_ROM_CODE_ITEM device_table[OW_MAX_DEVICES]; // OW-Device data table 00147 } tOW; 00148 00149 // global vars 00150 tOW ow; 00151 00152 /** Create an instance of the PCF8574 connected to specfied I2C pins, with the specified address. 00153 * 00154 * @param sda The I2C data pin 00155 * @param scl The I2C clock pin 00156 * @param address The I2C address for this DS2482 00157 */ 00158 00159 DS2482(PinName sda, PinName scl, int address); 00160 00161 //----------------------------------------------------------------------------- 00162 // functions 00163 00164 // crc buffer 00165 uint8_t crc_calc(uint8_t x); 00166 uint8_t crc_calc_buffer(uint8_t* pbuffer,uint8_t count); 00167 00168 uint8_t detect(void); 00169 int reset(void); 00170 uint8_t write_config(uint8_t config); 00171 00172 //uint8_t DS2482_channel_select(uint8_t channel); 00173 uint8_t OWReset(void); 00174 void OWWriteBit(uint8_t sendbit); 00175 uint8_t OWReadBit(void); 00176 uint8_t OWTouchBit(uint8_t sendbit); 00177 void OWWriteByte(uint8_t sendbyte); 00178 uint8_t OWReadByte(void); 00179 void OWBlock(uint8_t *tran_buf, uint8_t tran_len); 00180 uint8_t OWTouchByte(uint8_t sendbyte); 00181 uint8_t OWFirst(void); 00182 uint8_t OWNext(void); 00183 int OWVerify(void); 00184 void OWTargetSetup(uint8_t family_code); 00185 void OWFamilySkipSetup(void); 00186 uint8_t OWSearch(void); 00187 uint8_t search_triplet(uint8_t search_direction); 00188 uint8_t OWSpeed(uint8_t new_speed); 00189 uint8_t OWLevel(uint8_t new_level); 00190 uint8_t OWReadBitPower(uint8_t applyPowerResponse); 00191 uint8_t OWWriteBytePower(uint8_t sendbyte); 00192 00193 void DS18XX_Read_Address(void); 00194 void start_conversion(void); 00195 00196 bool ds1820_start_conversion(uint8_t command); 00197 bool ds18B20_read_hrtemp(void); 00198 00199 protected: 00200 00201 bool ow_read_rom(void); 00202 bool ow_read_scratchpad(void); 00203 bool ow_read_scratchpad_ds2438(uint8_t page); 00204 bool ow_write_scratchpad_ds18x20 (uint8_t th, uint8_t tl); 00205 bool ow_write_scratchpad_ds2438 (uint8_t th, uint8_t tl); 00206 bool ow_write_eeprom_ds18x20 (void); 00207 bool ow_write_eeprom_ds2438 (void); 00208 void ow_read_address (void); 00209 //----------------------------------------------------------------------------- 00210 // vars 00211 00212 I2C i2c; 00213 int addr; 00214 uint8_t crc_value; 00215 00216 // Search state 00217 // Variablen in einem Block ablegen 00218 uint8_t ow_scratchpad[OW_SCRATCHPAD_BYTES]; // Scratchpad memory 00219 uint8_t ow_rom_code[OW_ROM_CODE_BYTES]; // Temporary ROM code 00220 uint8_t ow_flags; 00221 uint8_t ROM_NO[8]; // temporary ROM Code 00222 uint8_t LastDiscrepancy; 00223 uint8_t LastFamilyDiscrepancy; 00224 uint8_t LastDeviceFlag; 00225 00226 // DS2482 state 00227 uint8_t c1WS, cSPU, cPPM, cAPU; 00228 uint8_t short_detected; 00229 00230 bool ds1820_request_pending; 00231 00232 }; // end class 00233 00234 #endif
Generated on Wed Jul 13 2022 07:09:37 by
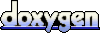