
The NXP LCP1768 and mbed Application Board on 2lemetry platform.
Dependencies: C12832_lcd EthernetInterface MMA7660 MQTT mbed-rtos mbed
Fork of MQTTTest by
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetInterface.h" 00003 #include "C12832_lcd.h" 00004 #include "PubSubClient.h" 00005 #include "MMA7660.h" 00006 00007 #define _2LEMETRY_USERID "<2lemetry user id>" 00008 #define _2LEMETRY_TOKEN "<2lemetry token>" 00009 #define _2LEMETRY_DOMAIN "<2lemetry domain - usually same as user id>" 00010 #define _2LEMETRY_STUFF "<2lemetry stuff - a grouping of devices - recommend 'mbed' to get started>" 00011 #define _2LEMETRY_DEVICE_ID "<2lemetry device id - unique device id - mac / serial # - recommend 'nxp01' to get started>" 00012 00013 C12832_LCD lcd; 00014 Serial pc(USBTX, USBRX); 00015 00016 char* serverIpAddr = "q.mq.tt"; 00017 int port = 1883; /*Sever Port*/ 00018 void callback(char* topic, char* payload, unsigned int len); /*Callback function prototype*/ 00019 PubSubClient mqtt(serverIpAddr, port, callback); 00020 EthernetInterface eth; 00021 00022 MMA7660 MMA(p28, p27); 00023 00024 void callback(char* topic, char* payload, unsigned int len) 00025 { 00026 // for now just print the payload 00027 lcd.printf("Payload: %s\r\n\r\n", payload); 00028 wait(2); 00029 lcd.cls(); 00030 } 00031 00032 int main() { 00033 char clientID[30]; 00034 char topicString[50]; 00035 00036 eth.init(); //Use DHCP 00037 eth.connect(); 00038 lcd.cls(); 00039 lcd.locate(0,3); 00040 pc.printf("IP Address is %s\n", eth.getIPAddress()); 00041 00042 pc.printf("mbed example on 2lemetry"); 00043 00044 // create MQTT client ID 00045 sprintf(clientID, _2LEMETRY_DEVICE_ID); 00046 00047 if(!mqtt.connect(clientID, _2LEMETRY_USERID, _2LEMETRY_TOKEN)){ 00048 pc.printf("\r\nConnect to server failed ..\r\n"); 00049 return -1; 00050 } 00051 00052 pc.printf("\r\nConnect to server successful ..\r\n"); 00053 00054 int i = 0; 00055 char pubString[50]; 00056 00057 // temporarily use pubTopic string to create subscription topic 00058 sprintf(topicString, "%s/%s/%s/cmd", _2LEMETRY_DOMAIN, _2LEMETRY_STUFF, _2LEMETRY_DEVICE_ID); 00059 00060 mqtt.subscribe(topicString); 00061 00062 // now overwrite with publish string 00063 sprintf(topicString, "%s/%s/%s", _2LEMETRY_DOMAIN, _2LEMETRY_STUFF, _2LEMETRY_DEVICE_ID); 00064 00065 while(1) { 00066 int x_val, y_val, z_val; 00067 x_val = (int)(MMA.x() * 1000); 00068 y_val = (int)(MMA.y() * 1000); 00069 z_val = (int)(MMA.z() * 1000); 00070 sprintf(pubString, "{\"x\":%d,\"y\":%d,\"z\":%d}", x_val, y_val, z_val); 00071 mqtt.publish(topicString, pubString); 00072 wait(1); 00073 mqtt.loop(); 00074 } 00075 }
Generated on Thu Jul 14 2022 20:18:31 by
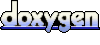