
Two player imu pong
Dependencies: 4DGL-uLCD-SE IMUfilter LSM9DS0 PinDetect mbed
ball.cpp
00001 00002 #include "ball.h" 00003 //#include "tempModule.h" 00004 00005 00006 00007 Ball::Ball(PinName pin) 00008 { 00009 //tempSensor = new TempModule(pin); 00010 setBaseVx(2.0); 00011 setBaseVy(2.0); 00012 setVySign(1); 00013 setVxSign(1); 00014 radius = 5; 00015 setX(50); 00016 setY(21); 00017 } 00018 00019 Ball::Ball(PinName pin, float Vx, float Vy, int rad,int xp, int yp) 00020 { 00021 //tempSensor = new TempModule(pin , Vx, Vy); 00022 setBaseVx(Vx); 00023 setBaseVy(Vy); 00024 setVxSign(1); 00025 setVySign(1); 00026 radius = rad; 00027 setX(xp); 00028 setY(yp); 00029 00030 00031 } 00032 00033 // set functions 00034 void Ball::setX(int xp) 00035 { 00036 x = xp; 00037 } 00038 void Ball::setY(int yp) 00039 { 00040 y = yp; 00041 00042 } 00043 void Ball::setRand(int seed) 00044 { 00045 srand(seed); 00046 int random = (rand() % (118 - 2*radius)) + radius; 00047 setX(random); 00048 int random2 = (rand() % (127 - 2*radius)) + radius; 00049 setY(random2); 00050 00051 } 00052 void Ball::setBaseVx(float Vx) 00053 { 00054 basevx = Vx; 00055 //tempSensor->setBaseVx(vxSign*Vx); 00056 00057 } 00058 00059 00060 void Ball::setBaseVy(float Vy) 00061 { 00062 basevy = Vy; 00063 //tempSensor->setBaseVy(Vy); 00064 00065 } 00066 00067 void Ball::setVxSign(int s) 00068 { 00069 vxSign = s; 00070 } 00071 00072 void Ball::setVySign(int s) 00073 { 00074 vySign = s; 00075 00076 } 00077 00078 00079 //get functions 00080 00081 int Ball::getVx() 00082 { 00083 return basevx; 00084 } 00085 00086 int Ball::getVy() 00087 { 00088 return basevy; 00089 } 00090 00091 int Ball::getFutureX() 00092 { 00093 return vxSign * getVx() + x ; 00094 //return vxSign * tempSensor->getVx() + x ; 00095 00096 } 00097 00098 int Ball::getFutureY() 00099 { 00100 return vySign * getVy() + y; 00101 //return vySign * tempSensor->getVy() + y; 00102 00103 } 00104 00105 int Ball::getRadius() 00106 { 00107 return radius; 00108 } 00109 //Member Functions 00110 00111 void Ball::reverseXDirection() 00112 { 00113 00114 vxSign = - vxSign; 00115 00116 } 00117 void Ball::reverseYDirection() 00118 { 00119 vySign = -vySign; 00120 00121 } 00122 /* 00123 void Ball::reset( int x, int y, int vx, int vy, uLCD_4DGL*) 00124 { 00125 00126 setBaseVx(vx); 00127 setBaseVy(vy); 00128 uLCD.circle(x,y,radius,WHITE); 00129 00130 00131 } 00132 */ 00133 void Ball::update(uLCD_4DGL *uLCD) 00134 { 00135 uLCD->circle(x,y,radius,BLACK); 00136 x = (int) getFutureX(); 00137 y = (int) getFutureY(); 00138 uLCD->circle(x,y,radius,WHITE); 00139 00140 00141 } 00142 /* 00143 void Ball::dispTemp(uLCD_4DGL *uLCD) 00144 { 00145 00146 float garbage = tempSensor->getVx(uLCD); 00147 00148 } 00149 */ 00150 bool Ball::CheckHit() 00151 { bool hit = false; 00152 /* 00153 if ((getFutureX()<=radius+1)) 00154 { 00155 reverseXDirection(); 00156 hit = true; 00157 } 00158 */ 00159 if ((getFutureY()<=radius+1) || (getFutureY()>=126-radius)) 00160 { 00161 reverseYDirection(); 00162 hit = true; 00163 } 00164 return hit; 00165 00166 } 00167 bool Ball::CheckLose() 00168 { 00169 if ((getFutureX()>=126-radius)) 00170 { 00171 //vx = 0; 00172 //vy = 0; 00173 return true; 00174 } 00175 00176 if ((getFutureX() <= radius)) 00177 { 00178 return true; 00179 } 00180 else 00181 {return false;} 00182 } 00183
Generated on Mon Jul 18 2022 23:37:14 by
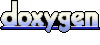